Angular Debugging Techniques: Tips and Tricks
1. Angular Debugging Techniques: Tips and Tricks
Debugging in Angular can be both a challenging and rewarding endeavor. As developers, we often find ourselves facing perplexing issues that hinder our progress. However, with the right techniques and tools at our disposal, we can efficiently troubleshoot and resolve these issues, ultimately improving the quality of our applications. In this article, we’ll explore some advanced debugging techniques for Angular, along with practical examples to illustrate each approach.
1.1. Logging and Console Debugging
One of the simplest yet most effective debugging techniques is logging and using the browser’s console to inspect variables, functions, and errors. Angular provides several built-in methods for logging messages, such as `console.log()`, `console.warn()`, and `console.error()`. These statements can help you track the flow of your application and identify potential issues.
Example:
```typescript // Component file import { Component } from '@angular/core'; @Component({ selector: 'app-example', templateUrl: './example.component.html', styleUrls: ['./example.component.css'] }) export class ExampleComponent { constructor() { console.log('Component initialized'); } } ```
Further Reading:
– MDN Web Docs – Console – https://developer.mozilla.org/en-US/docs/Web/API/Console
1.2. Augury Chrome Extension
Augury is a powerful Chrome extension specifically designed for debugging Angular applications. It provides insights into the structure of your components, the state of your application, and performance metrics. With Augury, you can visually inspect your application’s components, view data bindings, and analyze the dependency injection hierarchy.
Example:
Install the Augury extension from the Chrome Web Store and open the DevTools while running your Angular application to access its features.
Further Reading:
– Augury Documentation – https://augury.rangle.io/docs/
– Chrome Web Store – Augury Extension – https://chrome.google.com/webstore/detail/augury/elgalmkoelokbchhkhacckoklkejnhcd
3. Remote Debugging with Angular CLI
Angular CLI supports remote debugging, allowing you to debug your application on a different device or browser instance. This can be particularly useful for troubleshooting issues that only occur in specific environments or devices. By enabling remote debugging, you can connect your Angular application to the Chrome DevTools on another device and inspect its behavior in real-time.
Example:
```bash ng serve --host 0.0.0.0 --port 4200 --disable-host-check ```
Access your application from another device on the same network and open Chrome DevTools to debug remotely.
Further Reading:
– Angular CLI Documentation – Serving Applications – https://angular.io/cli/serve
Conclusion
Effective debugging is an essential skill for every Angular developer. By mastering these debugging techniques and tools, you can streamline your development process, identify issues quickly, and deliver high-quality applications. Whether you prefer traditional logging or advanced debugging tools like Augury, experimentation and practice are key to becoming proficient in Angular debugging.
Remember, debugging is not just about fixing errors; it’s also an opportunity to deepen your understanding of Angular and improve your overall development skills. So, embrace the challenge, experiment with different techniques, and never stop learning.
Happy debugging!
Additional Resources:
– Angular Documentation – https://angular.io/docs
– Stack Overflow – https://stackoverflow.com/questions/tagged/angular
– Angular Reddit Community – https://www.reddit.com/r/Angular2/
Table of Contents
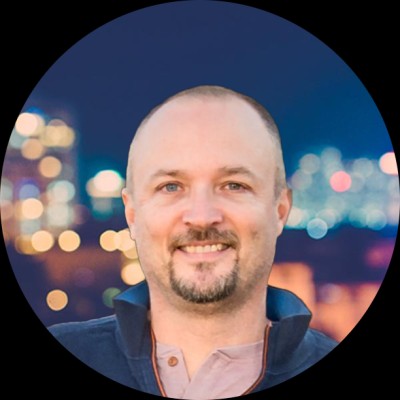
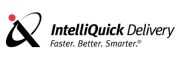