Working with Angular Directives: A Practical Guide
Angular, developed by Google, is a powerful and widely adopted JavaScript framework used for building dynamic web applications. One of the key features that make Angular so versatile and flexible is its use of directives. In this guide, we’ll explore what directives are, how they work, and how to use them effectively to enhance your Angular applications.
Table of Contents
1. What are Angular Directives?
At its core, a directive is a powerful tool in Angular that allows you to extend the functionality of HTML elements. Directives are used to manipulate the DOM, create reusable components, and provide additional behavior to HTML elements. Angular comes with several built-in directives, such as ngIf, ngFor, and ngStyle, but you can also create custom directives to suit your specific needs.
2. The Anatomy of an Angular Directive
Before we dive into the practical aspects of working with directives, let’s understand the structure of an Angular directive:
- Directive Decorator: To create a directive, you must use the @Directive decorator. This decorator is used to mark a TypeScript class as an Angular directive.
- Selector: The selector is a string used to identify the HTML element to which the directive will be applied. It can be an element selector, attribute selector, class selector, or a combination of these.
- HostListener and HostBinding (Optional): These decorators are used to listen to events on the host element and bind values to the host element’s properties, respectively.
- Directive Logic: The directive class contains the logic that defines what the directive does when applied to an element.
3. Built-in Directives in Angular
Angular provides several built-in directives that can be readily used in your applications:
3.1. ngIf
The ngIf directive allows you to conditionally render an element based on an expression. For example:
html <element *ngIf="condition">Content to display when condition is true.</element>
3.2. ngFor
The ngFor directive is used to loop over a collection and render elements dynamically. For example:
html <element *ngFor="let item of items">{{ item }}</element>
3.3. ngStyle
The ngStyle directive allows you to apply inline styles to an element based on an expression. For example:
html <element [ngStyle]="{ 'color': textColor, 'font-size': fontSize }">Styled Content</element>
4. Creating Custom Directives
While the built-in directives are useful, you will often encounter scenarios where you need custom functionality for your application. Angular makes it easy to create custom directives tailored to your needs. Let’s go through the steps to create a simple custom directive that highlights an element.
Step 1: Generate the Directive
To generate a new directive in your Angular project, you can use the Angular CLI:
bash ng generate directive highlight
This will create a new directive file named highlight.directive.ts.
Step 2: Implement the Directive Logic
Open the highlight.directive.ts file and implement the directive logic:
typescript import { Directive, ElementRef, HostListener, Input } from '@angular/core'; @Directive({ selector: '[appHighlight]' }) export class HighlightDirective { @Input('appHighlight') highlightColor: string; constructor(private el: ElementRef) { } @HostListener('mouseenter') onMouseEnter() { this.highlight(this.highlightColor || 'yellow'); } @HostListener('mouseleave') onMouseLeave() { this.highlight(null); } private highlight(color: string | null) { this.el.nativeElement.style.backgroundColor = color; } }
In this example, we create a directive called HighlightDirective that takes an input highlightColor to specify the background color. When the mouse enters the element, the background color is set to the specified color. When the mouse leaves the element, the background color is reset.
Step 3: Using the Custom Directive
Now that we have created the custom directive, let’s see how we can use it in our components:
html <div appHighlight highlightColor="lightblue"> Hover over me to see the highlight effect! </div>
In this example, we apply our appHighlight directive to a <div> element and provide the highlightColor input with the value “lightblue”. As a result, when we hover over the element, it will be highlighted with a light blue background.
5. Directive Lifecycle Hooks
As with components, directives have lifecycle hooks that allow you to tap into various stages of the directive’s life. Some common lifecycle hooks for directives include:
5.1. ngOnChanges
This hook is called when the input properties of the directive are updated. It receives a SimpleChanges object containing the previous and current values of the input properties.
5.2. ngOnInit
This hook is called once, right after the directive’s data-bound properties have been initialized. It is a good place to perform any setup logic.
5.3. ngDoCheck
This hook is called during every change detection cycle, allowing you to implement custom change detection logic.
5.4. ngOnDestroy
This hook is called just before the directive is destroyed, providing an opportunity to clean up resources.
6. Best Practices for Working with Directives
When working with Angular directives, it’s essential to follow some best practices to ensure maintainable and efficient code:
6.1. Keep Directives Simple and Focused
Each directive should have a specific, well-defined purpose. Avoid creating directives that try to handle multiple unrelated tasks.
6.2. Use Input and Output Properties
Use input properties to pass data into the directive and output properties to emit events out of the directive.
6.3. Leverage HostBindings and HostListeners
Use HostBinding to set properties on the host element and HostListener to listen to events on the host element. This keeps the directive logic encapsulated.
6.4. Avoid Direct DOM Manipulation
Manipulating the DOM directly within a directive can lead to bugs and performance issues. Instead, use Angular’s data binding and rendering mechanisms.
Conclusion
Angular directives are a powerful way to enhance the functionality and behavior of your web applications. In this practical guide, we explored the basics of directives, how to create custom directives, and best practices for working with directives. By harnessing the power of directives effectively, you can build more maintainable, scalable, and dynamic Angular applications.
So, go ahead and experiment with directives in your Angular projects, and unlock the full potential of this remarkable framework!
Table of Contents
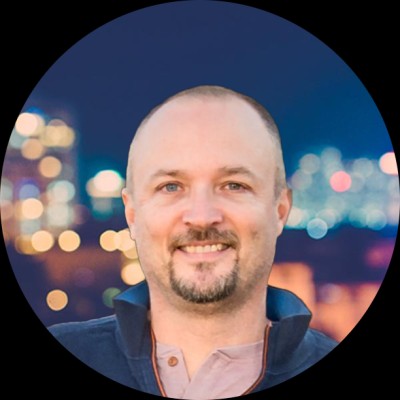
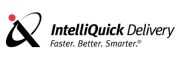