Angular and Firebase Authentication: Secure User Management
In the dynamic landscape of web development, ensuring robust user authentication and management is paramount. Angular, a popular frontend framework, combined with Firebase, a comprehensive backend platform, offers a seamless solution for building secure user authentication systems. In this guide, we’ll delve into the integration of Angular with Firebase Authentication to empower developers in crafting robust and secure user management systems.
1. Why Angular and Firebase?
Angular, maintained by Google, provides a structured framework for building single-page web applications. Its powerful features and extensive ecosystem make it a preferred choice for developers seeking scalability and maintainability.
Firebase, also developed by Google, offers a suite of tools for building and managing web and mobile applications. Firebase Authentication, a key component of the Firebase platform, provides simple yet secure authentication methods, including email/password, social authentication, and more.
2. Integration Steps
- Setting up Firebase Project: Begin by creating a Firebase project through the Firebase Console. Once the project is created, obtain the Firebase configuration details, including API keys and project IDs.
- Installing AngularFire: AngularFire is the official Angular library for Firebase. Install it in your Angular project using npm:
``` npm install @angular/fire firebase ```
- Configuration: Configure AngularFire with Firebase credentials by importing `AngularFireModule` and `AngularFireAuthModule` in your Angular app’s main module.
```typescript import { AngularFireModule } from '@angular/fire'; import { AngularFireAuthModule } from '@angular/fire/auth'; import { environment } from '../environments/environment'; @NgModule({ imports: [ AngularFireModule.initializeApp(environment.firebaseConfig), AngularFireAuthModule ], ... }) export class AppModule { } ```
- Implementing Authentication: Utilize AngularFireAuth service to implement various authentication methods in your Angular application. For example, to enable email/password authentication:
```typescript import { AngularFireAuth } from '@angular/fire/auth'; constructor(private afAuth: AngularFireAuth) {} signIn(email: string, password: string) { return this.afAuth.signInWithEmailAndPassword(email, password); } ```
- Securing Routes: Implement route guards to restrict access to certain routes based on user authentication status. AngularFire provides `AngularFireAuthGuard` for this purpose.
```typescript import { AngularFireAuthGuard } from '@angular/fire/auth-guard'; import { map } from 'rxjs/operators'; canActivate(route: ActivatedRouteSnapshot, state: RouterStateSnapshot) { return this.afAuth.authState.pipe( map(user => !!user) ); } ```
3. Examples of Angular-Firebase Authentication in Action
- User Authentication: Implementing user registration and login functionality using Firebase Authentication. Link to tutorial – https://angularfirebase.com/lessons/google-user-auth-with-firestore-custom-data/
- Social Authentication: Integrating social authentication providers like Google, Facebook, and Twitter into your Angular application using Firebase Authentication. Link to guide – https://fireship.io/lessons/angularfire-google-oauth/
- Real-time Database Integration: Leveraging Firebase Realtime Database with AngularFire to build a secure and scalable user management system. Link to tutorial – https://angularfirebase.com/lessons/firebase-realtime-database-crud-operations/
Conclusion
Angular and Firebase offer a potent combination for building secure and scalable user authentication systems. By following the integration steps and leveraging the powerful features of AngularFire, developers can streamline the process of implementing robust user management functionalities in their Angular applications.
With the flexibility and reliability provided by Angular and Firebase, developers can focus more on crafting delightful user experiences while ensuring the highest standards of security and performance.
Start harnessing the power of Angular and Firebase today to revolutionize your user authentication workflows and take your applications to the next level of excellence.
Table of Contents
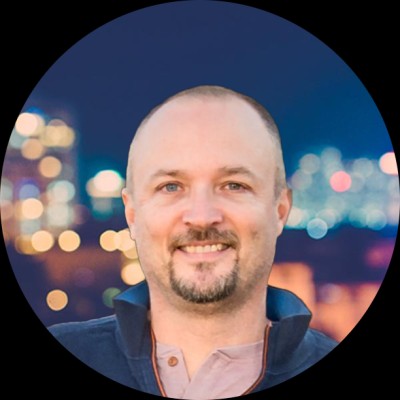
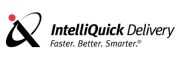