Unlocking the Power of Angular: A Comprehensive Guide to Directives
Angular is a powerful, widely-used platform for building robust, dynamic web applications, which makes it a popular choice for businesses looking to hire Angular developers. One of its core features is its directives, an integral tool that allows developers to extend the functionality of HTML. This unique feature transforms Angular into an ideal solution for creating responsive, highly interactive web apps. In this post, we’ll take a deep dive into Angular directives, shedding light on why they are so coveted and why you might want to hire Angular developers to leverage their full potential. This knowledge will not only help you sharpen your own web development skills but also give you insights into the capabilities of the Angular developers you plan to hire.
What are Angular Directives?
Angular directives are classes with a decorator. They allow developers to write new HTML syntax that is specific to their application or to manipulate the DOM structure (elements, attributes, and so on).
There are three types of directives in Angular:
- Component Directives: These are directives with a template. They essentially define a ‘component’, which is a combination of a view (HTML) and a controller (Class).
- Attribute Directives: These directives change the behavior, look, and feel of a DOM element. For example, changing the color or behavior of a button.
- Structural Directives: These directives change the layout by adding and removing DOM elements.
Now, let’s take a closer look at each of these directive types with some practical examples.
Component Directives
Component directives are the most common type of directive and the one you’ll likely interact with the most. A component directive defines a view and its associated logic.
Here is a basic example:
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: `<h1>Welcome to {{ title }}!</h1>`, }) export class AppComponent { title = 'My Angular App'; } ```
In this example, ‘app-root’ is the HTML tag that the Angular application looks for to insert the AppComponent view. The HTML within the template displays a welcome message.
Attribute Directives
Attribute directives are used to change the behavior or appearance of a DOM element, component, or another directive.
For instance, let’s create a directive that changes the background color of an element when hovered over:
```typescript import { Directive, ElementRef, HostListener } from '@angular/core'; @Directive({ selector: '[appHoverHighlight]' }) export class HoverHighlightDirective { constructor(private el: ElementRef) {} @HostListener('mouseenter') onMouseEnter() { this.highlight('yellow'); } @HostListener('mouseleave') onMouseLeave() { this.highlight(null); } private highlight(color: string) { this.el.nativeElement.style.backgroundColor = color; } } ```
In your HTML, you would use it like this:
```html <p appHoverHighlight>Hover over me!</p> ```
When you hover over the paragraph, it will turn yellow, and when you move the mouse away, it will return to its original color.
Structural Directives
Structural directives are responsible for HTML layout. They shape or reshape the DOM’s structure, by adding, removing, and manipulating elements.
For example, Angular comes with a built-in directive called ‘*ngFor’ that lets you loop over an array and create a DOM element for each item.
Here is a simple example:
```typescript import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div *ngFor="let item of items"> {{ item }} </div> `, }) export class AppComponent { items = ['Item 1', 'Item 2', 'Item 3']; } ```
In this case, the ‘*ngFor’ directive creates a new ‘div’ for each ‘item’ in the ‘items’ array.
Creating Custom Directives
To truly unleash the power of Angular directives,
you’ll want to create your own. Here’s an example of a simple custom directive:
```typescript import { Directive, ElementRef, Renderer2 } from '@angular/core'; @Directive({ selector: '[appCustomHighlight]' }) export class CustomHighlightDirective { constructor(private el: ElementRef, private renderer: Renderer2) { this.renderer.setStyle(this.el.nativeElement, 'backgroundColor', 'lightblue'); } } ```
And to use this directive in your HTML:
```html <p appCustomHighlight>My custom highlight!</p> ```
This will set the paragraph’s background color to light blue.
Conclusion
Angular directives are a powerful tool not only in any developer’s toolbox but also a primary reason to hire Angular developers. These professionals can effectively utilize directives for complex manipulations of the DOM, creating highly dynamic, interactive user interfaces. By understanding and leveraging component, attribute, and structural directives, Angular developers can create their own custom directives, resulting in more efficient, readable, and maintainable code. So, whether you’re looking to enhance your own Angular development skills or planning to hire Angular developers for your next project, understanding the importance and usage of directives is crucial. Take the plunge, start experimenting with directives today, and witness the transformation in your web development journey!
Table of Contents
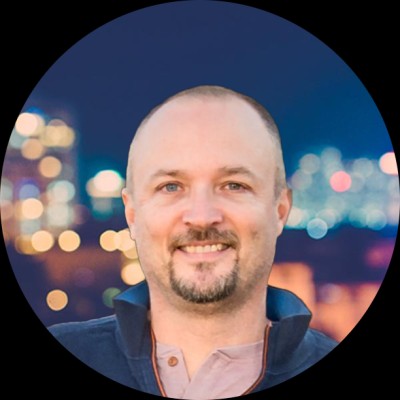
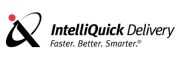