Angular Internationalization: Creating Multilingual Apps
In today’s globalized world, creating applications that cater to users from different linguistic backgrounds is crucial. Angular, a popular framework for building web applications, offers robust tools for internationalization (i18n), enabling developers to create multilingual apps efficiently. This article explores how to leverage Angular’s i18n features to create applications that support multiple languages and locales.
Understanding Internationalization (i18n) in Angular
Internationalization (i18n) is the process of designing your application so that it can be adapted to various languages and regions without requiring engineering changes. Angular provides built-in tools to handle translation, formatting, and localization, making it easier to create apps that are accessible to a global audience.
Setting Up Angular for Internationalization
Before diving into the implementation, you need to set up your Angular project to support i18n. This involves enabling Angular’s i18n tools and configuring your app for different languages.
1. Installing the Necessary Packages
Start by ensuring that your Angular project is ready for internationalization by installing the necessary packages:
```bash ng add @angular/localize ```
This command sets up the Angular framework to support localization and internationalization features.
2. Marking Text for Translation
The next step is to mark the text in your application that needs to be translated. Angular provides the `i18n` attribute to help you identify and manage translatable content.
Example: Marking Text for Translation
```html <p i18n="@@welcomeMessage">Welcome to our application!</p> ```
In this example, the `i18n` attribute marks the text “Welcome to our application!” for translation, and the `@@welcomeMessage` is a unique identifier for this translation unit.
Translating Your Application
Once you’ve marked the translatable text, the next step is to create translation files for each language you want to support. Angular uses XLIFF (XML Localization Interchange File Format) files for this purpose.
1. Extracting Translation Strings
You can extract the marked translatable strings into a translation file using Angular CLI:
```bash ng extract-i18n --output-path src/locale ```
This command generates a default translation file in the `src/locale` directory, which you can then translate into different languages.
2. Translating XLIFF Files
Translate the generated XLIFF file into the desired languages. Here’s an example translation for French (fr):
```xml <trans-unit id="welcomeMessage" datatype="html"> <source>Welcome to our application!</source> <target>Bienvenue dans notre application!</target> </trans-unit> ```
3. Building and Serving the Application for Different Locales
Once your translations are in place, you can build and serve the application for different locales using the following command:
```bash ng build --localize ```
Angular will generate a separate version of your app for each locale you have translations for, allowing users to experience your app in their preferred language.
Handling Dynamic Content with Angular Pipes
In addition to static text, you may need to internationalize dynamic content like dates, numbers, and currencies. Angular provides built-in pipes to handle these localizations based on the user’s locale.
Example: Formatting Dates and Numbers
```html <p>{{ today | date:'longDate' }}</p> <p>{{ price | currency:'EUR' }}</p> ```
In this example, the `date` and `currency` pipes automatically format the content according to the user’s locale.
Switching Languages Dynamically
To make your app more user-friendly, you might want to allow users to switch languages dynamically without reloading the page. Angular enables this through the use of a service that manages the active locale.
Example: Language Switcher Component
Here’s an example of how to implement a language switcher in your Angular app:
```typescript import { Component } from '@angular/core'; import { TranslateService } from '@ngx-translate/core'; @Component({ selector: 'app-language-switcher', template: ` <select (change)="switchLanguage($event.target.value)"> <option *ngFor="let lang of languages" [value]="lang">{{ lang }}</option> </select> ` }) export class LanguageSwitcherComponent { languages = ['en', 'fr', 'es']; constructor(private translateService: TranslateService) {} switchLanguage(language: string) { this.translateService.use(language); } } ```
This component allows users to select their preferred language from a dropdown, and the app will update dynamically.
Conclusion
Internationalizing your Angular application is essential for reaching a global audience. By utilizing Angular’s built-in i18n tools, you can efficiently create multilingual apps that cater to users from different linguistic and cultural backgrounds. From marking translatable content to managing dynamic content and enabling language switching, Angular provides all the necessary features to make your app accessible worldwide.
Further Reading:
Table of Contents
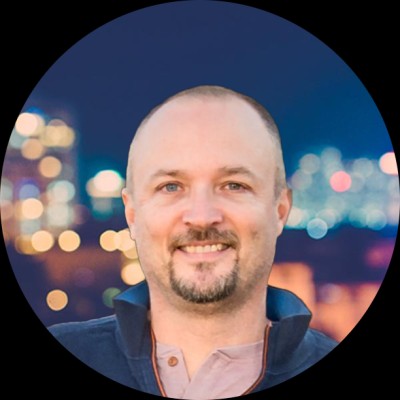
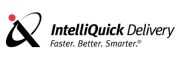