Navigating the Art of Hiring Angular Developers: A Comprehensive Interview Questions Guide
Angular, the dynamic front-end framework, has redefined the landscape of web development. To create outstanding web applications, hiring skilled Angular developers is essential. This guide is your compass through the hiring process, empowering you to evaluate candidates’ technical proficiency, problem-solving abilities, and Angular expertise. Let’s embark on this journey, uncovering the essential interview questions and strategies to pinpoint the perfect Angular developer for your team.
Table of Contents
1. How to Hire Angular Developers
Embarking on the journey to hire Angular developers? Follow these steps for success:
- Job Requirements: Define specific job requirements, outlining the skills and experience you desire.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ Angular expertise, relevant experience, and additional skills.
- Technical Assessment: Devise a robust technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of Angular Developers to Look For
When assessing Angular developers, keep an eye out for these pivotal skills:
- Angular Proficiency: A solid grasp of Angular syntax, components, directives, and services.
- Front-End Expertise: Familiarity with HTML, CSS, and JavaScript, and their integration with Angular.
- Single Page Application (SPA) Development: Experience building dynamic and interactive SPAs using Angular.
- Data Binding and Component Interaction: Understanding of data binding mechanisms and communication between components.
- Routing and Navigation: Proficiency in implementing routing and navigation for seamless user experiences.
- Unit Testing: Ability to write effective unit tests using testing frameworks like Jasmine and Karma.
- Problem-Solving Skills: Adeptness in dissecting complex problems and devising efficient solutions.
3. Overview of the Angular Developer Hiring Process
Here’s a bird’s-eye view of the Angular developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create enticing job descriptions that accurately reflect the role, attracting the right candidates.
3.3 Crafting Angular Developer Interview Questions
Develop a comprehensive set of interview questions covering Angular intricacies, problem-solving aptitude, and relevant technologies.
4. Sample Angular Developer Interview Questions and Answers
Dive into these sample questions with in-depth answers to assess candidates’ Angular skills:
Q1. Explain the concept of two-way data binding in Angular and provide an example.
A: Two-way data binding allows automatic synchronization of data between the view and the component. An example:
<input [(ngModel)]="name"> <p>Hello, {{ name }}!</p>
Q2. What are Angular services, and how are they used?
A: Angular services are singleton objects that provide functionality and data to components. They promote code reusability and separate concerns.
Q3. Write an Angular component that fetches data from an API and displays it in a template.
import { Component, OnInit } from '@angular/core'; import { ApiService } from './api.service'; @Component({ selector: 'app-data', template: '<div>{{ data }}</div>' }) export class DataComponent implements OnInit { data: any; constructor(private apiService: ApiService) {} ngOnInit() { this.apiService.getData().subscribe(result => { this.data = result; }); } }
Q4. Explain the concept of dependency injection in Angular and why it’s important.
A: Dependency injection is a design pattern that allows you to provide dependent objects to a class rather than creating them internally. It promotes loose coupling and modular design.
Q5. How does Angular routing work, and how do you configure routes in an Angular application?
A: Angular routing allows navigation between different views in a single page application. Routes are configured using the RouterModule and defined in the app-routing.module.ts file.
Q6. Describe the purpose of Angular directives and provide examples of built-in directives.
A: Directives are markers on a DOM element that tell Angular to perform specific behavior. Examples of built-in directives are ngIf, ngFor, and ngStyle.
Q7. Explain the concept of Angular template-driven forms and provide an example.
A: Template-driven forms in Angular allow you to create forms using Angular directives in the template. An example:
<form (ngSubmit)="onSubmit()"> <input [(ngModel)]="name" name="name" required> <button type="submit">Submit</button> </form>
Q8. How do you handle HTTP requests in Angular applications using HttpClient?
A: HttpClient is a module that facilitates making HTTP requests. An example of making a GET request:
import { HttpClient } from '@angular/common/http'; constructor(private http: HttpClient) {} fetchData() { this.http.get('/api/data').subscribe(data => { // Handle the data }); }
Q9. Explain the concept of Angular directives and provide examples of built-in directives.
A: Directives are markers on a DOM element that instruct Angular to apply specific behavior to that element or transform the DOM structure. Here are examples of built-in directives:
- ngIf: Conditionally adds or removes elements from the DOM based on an expression’s truthiness.
<div *ngIf="showContent">Content will be displayed if showContent is true.</div>
- ngFor: Iterates over a collection and generates HTML for each item.
<ul> <li *ngFor="let item of items">{{ item }}</li> </ul>
- ngStyle: Applies inline styles based on an expression’s value.
<p [ngStyle]="{ color: isActive ? 'green' : 'red' }">Dynamic color based on isActive.</p>
Q10. Explain the concept of Angular reactive forms and provide an example.
A: Reactive forms in Angular allow you to build forms programmatically using TypeScript. Here’s an example of creating a reactive form for user registration:
import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-registration', template: ` <form [formGroup]="registrationForm" (ngSubmit)="onSubmit()"> <label>Name: <input formControlName="name"></label> <label>Email: <input formControlName="email"></label> <button type="submit">Register</button> </form> `, }) export class RegistrationComponent { registrationForm: FormGroup; constructor(private fb: FormBuilder) { this.registrationForm = this.fb.group({ name: ['', Validators.required], email: ['', [Validators.required, Validators.email]], }); } onSubmit() { if (this.registrationForm.valid) { const formData = this.registrationForm.value; // Send formData to server } } }
By incorporating these additional technical questions and answers into your interview process, you’ll gain a more comprehensive understanding of candidates’ proficiency in Angular development. These questions cover essential aspects of directives and reactive forms, ensuring that you evaluate candidates’ capabilities across a range of crucial Angular concepts.
5. How to Hire Angular Developers through CloudDevs
CloudDevs simplifies the process of hiring top-tier Angular developers:
Step 1: Connect with CloudDevs: Initiate a dialogue with a CloudDevs consultant to discuss your project’s specifics, preferred skills, and anticipated experience.
Step 2: Find the Ideal Match: Within 24 hours, CloudDevs presents you with a curated roster of pre-screened Angular developers. Examine their profiles and choose the developer aligned with your project’s vision.
Step 3: Embark on a No-Risk Trial: Engage in discussions with your chosen developer to ensure seamless onboarding. Once satisfied, formalize the collaboration and begin a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and engage accomplished Angular developers, ensuring your team is equipped with the skills required to create exceptional web applications.
Conclusion
By immersing yourself in the insights shared within this comprehensive guide and employing the detailed interview questions, you’re primed to expertly evaluate the capabilities of Angular developers. Whether you’re crafting dynamic web applications or pioneering interactive front-end solutions, securing the right Angular developers for your team is the cornerstone of your project’s success.
Table of Contents
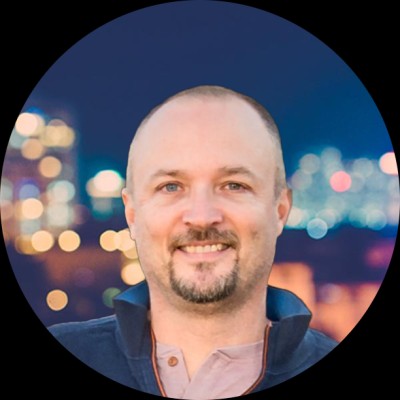
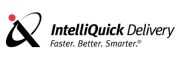