Angular and Performance Monitoring: Metrics and Analytics
Performance monitoring is vital for ensuring that Angular applications run smoothly and efficiently. By keeping track of key metrics and analyzing performance data, developers can identify bottlenecks and optimize their applications. This blog explores how Angular can be used for performance monitoring and provides practical examples of tracking and analyzing metrics.
Understanding Performance Monitoring
Performance monitoring involves collecting and analyzing data on how an application performs, including load times, memory usage, and user interactions. Effective monitoring helps developers ensure that their applications are responsive, efficient, and scalable.
Using Angular for Performance Monitoring
Angular offers various tools and techniques for monitoring performance, including built-in features like Angular CLI commands and third-party libraries. Below are some key methods and code examples demonstrating how to monitor and analyze performance in Angular applications.
1. Measuring Load Times with Angular CLI
The first step in performance monitoring is measuring the load times of your Angular application. The Angular CLI provides built-in commands to analyze build performance and optimize load times.
Example: Analyzing Build Performance with Angular CLI
Use the `ng build –stats-json` command to generate a `stats.json` file that provides detailed information about the build process. This file can be analyzed using tools like Webpack Bundle Analyzer.
```bash ng build --prod --stats-json ```
After generating the `stats.json` file, you can use Webpack Bundle Analyzer to visualize the size of your bundles and identify areas for optimization.
2. Monitoring Application Performance with Angular Performance APIs
Angular provides performance APIs that allow you to measure various aspects of your application’s performance, such as navigation timing, paint timing, and more.
Example: Using PerformanceObserver to Monitor Performance Metrics
Here’s how you can use the `PerformanceObserver` API to monitor key performance metrics in an Angular application.
```typescript import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-performance-monitor', templateUrl: './performance-monitor.component.html' }) export class PerformanceMonitorComponent implements OnInit { ngOnInit(): void { const observer = new PerformanceObserver((list) => { const entries = list.getEntries(); entries.forEach((entry) => { console.log(`${entry.name}: ${entry.startTime}`); }); }); observer.observe({ entryTypes: ['paint', 'navigation'] }); } } ```
This code snippet monitors paint and navigation timings, which are crucial for understanding how quickly your application renders and loads.
3. Analyzing User Interaction with Custom Metrics
Custom metrics can be tracked to analyze how users interact with your application. This can include tracking specific events, such as button clicks or form submissions, to understand user behavior.
Example: Tracking Custom Metrics with Angular Services
You can create a custom service to track user interactions and log them for further analysis.
```typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class AnalyticsService { trackEvent(eventName: string, data: any): void { console.log(`Event: ${eventName}`, data); // Send this data to an analytics server or service } } // Usage in a component import { Component } from '@angular/core'; import { AnalyticsService } from './analytics.service'; @Component({ selector: 'app-button', template: `<button (click)="onClick()">Click me</button>` }) export class ButtonComponent { constructor(private analyticsService: AnalyticsService) {} onClick(): void { this.analyticsService.trackEvent('button_click', { timestamp: new Date() }); } } ```
This approach allows you to capture and analyze custom metrics, providing insights into how users interact with your application.
4. Visualizing Performance Data with Third-Party Tools
Visualizing performance data can help you understand trends and patterns, making it easier to identify areas that need optimization. Angular can be integrated with third-party tools like Google Analytics or custom dashboards to visualize performance metrics.
Example: Integrating Google Analytics with Angular
To integrate Google Analytics with your Angular application, you can use the `ngx-google-analytics` library.
```bash ng add @angular/google-analytics ```
Once installed, you can track page views and events directly in your Angular components.
```typescript import { Component } from '@angular/core'; import { GoogleAnalyticsService } from 'ngx-google-analytics'; @Component({ selector: 'app-root', template: `<h1>Angular Performance Monitoring</h1>` }) export class AppComponent { constructor(private gaService: GoogleAnalyticsService) {} trackPageView(): void { this.gaService.pageView('/home', 'Home Page'); } } ```
This integration allows you to monitor user behavior and performance metrics in real-time.
Conclusion
Angular provides a comprehensive set of tools and techniques for performance monitoring. By leveraging Angular CLI commands, performance APIs, custom metrics, and third-party integrations, you can effectively monitor and optimize the performance of your Angular applications. Implementing these strategies will help you ensure that your applications remain responsive and efficient, providing a better user experience.
Further Reading
Table of Contents
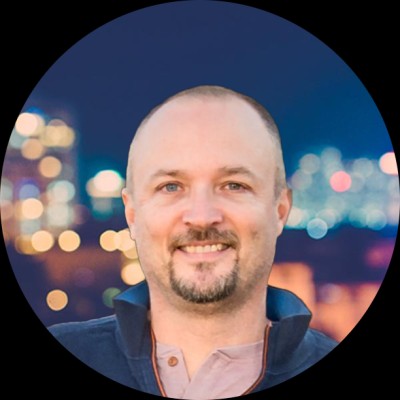
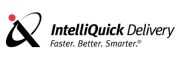