Enhancing Your Angular Application: 10 Performance Optimization Strategies for Faster Load Times
Angular is a robust, versatile, and popular web development framework that has gained significant traction in recent years. As the complexity of web applications increases, having a skilled team of Angular developers becomes even more crucial to effectively navigate the feature-rich environment of Angular. However, even with experienced Angular developers, apps created using this framework can sometimes face performance issues due to various reasons. To address these challenges, this article dives into key tips and techniques for optimizing Angular performance. Following these tips can ensure your applications run smoothly and efficiently, a testament to the skills and experience of your Angular developers. Hence, it’s not only important to hire Angular developers, but to make sure they’re adept at employing performance optimization techniques in Angular applications.
1. AOT Compilation
Ahead-of-Time (AOT) compilation is a technique that compiles your Angular app at the build time rather than at runtime. This means your browser loads executable code so it can render the app immediately, without waiting to compile the app first. The Angular AOT compiler also improves security by compiling HTML templates and components into JavaScript files before they’re served to the client.
```typescript ng build --prod --aot ```
Running this command enables AOT compilation, resulting in faster rendering, fewer asynchronous requests, smaller Angular framework download size, and improved detection of template errors.
2. Lazy Loading
Lazy loading is a design pattern that defers initialization of an object until it’s needed. In Angular, you can implement this for modules, loading them only when they’re needed, which can significantly improve load times.
To create a lazy-loaded feature module, you’d create a module with the Angular CLI and configure the routes to load lazily. Here’s a route configuration that lazy loads a module:
```typescript const routes: Routes = [ { path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) } ]; ```
This code instructs Angular to only load `FeatureModule` when the user navigates to “feature” in your app.
3. Change Detection Strategy
Angular’s default change detection mechanism is powerful but can lead to performance issues in large applications. By changing the change detection strategy to `OnPush`, Angular only checks the component when its input properties change or you manually ask it to check.
You can set this in your component like this:
```typescript @Component({ selector: 'app-my-component', templateUrl: './my-component.component.html', changeDetection: ChangeDetectionStrategy.OnPush }) export class MyComponent { } ```
4. TrackBy Function
Angular uses object identity to track insertions and deletions within the iterator and reproduce those changes in the DOM. However, if an object reference changes, Angular considers it a new object and re-renders the DOM element. This could lead to serious performance issues.
You can solve this problem by providing a `trackBy` function. It takes the index and current item as arguments and needs to return the unique identifier of this item:
```typescript @Component({ selector: 'app-my-component', template: ` <div *ngFor="let item of items; trackBy: trackByFn">{{item.id}}</div> `, }) export class MyComponent { items = [{id: 1}, {id: 2}, {id: 3}]; trackByFn(index, item) { return item.id; // unique id corresponding to the item } } ```
5. Web Workers
JavaScript is single-threaded, which can cause performance issues in heavy computational tasks. Web Workers make it possible to run a script operation in a background thread separate from the main execution thread. By using Web Workers in your Angular app, you can achieve parallelism and keep your UI interactive.
Web Workers can be integrated into Angular applications by using the `@angular/platform-webworker` package.
6. Use the Async Pipe
The Async pipe subscribes to an Observable or Promise and returns the latest value it has emitted. When a new
value is emitted, the async pipe marks the component to be checked for changes.
```html <div>{{ (observableData | async) }}</div> ```
This simple line will help reduce unnecessary subscriptions and memory leaks. The Async pipe unsubscribes automatically to avoid potential memory leaks, making your code cleaner and more maintainable.
7. Use enableProdMode
During development, Angular provides helpful error messages and checks. But in production, these checks can cause a performance hit. Enabling production mode disables the development specific checks and reduces the amount of generated code.
```typescript import {enableProdMode} from '@angular/core'; if (environment.production) { enableProdMode(); } ```
Remember to enable production mode when deploying your application to production.
8. Use Pure Pipes
In Angular, pipes allow you to transform output in your template. Angular offers two types of pipes – pure and impure. Pure pipes only run when Angular detects a change in the value or the parameters passed. This can significantly improve performance as it reduces the number of times the pipe needs to transform the data.
Here’s how you define a pure pipe:
```typescript @Pipe({ name: 'myPipe', pure: true }) export class MyPipe implements PipeTransform { transform(value: any, ...args: any[]): any { // Transform the value and return } } ```
9. Avoid Complex Computations in Templates
Angular templates are powerful and flexible tools in the hands of experienced Angular developers. However, they come with their limitations. Performing complex computations directly in templates can lead to performance issues as these computations run each time Angular runs change detection.
These challenges underscore the importance of hiring Angular developers who are well-versed in the idiosyncrasies of the framework. Experienced Angular developers are aware of these limitations and will implement strategies to minimize their impact. Instead of performing computations in templates, they will usually relegate this heavy lifting to the component class or a service, where it can be performed more efficiently.
Thus, hiring skilled and dedicated Angular developers isn’t just about utilizing the Angular framework, but more importantly, it’s about ensuring these professionals understand how to optimize their usage of the framework to create high-performing applications.
If you have a computation that is processor-intensive or has side-effects, consider moving it to the component class or a service.
For instance, instead of this:
```html <div>{{ calculateComplexValue() }}</div> ```
Do this:
```typescript export class MyComponent { complexValue: any; constructor() { this.complexValue = this.calculateComplexValue(); } calculateComplexValue() { // Perform complex computation here and return the value } } ``` ```html <div>{{ complexValue }}</div> ```
10. Compression
Last but not least, don’t forget to compress your code. Gzip compression can significantly reduce the size of your Angular app, thereby reducing the time it takes to download and load your app.
Conclusion
These tips and techniques should provide a solid foundation to improve the performance of your Angular applications. However, as with any optimization efforts, it’s essential to have a skilled team in place to execute these tasks efficiently. This is where the decision to hire Angular developers becomes crucial.
Experienced Angular developers will not only implement these performance-enhancing techniques but will also measure your application’s performance to identify bottlenecks and areas that need improvement. They can effectively use profiling tools, like the Chrome DevTools, to identify these issues and provide insights into how you can further optimize your Angular application.
Remember, the end goal of these optimizations is to create a faster app, which in turn leads to a better user experience and higher user engagement. And achieving this goal is much more feasible when you have the right team of Angular developers onboard.
Table of Contents
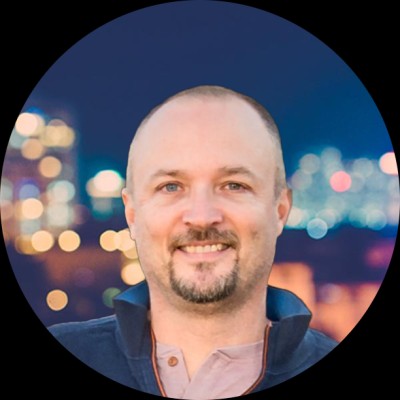
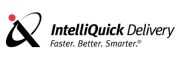