Angular and Performance Optimization: Techniques for Faster Apps
Angular is a powerful framework for building dynamic web applications. However, as applications grow in complexity, ensuring optimal performance becomes crucial for providing a seamless user experience. In this post, we’ll explore various techniques for optimizing the performance of Angular applications, helping you create faster and more efficient apps.
1. Lazy Loading Modules
One effective technique for improving Angular app performance is lazy loading modules. By lazy loading modules, you can split your application into smaller, manageable chunks and load them only when needed. This reduces the initial load time of your application, as users only download the code necessary for the current view.
To implement lazy loading in Angular, you can use the loadChildren property in your route configuration. This tells Angular to load the module asynchronously when the associated route is accessed. By strategically lazy loading modules, you can significantly improve the perceived performance of your application.
Example:
``` const routes: Routes = [ { path: 'dashboard', loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule) }, { path: 'profile', loadChildren: () => import('./profile/profile.module').then(m => m.ProfileModule) }, // Other routes... ]; ```
2. Tree Shaking
Tree shaking is a technique used to eliminate dead code from your application bundle. In Angular, tree shaking can help reduce the size of your bundle by removing unused dependencies and functions. This results in a smaller bundle size, faster download times, and improved performance.
To enable tree shaking in your Angular application, make sure you’re using the production build configuration (`ng build –prod`). Angular’s build tools automatically perform tree shaking when generating the production bundle, optimizing your application for performance.
Example:
``` ng build --prod ```
3. Change Detection Strategies
Angular’s default change detection strategy may not always be the most efficient option, especially for large and complex applications. By choosing the right change detection strategy, you can minimize unnecessary DOM updates and improve overall performance.
In Angular, you can configure change detection strategies at the component level using the ChangeDetectionStrategy enum. The OnPush strategy is particularly useful for optimizing performance, as it only triggers change detection when input properties change or when events are fired explicitly.
Example:
``` @Component({ selector: 'app-example', templateUrl: './example.component.html', changeDetection: ChangeDetectionStrategy.OnPush }) export class ExampleComponent {} ```
Conclusion
Optimizing the performance of Angular applications is essential for delivering fast and responsive user experiences. By implementing techniques such as lazy loading modules, tree shaking, and choosing the right change detection strategy, you can significantly improve the performance of your Angular apps. Keep experimenting with these techniques and stay updated with the latest best practices to ensure your apps remain fast and efficient.
External Links:
- Angular Documentation – Lazy Loading Modules – https://angular.io/guide/lazy-loading-ngmodules
- Webpack Documentation – Tree Shaking – https://webpack.js.org/guides/tree-shaking/
- Angular Change Detection Strategies – mhttps://angular.io/guide/change-detection
Table of Contents
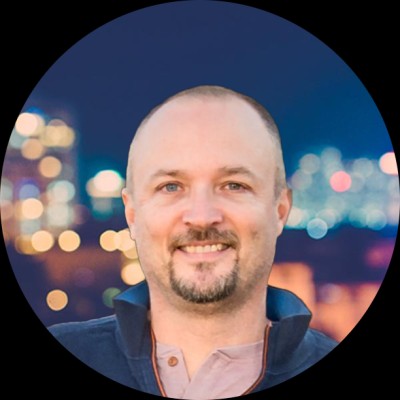
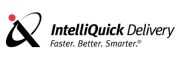