Angular and Reactive Programming with Redux-Observable
Reactive programming is a programming paradigm centered around data streams and the propagation of change. In Angular applications, reactive programming can be effectively utilized to manage state and handle asynchronous events, particularly when combined with tools like Redux-Observable. This blog explores how Redux-Observable enhances Angular’s state management by enabling reactive programming and provides practical examples to illustrate its implementation.
Understanding Reactive Programming in Angular
Reactive programming in Angular involves working with observables, which are a core part of Angular’s reactive forms and HTTP client. Observables allow for handling asynchronous events and data streams in a declarative manner. Integrating this approach with state management tools like Redux-Observable can greatly enhance the efficiency and maintainability of Angular applications.
Introduction to Redux-Observable
Redux-Observable is a middleware for Redux that enables the handling of side effects in a reactive way using RxJS observables. It allows developers to create Epics, which are functions that handle actions as streams, making it possible to compose complex asynchronous operations in a clean and concise manner.
Setting Up Redux-Observable in Angular
To start using Redux-Observable in your Angular application, you first need to install the necessary packages:
```bash npm install @ngrx/store @ngrx/effects redux-observable rxjs ```
Next, set up your Angular module to include the Redux store and the Redux-Observable middleware:
```typescript import { NgModule } from '@angular/core'; import { StoreModule } from '@ngrx/store'; import { EffectsModule } from '@ngrx/effects'; import { rootReducer } from './reducers'; import { ExampleEffects } from './effects/example.effects'; @NgModule({ imports: [ StoreModule.forRoot({ appState: rootReducer }), EffectsModule.forRoot([ExampleEffects]), ], }) export class AppModule {} ```
Creating Epics with Redux-Observable
An Epic is a function that takes a stream of actions and returns a stream of actions. Here’s a simple example of an Epic that handles an action to fetch data:
```typescript import { Injectable } from '@angular/core'; import { Actions, createEffect, ofType } from '@ngrx/effects'; import { HttpClient } from '@angular/common/http'; import { catchError, map, mergeMap } from 'rxjs/operators'; import { of } from 'rxjs'; import * as exampleActions from '../actions/example.actions'; @Injectable() export class ExampleEffects { fetchData$ = createEffect(() => this.actions$.pipe( ofType(exampleActions.fetchData), mergeMap(() => this.httpClient.get('/api/data').pipe( map(data => exampleActions.fetchDataSuccess({ data })), catchError(() => of(exampleActions.fetchDataFailure())) ) ) ) ); constructor(private actions$: Actions, private httpClient: HttpClient) {} } ```
In this example:
– `ofType` filters the actions to only pass through those that match the given type.
– `mergeMap` is used to perform an HTTP request when the action is dispatched.
– The result of the HTTP request is then mapped to a success or failure action.
Managing State with Redux-Observable
Redux-Observable fits neatly into the Redux architecture of Angular applications, allowing you to manage state reactively. Here’s how you can define a simple reducer and corresponding actions:
```typescript import { createReducer, on } from '@ngrx/store'; import * as exampleActions from '../actions/example.actions'; export interface AppState { data: any[]; loading: boolean; error: any; } export const initialState: AppState = { data: [], loading: false, error: null, }; export const rootReducer = createReducer( initialState, on(exampleActions.fetchData, state => ({ ...state, loading: true, })), on(exampleActions.fetchDataSuccess, (state, { data }) => ({ ...state, data, loading: false, })), on(exampleActions.fetchDataFailure, state => ({ ...state, loading: false, error: 'Failed to fetch data', })) ); ```
Best Practices for Using Redux-Observable in Angular
- Keep Epics Pure: Ensure that your Epics are pure functions and do not cause side effects directly. Use observables to handle asynchronous operations.
- Use Operators Wisely: RxJS provides a wide range of operators for transforming observables. Choose the right operator for the right task, such as `mergeMap`, `switchMap`, or `concatMap`.
- Handle Errors Gracefully: Always handle errors in your Epics by catching them and dispatching failure actions to manage the state accordingly.
- Modularize Epics: Break down large Epics into smaller, reusable ones to keep your code maintainable and testable.
Conclusion
Redux-Observable brings the power of reactive programming to Angular, enabling efficient state management and handling of asynchronous actions. By leveraging Epics and RxJS, developers can create scalable and maintainable Angular applications that are responsive to dynamic data streams and user interactions.
Further Reading
Table of Contents
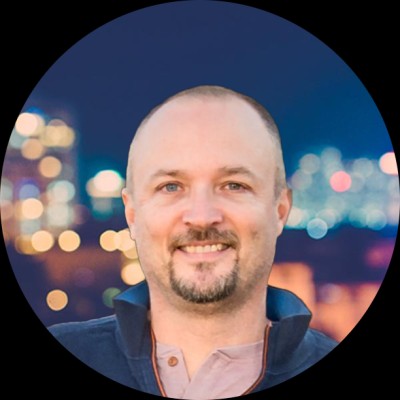
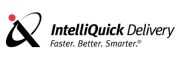