Exploring Angular Reactive Programming: Observables and Operators
In the world of web development, building dynamic and interactive applications is at the forefront of every developer’s mind. Angular, a popular JavaScript framework, provides developers with the tools they need to create such applications efficiently. One of the key features that sets Angular apart is its robust support for reactive programming through the use of Observables and Operators.
Table of Contents
Reactive programming is a programming paradigm that deals with asynchronous data streams. It allows developers to work with data that changes over time in a clean and predictable manner. In this blog post, we will dive deep into Angular Reactive Programming, focusing on Observables and Operators, and explore how they can enhance your web development skills.
1. Understanding Observables
1.1. What Are Observables?
At its core, an Observable is a data stream that emits a sequence of values over time. These values can be of any data type, such as numbers, strings, or custom objects. Observables are a fundamental concept in Angular’s reactive programming model and play a pivotal role in handling asynchronous data.
Here’s a basic example of creating an Observable in Angular:
typescript import { Observable } from 'rxjs'; const myObservable = new Observable<number>((observer) => { observer.next(1); observer.next(2); observer.next(3); observer.complete(); });
In this example, we create an Observable that emits the numbers 1, 2, and 3, and then completes the stream.
1.2. Subscribing to Observables
Observables are lazy by nature, which means they won’t emit values until someone subscribes to them. To consume the values emitted by an Observable, you need to subscribe to it. Here’s how you can do it:
typescript myObservable.subscribe({ next: (value) => console.log(value), complete: () => console.log('Observable completed.'), });
The subscribe method takes an object with functions for handling emitted values (next) and completion events (complete).
3. Operators in Angular Reactive Programming
3.1. What Are Operators?
Operators are functions that allow you to transform, filter, or combine Observables in various ways. They provide a powerful toolkit for manipulating data streams and are an essential part of reactive programming in Angular.
Angular leverages the RxJS library to provide a wide range of operators that you can use with Observables. Let’s explore some commonly used operators and see how they can enhance your Angular applications.
3.1.1. map Operator
The map operator allows you to transform the values emitted by an Observable into a new shape. This is incredibly useful when you want to modify the data before it reaches the subscriber. Here’s an example:
typescript import { of } from 'rxjs'; import { map } from 'rxjs/operators'; const numbers = of(1, 2, 3, 4, 5); const squaredNumbers = numbers.pipe( map((value) => value * value) ); squaredNumbers.subscribe((result) => console.log(result));
In this code snippet, we start with an Observable of numbers and use the map operator to square each number before it is delivered to the subscriber. The output will be the squared values: 1, 4, 9, 16, and 25.
3.1.2. filter Operator
The filter operator, as the name suggests, allows you to filter the values emitted by an Observable based on a given condition. Here’s an example:
typescript import { of } from 'rxjs'; import { filter } from 'rxjs/operators'; const numbers = of(1, 2, 3, 4, 5); const evenNumbers = numbers.pipe( filter((value) => value % 2 === 0) ); evenNumbers.subscribe((result) => console.log(result));
In this case, we start with an Observable of numbers and use the filter operator to only emit the even numbers. The output will be 2 and 4.
3.1.3. mergeMap Operator
The mergeMap operator is used to merge multiple Observables into a single stream. This is handy when you have multiple sources of asynchronous data that you want to combine. Here’s an example:
typescript import { of, interval } from 'rxjs'; import { mergeMap } from 'rxjs/operators'; const source = of('A', 'B', 'C'); const intervalObservable = interval(1000); const merged = source.pipe( mergeMap((letter) => intervalObservable.pipe(map((i) => `${letter}${i}`))) ); merged.subscribe((result) => console.log(result));
In this example, we have an Observable source that emits letters ‘A’, ‘B’, and ‘C’, and an intervalObservable that emits numbers every second. The mergeMap operator combines these two Observables, resulting in the output of combinations like ‘A0’, ‘B0’, ‘C0’, ‘A1’, ‘B1’, and so on.
3.1.4. combineLatest Operator
The combineLatest operator is used to combine the latest values from multiple Observables into a single stream whenever any of the source Observables emit a new value. This is useful when you need data from multiple sources to work together. Here’s an example:
typescript import { of, interval } from 'rxjs'; import { combineLatest } from 'rxjs/operators'; const source1 = of('A', 'B', 'C'); const source2 = interval(1000); const combined = combineLatest([source1, source2]); combined.subscribe(([letter, number]) => console.log(`${letter}${number}`));
In this code snippet, combineLatest combines the latest values emitted by source1 and source2, resulting in output like ‘A0’, ‘B0’, ‘B1’, ‘C1’, and so on.
4. Putting It All Together: A Real-World Example
To illustrate the power of Observables and Operators in Angular, let’s consider a real-world scenario: fetching and displaying data from an API.
Suppose you have an Angular component that needs to fetch a list of user profiles from a remote API and display them in your application. Here’s how you can achieve this using Observables and Operators:
typescript import { Component, OnInit } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; import { map } from 'rxjs/operators'; interface UserProfile { id: number; name: string; email: string; } @Component({ selector: 'app-user-list', templateUrl: './user-list.component.html', styleUrls: ['./user-list.component.css'] }) export class UserListComponent implements OnInit { users$: Observable<UserProfile[]>; constructor(private http: HttpClient) {} ngOnInit() { this.users$ = this.http.get<UserProfile[]>('https://api.example.com/users').pipe( map(response => response.map(user => ({ id: user.id, name: user.name, email: user.email }))) ); } }
In this example, we create an Angular component called UserListComponent. In the ngOnInit method, we use the Angular HttpClient to make an HTTP GET request to an API endpoint that returns a list of user profiles. We then apply the map operator to transform the API response into a more manageable format before assigning it to the users$ property. This property is bound to the template to display the user profiles in the UI.
By utilizing Observables and the map operator, we’ve created a clean and efficient way to handle asynchronous data in our Angular application.
Conclusion
Angular Reactive Programming, powered by Observables and Operators, is a game-changer for web developers. It provides a structured and powerful approach to handling asynchronous data, making it easier to create dynamic and responsive applications.
In this blog post, we’ve explored the fundamentals of Observables, how to subscribe to them, and some commonly used Operators like map, filter, mergeMap, and combineLatest. We’ve also demonstrated their practical use in a real-world example, fetching and displaying data from an API.
As you continue your journey with Angular, mastering reactive programming with Observables and Operators will be an invaluable skill. It opens up a world of possibilities for building feature-rich and responsive web applications. So, go ahead, experiment with Observables and Operators, and unlock the full potential of Angular in your projects. Happy coding!
Table of Contents
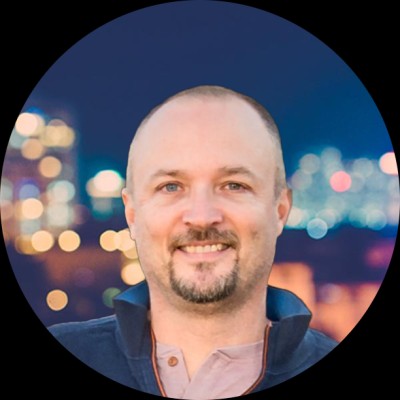
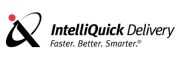