Securing Your Angular Applications: Best Practices and Techniques
In the rapidly evolving landscape of web development, building secure applications is of paramount importance. With Angular being one of the most popular front-end frameworks, it’s crucial to understand the best practices and techniques to secure your Angular applications. This blog will provide you with comprehensive insights into securing your Angular apps, safeguarding sensitive data, and protecting against common vulnerabilities.
Table of Contents
1. Why Security Matters for Angular Applications
Before diving into the best practices, let’s understand why security is a top priority for Angular applications.
1.1. Importance of Security
Angular applications often handle sensitive user data and execute critical functionalities, making them a prime target for cyberattacks. Security breaches can result in significant data leaks, unauthorized access, and loss of user trust. By prioritizing security, you not only protect your users but also safeguard your reputation as a developer or organization.
1.2. Common Security Threats
Some common security threats faced by Angular applications include:
- Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages viewed by other users, compromising their data and sessions.
- Cross-Site Request Forgery (CSRF): This attack forces users to perform actions they didn’t intend, potentially leading to unauthorized operations.
- Injection Attacks: Attackers exploit vulnerabilities in the application to inject malicious code, often leading to data breaches.
- Authentication and Authorization Issues: Weak authentication mechanisms or improper authorization can grant unauthorized access to sensitive resources.
2. Angular Security Best Practices
Let’s explore essential security best practices to enhance the security posture of your Angular applications.
2.1. Keep Angular and Dependencies Updated
Regularly updating Angular and its dependencies is crucial to stay protected against known vulnerabilities. The Angular team and the open-source community actively work on fixing security issues, so ensure your project uses the latest stable versions.
Code Sample: Update Angular and its dependencies using npm (Node Package Manager).
bash npm update @angular/core
2.2. Enable Strict Content Security Policy (CSP)
Content Security Policy (CSP) helps prevent XSS attacks by controlling which resources can be loaded on a web page. Enabling strict CSP directives ensures that only trusted sources are allowed to execute scripts, reducing the risk of malicious script execution.
Code Sample: Add a CSP meta tag to your index.html file.
html <meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' https://trusted-cdn.com;">
2.3. Use Angular’s Built-in Security Mechanisms
Angular provides built-in security mechanisms that help prevent common attacks. Utilize features like Angular’s template syntax, which automatically escapes unsafe data, mitigating XSS vulnerabilities.
Code Sample: Angular automatically escapes unsafe data in templates.
typescript import { Component } from '@angular/core'; @Component({ selector: 'app-blog', template: ` <div> <h1>Welcome, {{ username }}</h1> <!-- Username will be automatically escaped --> </div> `, }) export class BlogComponent { username = '<script>alert("XSS Attack!")</script>'; }
2.4. Implement Secure Authentication and Authorization
Secure authentication and authorization are fundamental for protecting sensitive resources. Use strong hashing algorithms for password storage, enforce password policies, and consider implementing multi-factor authentication for enhanced security.
Code Sample: Authenticate users using Angular’s authentication service.
typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class AuthService { private users = [ { username: 'user1', passwordHash: 'hashed_password_1' }, { username: 'user2', passwordHash: 'hashed_password_2' }, // Add more users... ]; login(username: string, password: string): boolean { const user = this.users.find((u) => u.username === username); if (user && this.validatePassword(password, user.passwordHash)) { // User is authenticated return true; } return false; } private validatePassword(password: string, passwordHash: string): boolean { // Implement strong password validation logic and hash comparison // For demonstration purposes, we'll just compare the plain password return password === passwordHash; } }
2.5. Sanitize User Input
Sanitizing user input is crucial to prevent injection attacks. Utilize Angular’s built-in DOM Sanitizer to sanitize user-generated content before rendering it on the page.
Code Sample: Sanitize user input using Angular’s DomSanitizer.
typescript import { Component } from '@angular/core'; import { DomSanitizer } from '@angular/platform-browser'; @Component({ selector: 'app-blog', template: ` <div [innerHTML]="unsafeHTML"></div> `, }) export class BlogComponent { unsafeHTML = '<script>alert("XSS Attack!")</script>'; constructor(private sanitizer: DomSanitizer) {} get safeHTML() { return this.sanitizer.bypassSecurityTrustHtml(this.unsafeHTML); } }
3. Securing Communication with APIs
Securing communication between your Angular app and APIs is vital to protect sensitive data and prevent unauthorized access.
3.1. Use HTTPS
Always use HTTPS for communication to encrypt data exchanged between the client and the server, preventing data interception and tampering.
3.2. Implement CORS (Cross-Origin Resource Sharing) Protection
Configure your server to allow requests only from trusted origins using CORS headers. This mitigates unauthorized cross-origin requests from other domains.
Code Sample: Configure CORS in your backend server.
typescript const express = require('express'); const app = express(); // Allow requests only from trusted origins const allowedOrigins = ['https://example.com', 'https://subdomain.example.com']; app.use((req, res, next) => { const origin = req.headers.origin; if (allowedOrigins.includes(origin)) { res.setHeader('Access-Control-Allow-Origin', origin); } res.header('Access-Control-Allow-Headers', 'Origin, X-Requested-With, Content-Type, Accept'); next(); });
4. Protecting Sensitive Data
4.1. Encrypt Sensitive Data
Encrypt sensitive data on the client-side before sending it to the server, ensuring data confidentiality even if intercepted during transmission.
4.2. Avoid Storing Sensitive Data on the Client
Avoid storing sensitive data on the client-side. If required, utilize secure storage mechanisms like HTTP-only cookies for tokens and credentials.
Conclusion
In conclusion, securing your Angular applications is an ongoing process that demands constant vigilance and adherence to best practices. By keeping your Angular and its dependencies up-to-date, leveraging Angular’s built-in security features, implementing secure authentication and authorization, and protecting communication with APIs, you can significantly enhance the security posture of your applications. Remember to sanitize user input, encrypt sensitive data, and avoid storing sensitive information on the client. By following these best practices and staying informed about emerging security threats, you can build robust and secure Angular applications that safeguard both your users and your reputation as a developer.
Remember, security is not a one-time task but an ongoing journey of continuous improvement to stay ahead of malicious actors and protect your users’ valuable data. Stay informed, be proactive, and always prioritize security in your Angular projects.
Table of Contents
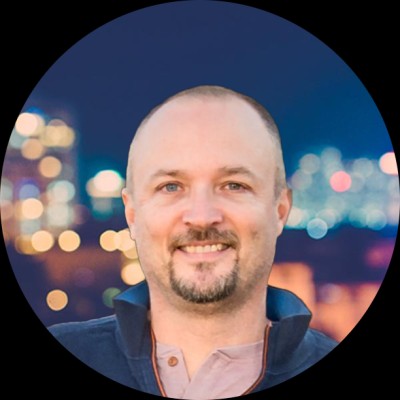
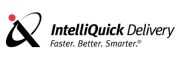