Demystifying Angular Services: A Comprehensive Guide for Seamless Data Management Across Your Application
Angular is a robust platform that enables developers, including those you might hire Angular developers, to build intricate web applications. One critical aspect that these developers work with is Angular services. Services constitute a significant part of Angular applications. They not only streamline application functionality but also play an indispensable role in sharing data and logic across components, enhancing the overall efficiency of your app.
What are Angular Services?
Angular Services are classes with a specific purpose and use. They contain methods that maintain data throughout the life of an application, i.e., data persistence. Services can be injected into components as dependencies, making your code modular, reusable, and efficient.
The primary role of a service is to organize and share code across your application. An Angular service is a singleton, meaning that there’s only one instance of it throughout the application.
Let’s look at some practical examples of Angular services and understand their power and capabilities.
Creating a Basic Angular Service
First, let’s create a simple service. We’ll use the Angular CLI command `ng generate service data`:
```typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class DataService { constructor() { } getData() { return 'Hello, World!'; } } ```
In the example above, the `DataService` has a `getData()` method that returns a string. The `@Injectable()` decorator indicates that this class can be provided and injected as a dependency in other components.
Using Angular Service in a Component
To use this service in a component, you’ll need to import it and add it to the component’s constructor as a parameter:
```typescript import { Component } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title: string; constructor(private dataService: DataService) { this.title = this.dataService.getData(); } } ```
In the `AppComponent` class, we’re injecting the `DataService` in the constructor. We can then call the `getData()` method from `DataService` to set the value of `title`.
Sharing Data between Components
Services can also be used to share data between components – a skill well mastered by professional Angular developers you might hire. For instance, envision a scenario where you have two components — a list component and a detail component. A hallmark of a well-crafted Angular application, possibly crafted by skilled Angular developers, is seamless interaction between components. When you select an item in the list component, the application, as per this ideal, should effortlessly display the details of the selected item in the detail component.
Here’s how you could create a service to facilitate this:
```typescript import { Injectable } from '@angular/core'; import { BehaviorSubject } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class SharedDataService { private data = new BehaviorSubject<any>(null); sharedData = this.data.asObservable(); constructor() { } updateData(data: any) { this.data.next(data); } } ```
In this `SharedDataService`, we’re using a `BehaviorSubject` from RxJS. A `BehaviorSubject` is a type of subject, a special type of observable, that allows subscribers to always get the last (or initial) value.
In our two components, we can inject this service and use it to share data:
```typescript import { Component } from '@angular/core'; import { SharedDataService } from './shared-data.service'; @Component({ selector: 'app-list', templateUrl: './list.component.html', styleUrls: ['./list.component.css'] }) export class ListComponent { constructor(private sharedDataService: SharedDataService) { } selectItem(item: any) { this.sharedDataService.updateData(item); } } ``` ```typescript import { Component, OnInit } from '@angular/core'; import { SharedDataService } from './shared -data.service'; @Component({ selector: 'app-detail', templateUrl: './detail.component.html', styleUrls: ['./detail.component.css'] }) export class DetailComponent implements OnInit { item: any; constructor(private sharedDataService: SharedDataService) { } ngOnInit() { this.sharedDataService.sharedData.subscribe(data => { this.item = data; }); } } ```
In the `ListComponent`, when an item is selected, it calls the `updateData()` method of `SharedDataService`. The `DetailComponent` subscribes to `sharedData` from `SharedDataService` and sets its `item` property whenever the shared data is updated.
Conclusion
Angular Services are a powerful way to organize and share code across your application. They enable efficient data management, ensuring your components stay lean and focused on presenting data. With a deep understanding of how to utilize services, you can create more maintainable and scalable Angular applications.
While we’ve only just begun to explore the capabilities of Angular services, these examples should offer a solid foundation for grasping their vital role in Angular applications. As you delve further into Angular or decide to hire Angular developers, you’ll discover more ways to leverage services. These include handling HTTP operations, managing user authentication, and interfacing with local storage, among others. Remember, the principal objective of services, often leveraged by professional Angular developers, is to ensure your components remain clean, testable, and intuitive. This approach allows you to focus on the unique functionality of your application, improving both user experience and overall efficiency.
Table of Contents
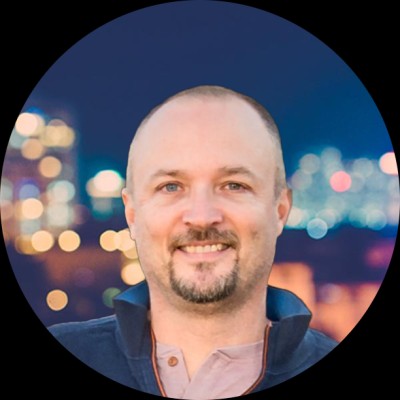
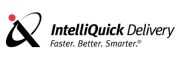