Angular State Management with Akita: Simplifying Complex Apps
Managing state in large Angular applications can be challenging, especially as the complexity of the app grows. Akita, a state management library for Angular, provides a simple yet powerful way to manage state, making it easier to build and maintain complex applications. This blog will explore how Akita simplifies state management in Angular, with practical examples to help you integrate it into your projects.
Understanding State Management in Angular
State management is crucial in Angular applications for maintaining and updating the application’s state across different components. Without an efficient state management solution, handling the state of large applications can lead to issues like inconsistent data, difficult debugging, and code that is hard to maintain.
Why Choose Akita for State Management?
Akita offers a structured approach to state management, focusing on simplicity, scalability, and testability. It provides a straightforward API and integrates seamlessly with Angular, making it an ideal choice for developers looking to manage complex state in their applications.
Setting Up Akita in Your Angular Project
Before diving into state management, you’ll need to set up Akita in your Angular project. The following steps will guide you through the installation and basic configuration.
```bash npm install @datorama/akita ```
Once installed, you can start setting up stores, queries, and services to manage your application state.
1. Creating a Store with Akita
A store in Akita is where you manage the state of a particular feature or domain in your application. Here’s how you can create a basic store in an Angular application.
```typescript import { Injectable } from '@angular/core'; import { EntityState, EntityStore, StoreConfig } from '@datorama/akita'; export interface Product { id: number; name: string; price: number; } export interface ProductState extends EntityState<Product, number> {} @Injectable({ providedIn: 'root' }) @StoreConfig({ name: 'products' }) export class ProductStore extends EntityStore<ProductState, Product> { constructor() { super(); } } ```
This example defines a `ProductStore` for managing the state of products in your application.
2. Querying the State with Akita
Queries in Akita are used to retrieve data from the store. They provide a way to select specific parts of the state and listen for changes.
```typescript import { Injectable } from '@angular/core'; import { QueryEntity } from '@datorama/akita'; import { ProductStore, ProductState } from './product.store'; @Injectable({ providedIn: 'root' }) export class ProductQuery extends QueryEntity<ProductState> { constructor(protected store: ProductStore) { super(store); } selectProducts() { return this.selectAll(); } selectProductPrice(productId: number) { return this.selectEntity(productId).price; } } ```
In this example, the `ProductQuery` class is used to retrieve all products and specific product prices from the store.
3. Updating State with Akita
Updating the state in Akita is done through the store. Here’s an example of how you can update the state of a product in the store.
```typescript import { Injectable } from '@angular/core'; import { ProductStore } from './product.store'; @Injectable({ providedIn: 'root' }) export class ProductService { constructor(private productStore: ProductStore) {} updateProduct(id: number, product: Partial<Product>) { this.productStore.update(id, product); } addProduct(product: Product) { this.productStore.add(product); } removeProduct(id: number) { this.productStore.remove(id); } } ```
This `ProductService` class demonstrates how to update, add, and remove products from the store.
4. Visualizing State Changes
Visualizing state changes can be beneficial for debugging and understanding the flow of data within your application. Akita provides tools like `Akita Devtools` to help visualize state changes.
```typescript import { AkitaNgDevtools } from '@datorama/akita-ngdevtools'; import { environment } from '../environments/environment'; @NgModule({ imports: [ AkitaNgDevtools.forRoot() ], }) export class AppModule {} ```
This integration allows you to monitor state changes in real-time within your browser, making it easier to debug and optimize your application.
Conclusion
Akita offers a robust and scalable solution for state management in Angular applications, particularly when dealing with complex state logic. By providing a clear structure and powerful API, Akita simplifies the process of managing state, leading to cleaner, more maintainable code. Integrating Akita into your Angular projects can greatly enhance the efficiency and scalability of your applications.
Further Reading:
Table of Contents
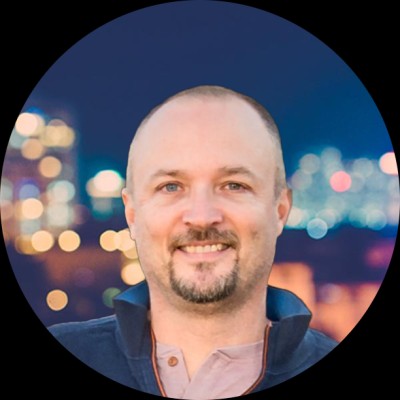
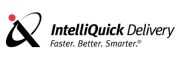