Crafting User-Friendly Interfaces with C# GUI Design
When it comes to developing graphical user interface (GUI) applications for Windows, .NET Framework with C# and Windows Forms (WinForms) is a powerful combination that makes GUI development an approachable task even for beginners. This is one of the reasons why businesses often opt to hire C# developers. This article will guide you through the process of building a simple GUI application using C# and WinForms, showcasing the skills you might look for when you hire C# developers.
Introduction
C# is a modern, object-oriented programming language developed by Microsoft that runs on the .NET Framework. WinForms is a set of classes in the .NET Framework that provides a platform to develop rich client applications for desktop, laptop, and tablets. WinForms offers an extensive UI framework that is straightforward to learn and provides a robust set of tools to create complex applications.
Setup
Before we start building the application, you need to have the necessary tools installed. You will need Visual Studio, a development environment where you’ll write and test your code. As of the cut-off knowledge in September 2021, Visual Studio 2019 is the latest stable version, and you can download it from the official Microsoft website.
Creating a New Project
Once Visual Studio is installed, launch it, and let’s start creating a new project.
- Click on “Create a new project”.
- Select “Windows Forms App (.NET Framework)” and click “Next”.
- Name your project, choose a location to save it, and click “Create”.
After a few seconds, Visual Studio generates a new WinForms project for you. The main components are Form1.cs, which is the primary window of your application, and Program.cs, which is the entry point for the application.
Understanding the Interface
Before we delve into creating our application, let’s understand the Visual Studio interface.
– Solution Explorer: This pane, usually on the right side, contains all the files and resources in your project.
– Properties Window: Below the Solution Explorer, this window displays properties of the selected form or control.
– Toolbox: It contains a list of controls or components that you can drag and drop onto your form.
– Form Designer: The central part of the interface where you can design your form’s UI.
Building Our Application
Our application will be a simple form where users can enter their name and click a button to display a welcome message. Here are the steps:
- In the Solution Explorer, double-click on Form1.cs. This action will open the Form Designer.
- In the Toolbox, find the “Label” control and drag it onto the form. This label will be used to prompt users to enter their name.
- In the Properties window, change the Text property of the label to “Enter your name:”.
- Now, drag a “TextBox” control from the Toolbox onto the form, positioning it next to the label. This textbox is where users will input their name.
- Drag a “Button” control from the Toolbox onto the form. Change its Text property to “Display Welcome Message”.
We have now created the UI for our application. It’s time to write the C# code that will be executed when the button is clicked.
- Double-click on the button in the Form Designer. This action will open the code editor with a new method, `button1_Click`. This method will be called every time the button is clicked.
- Inside this method, write the following code:
```csharp string name = textBox1.Text; MessageBox.Show("Welcome, " + name + "!", "Welcome");
This code retrieves the text from the textbox and displays it in a message box with a welcome message. Save your changes and run your application by pressing F5 or clicking the “Start Debugging” button. Test it out by entering your name and clicking the button.
Enhancing Our Application
The application works fine, but let’s add a little error handling to make it more robust. If a user clicks the button without entering a name, we should display an error message.
Modify your button click method to look like this:
```csharp string name = textBox1.Text; if(string.IsNullOrEmpty(name)) { MessageBox.Show("Please enter your name.", "Error"); } else { MessageBox.Show("Welcome, " + name + "!", "Welcome"); }
Now, if the textbox is empty when the button is clicked, an error message will be displayed. Run the application again and test this new functionality.
Conclusion
In this article, we’ve created a simple GUI application using C#, WinForms, and Visual Studio. This demonstration underlines why many businesses choose to hire C# developers. We’ve seen how to use various controls, such as labels, textboxes, and buttons, and how to handle button click events. We’ve also added basic error handling to our application.
Building GUI applications with C# and WinForms is straightforward and approachable, even for beginners. It provides a rich set of tools and controls that make it easy to design and create complex applications. These skill sets are exactly what you gain when you hire C# developers. With this foundation, you can start building more complex applications and exploring the many other features that WinForms offers.
Remember, practice is the key to mastery. So keep building, keep experimenting, and happy coding!
Table of Contents
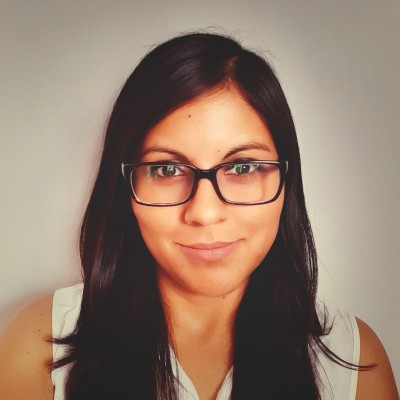
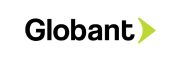