How to configure Entity Framework Core using Fluent API in C#?
Configuring Entity Framework Core (EF Core) using Fluent API in C# provides fine-grained control over how your entity classes map to database tables and how relationships between entities are defined. Here’s a step-by-step guide on how to configure EF Core using Fluent API:
- Create a DbContext:
Start by creating a class that inherits from `DbContext`, which represents your database context. This class will contain DbSet properties for your entity classes and the configuration using Fluent API.
```csharp public class YourDbContext : DbContext { public DbSet<EntityType> EntityTypes { get; set; } // DbSet properties for other entities protected override void OnModelCreating(ModelBuilder modelBuilder) { // Fluent API configuration goes here } } ```
- Configure Entity Properties:
Inside the `OnModelCreating` method, you can configure entity properties using Fluent API. For example, to specify a maximum length for a string property, you can use the `HasMaxLength` method:
```csharp modelBuilder.Entity<EntityType>() .Property(e => e.PropertyName) .HasMaxLength(50); ```
- Define Primary Keys:
To define primary keys for your entities, use the `HasKey` method:
```csharp modelBuilder.Entity<EntityType>() .HasKey(e => e.Id); ```
- Configure Relationships:
Use Fluent API to configure relationships between entities. For instance, to define a one-to-many relationship, use `HasOne` and `WithMany`:
```csharp modelBuilder.Entity<Author>() .HasMany(a => a.Books) .WithOne(b => b.Author); ```
- Specify Table Names:
You can set custom table names using the `ToTable` method:
```csharp modelBuilder.Entity<EntityType>() .ToTable("CustomTableName"); ```
- Indexes and Constraints:
To define indexes and constraints, use methods like `HasIndex` and `HasCheckConstraint`:
```csharp modelBuilder.Entity<EntityType>() .HasIndex(e => e.SomeProperty) .IsUnique(); modelBuilder.Entity<EntityType>() .HasCheckConstraint("CheckConstraintName", "SomeProperty > 0"); ```
- Seed Data:
You can also seed initial data using Fluent API. Override the `OnModelCreating` method and use the `HasData` method:
```csharp modelBuilder.Entity<EntityType>() .HasData( new EntityType { Id = 1, Property1 = "Value1", Property2 = "Value2" }, // Add more seed data ); ```
By following these steps and utilizing Fluent API in EF Core, you can tailor the database schema and entity behavior to your specific application requirements. It offers a powerful way to configure your data model with precision and flexibility.
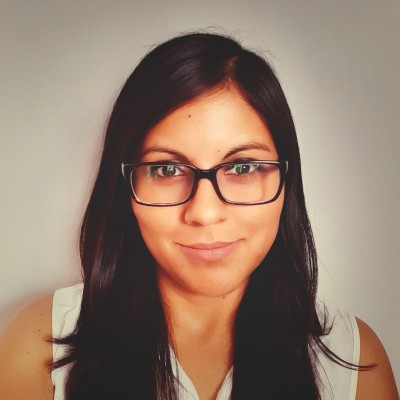
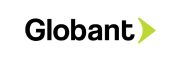