How to document my API with Swagger in ASP.NET Core?
Documenting your API with Swagger in ASP.NET Core is a smart choice to provide comprehensive and interactive documentation for your web services. Swagger simplifies the process of documenting your API endpoints, making it easier for both developers and consumers to understand how your API works. Here’s a step-by-step guide on how to document your API with Swagger in ASP.NET Core:
- Install the Swagger NuGet Package:
– Open your ASP.NET Core project in Visual Studio.
– Go to the “Tools” menu and select “NuGet Package Manager,” then choose “Manage NuGet Packages for Solution.”
– Search for and install the “Swashbuckle.AspNetCore” package, which is the official Swagger package for ASP.NET Core.
- Configure Swagger in Startup.cs:
– In your ASP.NET Core project, open the `Startup.cs` file.
– Inside the `ConfigureServices` method, add the following code to configure Swagger:
```csharp services.AddSwaggerGen(c => { c.SwaggerDoc("v1", new OpenApiInfo { Title = "Your API Name", Version = "v1" }); }); ```
Replace “Your API Name” with your actual API’s name.
– In the `Configure` method, add the following code to enable Swagger middleware and specify the Swagger JSON endpoint:
```csharp app.UseSwagger(); app.UseSwaggerUI(c => { c.SwaggerEndpoint("/swagger/v1/swagger.json", "Your API Name v1"); }); ```
- Add Swagger Annotations to Your API Controllers:
– In your API controller classes, use Swagger annotations (attributes) to provide information about your API endpoints. For example:
```csharp [Route("api/[controller]")] [ApiController] public class UsersController : ControllerBase { [HttpGet] [SwaggerOperation(Summary = "Get a list of all users")] [ProducesResponseType(StatusCodes.Status200OK)] public IActionResult GetAllUsers() { // Your API logic here } } ```
- Generate Documentation:
– Build and run your ASP.NET Core application.
– Access the Swagger documentation by navigating to `https://yourdomain.com/swagger/index.html` in your web browser.
- Explore and Test Your API:
– In the Swagger documentation, you can explore your API endpoints, see their descriptions, and even test them by providing input parameters and observing the responses.
- Customize Swagger Documentation (Optional):
– You can customize the generated Swagger documentation further by adding descriptions, grouping endpoints, or providing additional information using Swagger attributes.
By following these steps, you can easily document your API with Swagger in ASP.NET Core, enhancing the developer experience and making it easier for others to consume your web services.
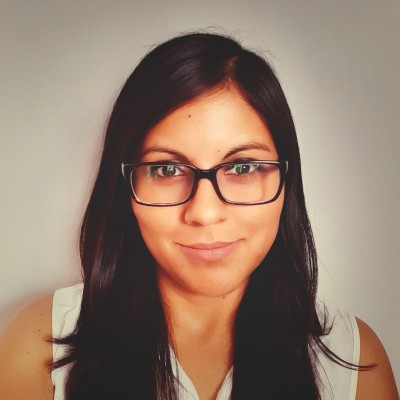
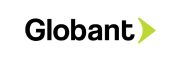