How to implement authentication and authorization in C#?
Implementing authentication and authorization in a C# application is crucial for securing your application’s resources and ensuring that only authorized users can access certain parts of it. Here’s a high-level overview of how to implement authentication and authorization in C#:
Authentication:
Authentication is the process of verifying the identity of a user. In C# applications, you can implement authentication using frameworks like ASP.NET Identity or external identity providers like OAuth or OpenID Connect. Here are the steps to implement authentication:
- Choose an Authentication Method: Decide on the authentication method that best suits your application’s needs, such as username and password, social media logins, or multi-factor authentication (MFA).
- Implement Authentication Middleware: Integrate authentication middleware into your application’s pipeline. In ASP.NET, this can be done through the `Startup.cs` file using middleware like `UseAuthentication()`.
- User Registration: Implement a user registration process, allowing users to create accounts with unique usernames and secure passwords. Use hashing algorithms to securely store passwords.
- Login: Create a login mechanism where users can provide their credentials (username and password) or use external providers like Google or Facebook for single sign-on (SSO).
- Token-Based Authentication: For APIs or stateless applications, consider using token-based authentication like JWT (JSON Web Tokens) to issue and validate tokens for secure communication.
Authorization:
Authorization controls what actions or resources authenticated users can access. It defines roles, permissions, and policies that determine access levels. Here’s how to implement authorization:
- Define Roles and Permissions: Identify roles (e.g., admin, user) and assign permissions (e.g., read, write) to them. Roles and permissions can be stored in a database or configuration files.
- Role-Based Authorization: Implement role-based authorization by associating users with roles. Use attributes or middleware to restrict access to specific controllers or actions based on roles.
- Claims-Based Authorization (Optional): For fine-grained control, use claims-based authorization, where user claims (attributes) define access. Claims can be associated with users during authentication.
- Policy-Based Authorization: ASP.NET Core provides policy-based authorization, where you define policies and apply them to controllers or actions. Policies are flexible and allow complex authorization rules.
- Handle Unauthorized Access: Implement logic to handle unauthorized access, such as redirecting to a login page or returning a forbidden (403) status code.
- Testing: Thoroughly test your authentication and authorization mechanisms to ensure they work as expected. Test both authorized and unauthorized scenarios.
By following these steps and using appropriate authentication and authorization mechanisms, you can secure your C# application and ensure that only authorized users can access protected resources. Security is an ongoing concern, so regularly update and monitor your authentication and authorization systems to stay protected against emerging threats.
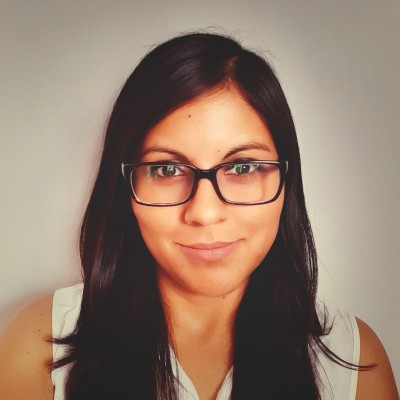
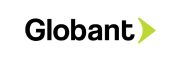