Interview questions to hire expert C# developers
C# is a powerful programming language widely used for building Windows applications, web applications, and games. When seeking to hire C# developers, it’s essential to assess their technical expertise, problem-solving skills, and experience with C# and the .NET framework.
Table of Contents
This comprehensive interview guide will assist you in identifying top C# talent and assembling a proficient development team.
1. Key Skills of C# Developers to Look Out For:
Before delving into the interview process, take note of the crucial skills to look for in C# developers:
- Proficiency in C#: Strong knowledge and experience in C# programming and object-oriented principles.
- .NET Framework Expertise: In-depth understanding of the .NET framework and its various components, such as ASP.NET and ADO.NET.
- Front-end Development: Familiarity with HTML, CSS, and JavaScript, as well as front-end frameworks like Angular or React.
- Database Management: Experience working with databases and SQL for data manipulation and retrieval.
- API Integration: Knowledge of working with APIs and integrating third-party services into applications.
2. An Overview of the C# Developer Hiring Process:
- Defining Job Requirements & Skills: Clearly outline the technical skills and experience you are seeking in a C# developer, including proficiency in C#, .NET framework, front-end technologies, and database management.
- Creating an Effective Job Description: Craft a comprehensive job description with a clear title, specific requirements, and details about the projects the developer will work on.
- Preparing Interview Questions: Develop a list of technical questions to assess candidates’ C# knowledge, problem-solving abilities, and experience in application development.
- Evaluating Candidates: Use a combination of technical assessments, coding challenges, and face-to-face interviews to evaluate potential candidates and determine the best fit for your organization.
3. Technical Interview Questions and Sample Answers:
Q1. What are access modifiers in C# and how are they used to control access to class members?
Access modifiers in C# determine the visibility and accessibility of class members. The common access modifiers are ‘public’, ‘private’, ‘protected’, and ‘internal’. ‘Public’ allows access from any class, ‘private’ restricts access to the same class, ‘protected’ enables access within the same class or derived classes, and ‘internal’ allows access within the same assembly.
Q2. Explain the concept of inheritance in C# and how it promotes code reusability.
Inheritance is a fundamental principle of object-oriented programming (OOP) in C#. It allows a class (derived class) to inherit properties and behavior from another class (base class). This promotes code reusability as the derived class can use the functionality of the base class without redefining it. For example:
// Base class public class Animal { public void Speak() { Console.WriteLine("Animal speaks."); } } // Derived class inheriting from Animal public class Dog : Animal { // Dog inherits the Speak() method from the Animal class. }
Q3. How do you handle exceptions in C# using try-catch blocks?
In C#, try-catch blocks are used to handle exceptions and prevent application crashes. The ‘try’ block contains the code that may throw an exception, and the ‘catch’ block catches the exception and handles it gracefully. For example:
try { // Code that may throw an exception } catch (Exception ex) { Console.WriteLine("An error occurred: " + ex.Message); }
Q4. How do you work with asynchronous programming in C# using async and await keywords?
The ‘async’ and ‘await’ keywords in C# are used to implement asynchronous programming, allowing non-blocking execution of tasks. The ‘async’ modifier is used on a method, and the ‘await’ keyword is used to pause execution until the awaited task completes. For example:
public async Task<string> FetchDataAsync() { // Asynchronous operation await Task.Delay(1000); return "Data fetched successfully."; }
Q5. Explain the purpose of LINQ (Language Integrated Query) in C#. How do you use it to query collections?
LINQ is a powerful feature in C# that provides a unified way to query and manipulate data from different data sources, including collections, databases, and XML documents. To use LINQ to query a collection, we can write queries using the ‘from’, ‘where’, ‘select’, and other keywords. For example:
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 }; var evenNumbers = from num in numbers where num % 2 == 0 select num; // 'evenNumbers' will contain [2, 4].
Q6. How do you implement multithreading in C#? What are the benefits and challenges of using multithreading?
Multithreading in C# is achieved using the ‘System.Threading’ namespace. By creating multiple threads, we can execute multiple tasks simultaneously, improving application performance. However, multithreading introduces challenges such as thread synchronization, potential race conditions, and deadlocks. Proper synchronization mechanisms like ‘lock’ and ‘Monitor’ are used to ensure thread safety.
Q7. How do you perform file I/O (Input/Output) operations in C#? Provide an example of reading data from a file.
File I/O operations in C# are handled using the ‘System.IO’ namespace. To read data from a file, we can use the ‘StreamReader’ class. For example:
string filePath = "data.txt"; using (StreamReader reader = new StreamReader(filePath)) { string data = reader.ReadToEnd(); Console.WriteLine(data); }
Q8. How do you implement dependency injection in C# to achieve loose coupling between classes?
Dependency Injection (DI) in C# is a design pattern used to achieve loose coupling between classes. Instead of creating dependencies inside a class, dependencies are injected from outside. This improves testability and maintainability. We can use constructor injection, property injection, or method injection to achieve DI.
Q9. Explain the concept of delegates and events in C#. How do they facilitate event-driven programming?
Delegates in C# are function pointers that allow methods to be assigned as parameters or return values. Events are a type of delegate that enables event-driven programming. An event in C# is a mechanism for a class to notify other classes when something happens. Event handlers are used to respond to events. For example:
public class Button { // Declare a delegate (if using non-generic pattern). public delegate void ClickEventHandler(object sender, EventArgs e); // Declare an event using the delegate. public event ClickEventHandler Click; // This method will raise the event. public void OnClick() { // Check if there are subscribers to the event. if (Click != null) { Click(this, EventArgs.Empty); } } }
4. The Importance of the Interview Process to Find the Right C# Talent:
The interview process is of paramount importance when hiring C# developers to ensure you select candidates with the necessary technical skills, problem-solving abilities, and experience in C# development. Conducting a rigorous interview helps in identifying developers who possess the expertise to build robust and scalable applications.
Moreover, the interview process enables you to evaluate candidates’ compatibility with your organization’s culture, work ethics, and collaborative capabilities. Finding developers who fit well within your team is crucial for fostering a positive and productive work environment.
5. How CloudDevs Can Help You Hire C# Developers:
Hiring C# developers can be a challenging task, especially when you have specific project requirements and tight deadlines. CloudDevs offers a platform that streamlines the hiring process by providing access to a curated pool of pre-screened senior C# developers.
With CloudDevs, you can:
- Connect with a dedicated consultant to discuss your project requirements and desired skill sets.
- Receive a shortlist of top C# developers within 24 hours, saving you time and effort in the initial screening process.
- Conduct interviews and assess candidates’ technical skills through coding challenges and face-to-face interviews.
- Start a no-risk trial with your selected C# developer to ensure the perfect fit for your projects.
By partnering with CloudDevs, you gain access to highly skilled C# developers who can deliver exceptional results and contribute to the success of your projects. Let CloudDevs handle the hiring process while you focus on building innovative applications with confidence.
Table of Contents
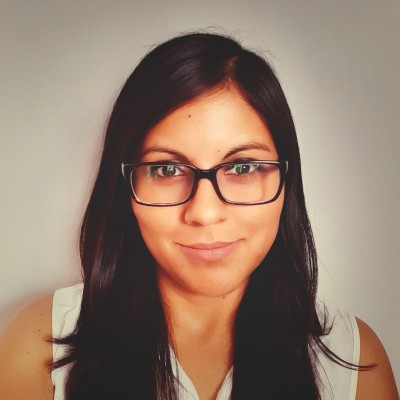
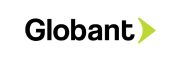