How to override a method in C#?
Method overriding is a fundamental concept in object-oriented programming (OOP) that allows a derived class (subclass) to provide a specific implementation of a method that is already defined in its base class (superclass). In C#, method overriding enables you to customize the behavior of inherited methods to suit the needs of the derived class, adhering to the “is-a” relationship between base and derived classes.
To override a method in C#, you need to follow these essential steps:
- Inheritance: Ensure that the derived class extends (inherits from) the base class. Method overriding is only possible within a class hierarchy.
- Base Class Method: Identify the method in the base class that you want to override. The method in the derived class must have the same name, return type, and parameter list as the one in the base class. The method in the base class should also be declared as `virtual`, `abstract`, or `override`.
- Override Keyword: In the derived class, use the `override` keyword before the method declaration. This keyword informs the compiler that you intend to provide a new implementation for the method.
- Implementation: Inside the overridden method in the derived class, provide the specific implementation that differs from the base class. You can access the base class implementation using the `base` keyword if needed.
Here’s a simplified example:
```csharp public class Animal { public virtual void MakeSound() { Console.WriteLine("Animal makes a sound"); } } public class Dog : Animal { public override void MakeSound() { Console.WriteLine("Dog barks"); } } ```
In this example, the `MakeSound` method in the `Dog` class overrides the method with the same name in the `Animal` base class. When you create an instance of `Dog` and call `MakeSound`, it will execute the custom implementation in the `Dog` class.
Method overriding in C# allows you to leverage polymorphism, enabling objects of the derived class to be treated as objects of the base class while using their specific implementations when necessary. It’s a powerful mechanism for achieving flexibility and extensibility in your object-oriented designs.
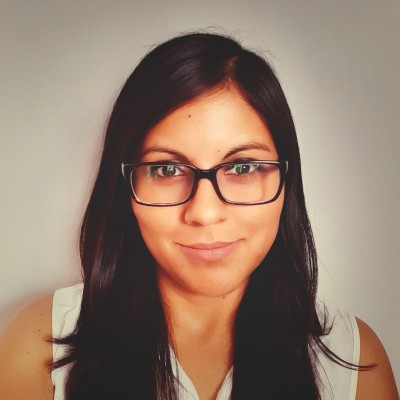
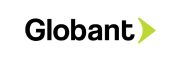