How to use middleware in ASP.NET Core?
Middleware in ASP.NET Core is a crucial component of the request pipeline that allows you to handle requests and responses in a flexible and modular way. Middleware components are essentially software components that process HTTP requests and responses as they flow through the ASP.NET Core pipeline. Each middleware component can perform a specific task, such as authentication, logging, routing, or serving static files.
Here’s how you can use middleware in ASP.NET Core:
- Built-in Middleware: ASP.NET Core provides a set of built-in middleware components that you can easily add to your application. For example, you can use the `UseAuthentication` middleware to enable authentication, or `UseRouting` to enable request routing. To use built-in middleware, you typically add them in the `Configure` method of the `Startup` class, within the `Startup.cs` file.
- Custom Middleware: You can also create your own custom middleware components to perform specific tasks that are not covered by the built-in middleware. Custom middleware is implemented as a C# class with a specific signature that accepts a `HttpContext` and a `Func<Task>` delegate. You can add your custom middleware to the pipeline using the `UseMiddleware` extension method.
- Ordering Middleware: The order in which middleware components are added to the pipeline matters. Middleware components are executed in the order they are added. For example, you’d typically add authentication and routing middleware before your custom middleware to ensure that authentication is processed before your custom logic.
- Middleware Pipeline: The middleware pipeline in ASP.NET Core is essentially a sequence of middleware components, where each component can process the incoming request, modify it, and pass it along to the next component. The final middleware component typically sends the response back to the client.
Here’s a simple example of adding middleware to handle CORS (Cross-Origin Resource Sharing) requests:
```csharp public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Home/Error"); app.UseHsts(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseCookiePolicy(); app.UseCors(builder => { builder.WithOrigins("https://example.com") .AllowAnyHeader() .AllowAnyMethod(); }); app.UseRouting(); // Add more middleware components as needed app.UseEndpoints(endpoints => { endpoints.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); }); } ```
In this example, we’ve added middleware to handle CORS requests, but you can add various other middleware components to cater to your application’s specific needs. Middleware in ASP.NET Core allows you to modularize your application’s behavior and make it more maintainable and extensible.
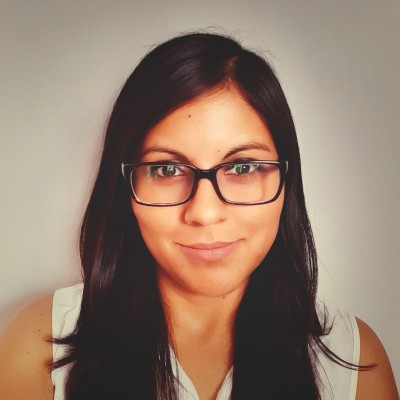
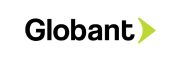