Exploring the C# Reflection API: Unveiling Hidden Powers
In the world of software development, flexibility and adaptability are paramount. As developers, we often encounter scenarios where we need to inspect and manipulate types dynamically at runtime. This is where the C# Reflection API comes to our rescue. Reflection provides a powerful set of tools that enable us to explore and interact with types, properties, methods, and other members of a C# assembly dynamically.
In this blog post, we will embark on a journey to unveil the hidden powers of the C# Reflection API. We will explore its features, learn how to leverage them effectively, and discover how Reflection can significantly enhance the flexibility and extensibility of our code.
What is Reflection?
Reflection is a powerful feature of C# that allows us to examine and manipulate the structure, behavior, and metadata of types at runtime. It provides a way to discover information about assemblies, types, and their members dynamically. With Reflection, we can inspect and modify properties, invoke methods, and even create objects dynamically.
Discovering Types with Reflection
1. Accessing Assembly Information:
At the core of the Reflection API lies the Assembly class, which represents an assembly in .NET. We can use the Assembly class to access information about loaded assemblies and perform various operations on them. Here’s an example of how to retrieve the assembly information:
csharp Assembly assembly = Assembly.GetExecutingAssembly(); Console.WriteLine($"Assembly Name: {assembly.FullName}"); Console.WriteLine($"Location: {assembly.Location}");
2. Exploring Types and Their Members:
Once we have an assembly, we can explore its types and their members using the Type class. The Type class provides a wealth of information about a type, such as its name, base type, implemented interfaces, properties, methods, fields, and more. Here’s an example of how to retrieve and explore the members of a type:
csharp Type type = typeof(MyClass); Console.WriteLine($"Type Name: {type.Name}"); Console.WriteLine("Properties:"); foreach (PropertyInfo property in type.GetProperties()) { Console.WriteLine(property.Name); } Console.WriteLine("Methods:"); foreach (MethodInfo method in type.GetMethods()) { Console.WriteLine(method.Name); }
Inspecting and Modifying Members
1. Retrieving Field and Property Values:
Reflection allows us to read and modify the values of fields and properties dynamically. We can use the FieldInfo and PropertyInfo classes to retrieve and manipulate the values of fields and properties, respectively. Here’s an example that demonstrates how to get and set property values dynamically:
csharp object instance = Activator.CreateInstance(typeof(MyClass)); PropertyInfo property = typeof(MyClass).GetProperty("MyProperty"); property.SetValue(instance, "New Value"); Console.WriteLine(property.GetValue(instance));
2. Invoking Methods Dynamically:
With Reflection, we can invoke methods dynamically by obtaining the MethodInfo and invoking it using the Invoke method. This allows us to execute methods on objects without having direct compile-time knowledge of their existence. Here’s an example:
csharp object instance = Activator.CreateInstance(typeof(MyClass)); MethodInfo method = typeof(MyClass).GetMethod("MyMethod"); method.Invoke(instance, null);
Dynamic Object Creation
1. Creating Instances of Types:
Reflection enables us to create instances of types dynamically, even if we don’t have compile-time knowledge of the types. We can use the Activator class to instantiate objects at runtime. Here’s an example:
csharp object instance = Activator.CreateInstance(typeof(MyClass));
2. Activating Objects with Activator:
The Activator class provides additional methods that allow us to create objects with constructor arguments or activate objects that are already instantiated but need initialization. Here’s an example:
csharp object instance = Activator.CreateInstance(typeof(MyClass), constructorArg1, constructorArg2);
Custom Attributes and Reflection
1. Understanding Attributes:
Attributes provide a way to associate metadata with types, members, and other program elements. Reflection enables us to discover and utilize custom attributes at runtime. Custom attributes can be used for a variety of purposes, such as adding additional behavior, specifying serialization settings, or defining security policies.
2. Reading and Using Custom Attributes:
We can use Reflection to read and interpret custom attributes applied to types and their members. By analyzing the attributes at runtime, we can make decisions or alter the behavior of our code dynamically. Here’s an example:
csharp Type type = typeof(MyClass); MyAttribute attribute = type.GetCustomAttribute<MyAttribute>(); if (attribute != null) { // Do something based on the attribute }
Performance Considerations
While Reflection provides powerful dynamic capabilities, it comes with a performance cost. The Reflection API is slower than direct, compile-time code due to the additional runtime analysis it performs. Therefore, it’s important to use Reflection judiciously and consider alternative solutions when performance is critical.
Conclusion
In this blog post, we delved into the world of C# Reflection and explored its hidden powers. We learned how to discover types, inspect and modify their members dynamically, create objects at runtime, and leverage custom attributes for runtime decisions. By harnessing the capabilities of the Reflection API, we can enhance the flexibility and extensibility of our code. However, it’s important to balance its usage with performance considerations. With a solid understanding of Reflection, we can unlock new possibilities and build more dynamic and adaptable software.
Table of Contents
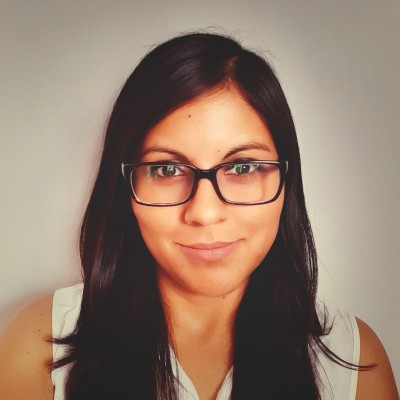
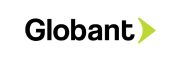