Secure Coding Practices in C#: Protecting Your Application
In today’s digital landscape, application security is of paramount importance. With the rise in cyberattacks and data breaches, developers must prioritize secure coding practices to safeguard their applications and protect sensitive user information. This blog will explore essential techniques and best practices for secure coding in C#, providing insights into common vulnerabilities and ways to mitigate them. By implementing these strategies, you can enhance the security of your C# applications and fortify them against potential threats.
Understanding the Importance of Secure Coding Practices
1. What is Secure Coding?
Secure coding refers to the practice of writing software with security considerations in mind. It involves following established guidelines, utilizing secure programming principles, and applying security best practices throughout the development lifecycle.
2. Benefits of Secure Coding
- Protection against Common Vulnerabilities: Secure coding practices help identify and mitigate common vulnerabilities, such as injection attacks, cross-site scripting (XSS), and insecure direct object references.
- Enhanced Trust and Reputation: By prioritizing security, developers build trust with users and stakeholders, which can lead to increased adoption and positive reputation.
- Cost-Effectiveness: Addressing security concerns during the development phase is more cost-effective than addressing them after an application has been deployed, saving both time and resources.
Common Vulnerabilities in C# Applications
1. Injection Attacks
Injection attacks, such as SQL injection and command injection, occur when untrusted data is improperly handled. Attackers can exploit these vulnerabilities to execute malicious code or gain unauthorized access to sensitive data.
Code Sample:
csharp // Example of vulnerable code susceptible to SQL injection string query = "SELECT * FROM Users WHERE Username = '" + userInput + "'"; SqlCommand command = new SqlCommand(query, connection);
To mitigate injection attacks, developers should adopt parameterized queries or use an ORM (Object-Relational Mapping) framework that automatically sanitizes user inputs.
2. Cross-Site Scripting (XSS)
XSS attacks involve injecting malicious scripts into a website or application, which are then executed by unsuspecting users’ browsers. This can lead to various security risks, including data theft, session hijacking, and defacement.
Code Sample:
csharp // Example of vulnerable code susceptible to XSS string userMessage = "<script>maliciousFunction();</script>"; Response.Write(userMessage);
To prevent XSS attacks, developers should employ input validation and output encoding techniques, such as HTML encoding or using secure templating engines.
3. Insecure Direct Object References
Insecure direct object references occur when developers expose internal implementation details, such as database keys or filenames, as direct references in URLs or parameters. Attackers can manipulate these references to access unauthorized resources or escalate privileges.
Code Sample:
// Example of vulnerable code with insecure direct object reference int userId = Convert.ToInt32(Request.QueryString["userId"]); User user = dbContext.Users.Find(userId);
To mitigate insecure direct object references, developers should use indirect references, such as a randomly generated token or a mapping table, to access sensitive resources.
Best Practices for Secure Coding in C#
1. Input Validation
Implement robust input validation by enforcing strict data type checks, length restrictions, and using whitelist-based validation. Sanitize and validate all user inputs to prevent injection attacks and ensure data integrity.
Code Sample:
csharp // Example of input validation using regular expressions if (Regex.IsMatch(input, @"^\d{4}-\d{2}-\d{2}$")) { // Valid date format // Process the input }
2. Output Encoding
Always encode user-supplied data before displaying it in HTML, URLs, or other contexts. This prevents malicious code execution and ensures the integrity of the displayed content.
Code Sample:
csharp // Example of output encoding using HTML encoding string userInput = "<script>alert('XSS attack!');</script>"; string encodedInput = HttpUtility.HtmlEncode(userInput); Response.Write(encodedInput);
3. Authentication and Authorization
Implement secure authentication mechanisms, such as strong password hashing, multi-factor authentication, and session management. Apply the principle of least privilege and perform authorization checks to restrict access to sensitive functionality and resources.
Code Sample:
csharp // Example of password hashing using bcrypt string hashedPassword = BCrypt.Net.BCrypt.HashPassword(plainPassword);
4. Secure Configuration Management
Avoid hardcoding sensitive information, such as passwords or API keys, in your code. Instead, store them securely in configuration files or use secure storage mechanisms like Azure Key Vault. Regularly review and rotate secrets to minimize the impact of a potential compromise.
Code Sample:
csharp // Example of accessing secrets from Azure Key Vault var secretValue = await new SecretClient(vaultUri, credential).GetSecretAsync("MySecret"); string secret = secretValue.Value.Value;
Conclusion
By implementing secure coding practices in C#, developers can significantly enhance the security of their applications. Through input validation, output encoding, authentication and authorization, and secure configuration management, vulnerabilities like injection attacks, XSS, and insecure direct object references can be mitigated effectively. Remember, application security is an ongoing process, and staying up to date with emerging threats and best practices is crucial to protecting your application and the data it handles. Prioritize security from the start, and your C# applications will be better equipped to withstand potential attacks in today’s evolving threat landscape.
Table of Contents
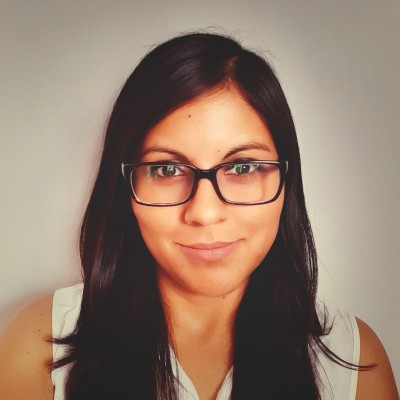
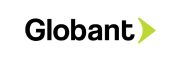