How to serialize and deserialize JSON in C#?
Serializing and deserializing JSON in C# is a common task, often required when working with web APIs, configuration files, or data exchange between different systems. You can achieve this using libraries like Newtonsoft.Json (JSON.NET) or the built-in System.Text.Json in .NET Core and .NET 5 onwards. Here’s a step-by-step guide on how to serialize and deserialize JSON in C#:
Serialization (Object to JSON):
- Add JSON Serialization Library: If you’re not already using one, install a JSON serialization library like Newtonsoft.Json (available via NuGet) or use the built-in System.Text.Json.
- Create an Object: Define a C# object or use an existing one that you want to serialize to JSON. Ensure that the object’s properties match the structure of the JSON data you want to produce.
- Serialization: Use the JSON serialization library to convert the object into a JSON-formatted string. Here’s a basic example using Newtonsoft.Json:
```csharp using Newtonsoft.Json; // Create an object var myObject = new MyObject { Name = "John", Age = 30 }; // Serialize the object to JSON string json = JsonConvert.SerializeObject(myObject); ```
- JSON Output: The variable `json` now holds the JSON representation of your C# object.
Deserialization (JSON to Object):
- JSON Input: Have a valid JSON string that you want to deserialize into a C# object. This JSON string should match the structure of the C# object you’re expecting.
- Deserialization: Use the JSON serialization library to deserialize the JSON string into a C# object. In the case of Newtonsoft.Json:
```csharp using Newtonsoft.Json; // JSON input string json = "{\"Name\":\"Alice\",\"Age\":25}"; // Deserialize JSON to object MyObject myObject = JsonConvert.DeserializeObject<MyObject>(json); ```
- Object Usage: You can now work with the `myObject` as a fully populated C# object.
Remember to handle exceptions and validation as needed during the deserialization process to ensure that the JSON data aligns with the expected object structure. Proper error handling helps prevent runtime issues when dealing with JSON data from external sources.
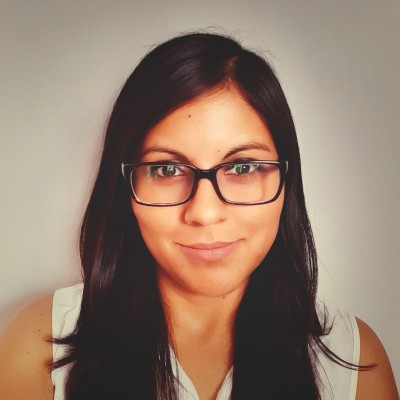
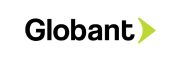