How to use transactions in Entity Framework Core?
In Entity Framework Core (EF Core), transactions are a fundamental concept for managing database operations as a single, atomic unit of work. They ensure that a series of database operations either succeed entirely or fail entirely, maintaining data integrity and consistency. Here’s a step-by-step guide on how to use transactions in EF Core:
- Create a Transaction Scope: To begin a transaction in EF Core, you first create a transaction scope. This scope defines the boundaries within which database operations are treated as a single transaction. You can use the `BeginTransaction` method provided by the `DbContext` class:
```csharp using var dbContext = new YourDbContext(); using var transaction = dbContext.Database.BeginTransaction(); ```
- Perform Database Operations: Once you have a transaction scope, you can execute various database operations within it. These operations can include inserts, updates, deletes, or any other operations supported by EF Core. All the changes made within the transaction scope will be considered part of the same transaction.
```csharp dbContext.Add(entity1); dbContext.Update(entity2); dbContext.Remove(entity3); ```
- Commit or Rollback: After executing the database operations, you have two options:
– Commit: If all operations within the transaction are successful and you want to make the changes permanent in the database, you can call `transaction.Commit()`. This action finalizes the transaction, and all changes are saved to the database.
– Rollback: If an error occurs during any of the operations or you decide to discard the changes, you can call `transaction.Rollback()`. This action undoes all the changes made within the transaction, ensuring that none of the modifications are persisted to the database.
Here’s a sample code snippet demonstrating the usage of transactions in EF Core:
```csharp using var dbContext = new YourDbContext(); using var transaction = dbContext.Database.BeginTransaction(); try { // Perform database operations within the transaction dbContext.Add(entity1); dbContext.Update(entity2); // Commit the transaction to save changes to the database transaction.Commit(); } catch (Exception) { // Handle exceptions and roll back the transaction if necessary transaction.Rollback(); } ```
Transactions in EF Core are essential for maintaining data consistency, especially in scenarios where multiple related database operations need to be coordinated. They ensure that either all changes are applied successfully, or none of them are, preventing data corruption or incomplete updates.
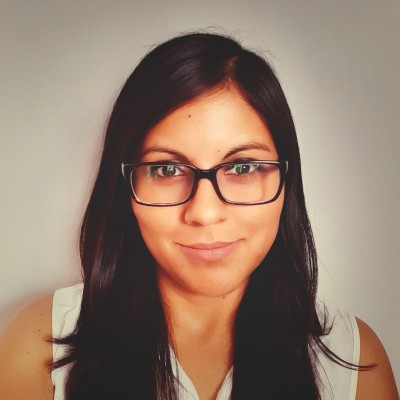
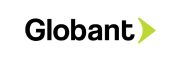