How to use LINQ in C#?
LINQ (Language Integrated Query) is a powerful feature in C# that allows you to query and manipulate data in a declarative and readable manner. LINQ provides a uniform way to query different data sources such as arrays, collections, databases, XML, and more. Here are the key steps to use LINQ in C#:
- Import LINQ Namespace:
To use LINQ, ensure that you import the `System.Linq` namespace in your code file by adding `using System.Linq;` at the beginning.
- Create a Data Source:
Start by having a data source, such as an array, a `List`, a database, or any enumerable collection, from which you want to retrieve or manipulate data.
- Write LINQ Query Expressions:
LINQ queries are written using query expression syntax or method syntax. Query expression syntax is similar to SQL, making it easy to read and understand. Here’s an example using query expression syntax to filter even numbers from an array:
```csharp int[] numbers = { 1, 2, 3, 4, 5, 6 }; var evenNumbers = from num in numbers where num % 2 == 0 select num; ```
- Use LINQ Extension Methods:
Alternatively, you can use LINQ extension methods provided by `System.Linq` for method syntax. The previous query can be rewritten as follows:
```csharp var evenNumbers = numbers.Where(num => num % 2 == 0); ```
- Execute the Query:
LINQ queries are not executed immediately; they are deferred until you enumerate the results. You can enumerate the results using a `foreach` loop, `ToList()`, `ToArray()`, or other methods to trigger execution.
- Handle the Results:
Once the query is executed, you can iterate through the results and perform actions as needed.
LINQ simplifies data querying and manipulation in C#, providing a consistent and concise way to work with various data sources. It offers a wide range of operators like `Where`, `Select`, `OrderBy`, `GroupBy`, and more, making it a valuable tool for developers dealing with data-related tasks. Whether you’re working with collections, databases, or XML, LINQ can streamline your code and improve readability.
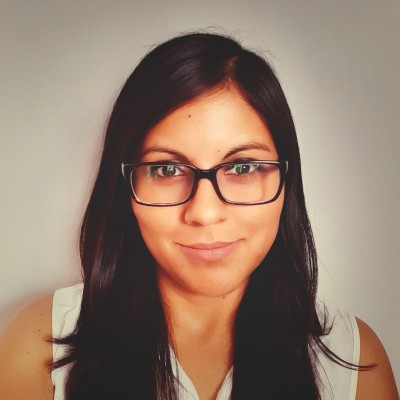
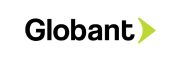