CodeIgniter Q & A
How to use CodeIgniter’s built-in form helper functions?
CodeIgniter’s built-in form helper functions provide a convenient way to create and manage HTML forms in your web applications. These helpers simplify the process of generating form elements, handling form submissions, and performing validation. Here’s a guide on how to use CodeIgniter’s form helper functions effectively:
- Loading the Form Helper: Before you can use the form helper functions, you need to load the helper. In your controller’s constructor or method, use the following code:
```php $this->load->helper('form'); ```
- Creating Form Open and Close Tags: To start and end your HTML form, use the `form_open()` and `form_close()` functions. These functions automatically generate the `<form>` tags with the necessary attributes:
```php echo form_open('controller/method'); // Replace 'controller/method' with the actual URL where the form should be submitted. // Form elements go here echo form_close(); ```
- Adding Form Elements: Use functions like `form_input()`, `form_textarea()`, and `form_dropdown()` to create various form elements like text inputs, textareas, and dropdown menus. These functions allow you to specify attributes and default values easily:
```php echo form_input('username', '', 'placeholder="Username"'); echo form_password('password', '', 'placeholder="Password"'); echo form_textarea('comments', '', 'placeholder="Comments"'); echo form_dropdown('gender', $options, 'male'); ```
- Handling Form Submission: In your controller, check for form submission using the `$_POST` or `$_GET` variables. You can use CodeIgniter’s form validation library in combination with the form helper to validate and process form data. For example:
```php $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required'); if ($this->form_validation->run() === true) { // Form data is valid; process it here } else { // Form data is invalid; display the form with error messages $this->load->view('your_form_view'); } ```
- Displaying Form Errors: CodeIgniter’s form validation library also helps you display error messages alongside form elements. Use `form_error()` to display validation errors next to the corresponding fields in your view:
```php echo form_input('username', set_value('username'), 'placeholder="Username"'); echo form_error('username'); ```
- Security: CodeIgniter’s form helper functions automatically generate CSRF tokens, which protect your forms against cross-site request forgery (CSRF) attacks. There’s no need to manually handle CSRF tokens when using these functions.
By leveraging CodeIgniter’s form helper functions, you can streamline the process of creating, processing, and validating forms in your web applications, making it easier to build interactive and secure user interfaces.
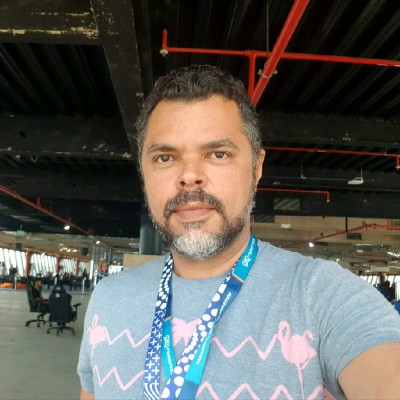
Previously at
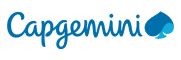
Experienced Full Stack Systems Analyst, Proficient in CodeIgniter with extensive 5+ years experience. Strong in SQL, Git, Agile.