CodeIgniter Caching: A Game Changer for Web Application Performance
One of the most powerful yet underutilized aspects of modern web application development is caching. In an era where a fraction of a second delay can turn users away, speed and performance have become fundamental. CodeIgniter, a robust PHP framework, has comprehensive caching mechanisms that, when properly used, can significantly enhance the performance and speed of your applications. This blog will explore the CodeIgniter caching system, its types, and will illustrate their use through practical examples.
What is Caching?
In the realm of computing, caching refers to the practice of storing copies of data in caches – fast storage components – so future requests for that data can be served quickly. The data stored in a cache might result from an earlier computation or a copy of data stored elsewhere. When a web application needs to access data, it first checks the cache. If the data exists, this is a ‘cache hit.’ If not, it’s a ‘cache miss,’ and the data needs to be fetched from its primary storage area, which is often slower.
Understanding CodeIgniter Caching
CodeIgniter supports several types of caching:
- Page Caching: This technique involves storing the full content of a dynamically generated page for quicker retrieval in subsequent requests. Page caching can drastically reduce server load by avoiding database hits.
- Database Query Caching: This method stores the results of a database query. When the same query is made, it retrieves the cached result instead of running the query again.
- Driver-based Caching: CodeIgniter has a caching class that allows storing data in different cache storages like APC, Memcached, Redis, etc.
Let’s delve deeper into each of these caching types with some practical examples.
Page Caching in CodeIgniter
In CodeIgniter, page caching is straightforward. When a page is loaded for the first time, the cache file is written to your application/cache folder, and for subsequent page loads, this file is served instead of the original file.
Example
```php $this->output->cache(n); ``` In this code, `n` is the number of minutes you wish the page to remain cached between refreshes. Let's look at a more concrete example: ```php public function index() { $this->output->cache(60); // Cache for 60 minutes $this->load->view('my_view'); } ```
In the above code, ‘my_view’ will remain cached for 60 minutes. If you want to clear the cache before the 60 minutes, you must manually delete the cache files.
Database Query Caching in CodeIgniter
Query caching can save a lot of time for those database queries that are run repeatedly but do not change often.
Example
```php $this->db->cache_on(); $query = $this->db->query("SELECT * FROM my_table"); ```
In the code snippet above, caching is turned on with the `$this->db->cache_on();` method. The query results are then stored in the cache directory. For subsequent executions of the query, CodeIgniter uses the cached result instead of running the query again.
If you want to turn off the caching, you can do so with `$this->db->cache_off();`.
Example
```php $this->db->cache_delete('controller', 'method'); ```
The `cache_delete()` method is used to delete cached files for a specific page. In the above code, replace ‘controller’ and ‘method’ with the respective controller name and method name.
Driver-based Caching in CodeIgniter
CodeIgniter’s Cache driver provides a consistent interface to work with different types of cache, including file-based caching, Memcached, and APC.
To use the Cache driver, you need to initialize it. You can autoload it by adding it to your `autoload.php` file, or you can manually load it using `$this->load->driver(‘cache’);`.
Example
```php // Save data to cache if ( ! $foo = $this->cache->get('foo')) { echo 'Saving to the cache!<br />'; $foo = 'foobarbaz!'; // Save into the cache for 5 minutes $this->cache->save('foo', $foo, 300); } echo $foo; ```
In this example, we attempt to get data from the cache. If it does not exist (`$this->cache->get(‘foo’)`), we echo a message, assign a value to `$foo`, and save that value into the cache (`$this->cache->save(‘foo’, $foo, 300)`). The last parameter in the `save()` function is the time-to-live, or how long the data should remain in the cache in seconds. If the data is in the cache, we simply output it.
To delete cache data, use the `delete()` function:
```php $this->cache->delete('foo'); ```
In this code, the cached data under the key ‘foo’ is deleted.
Wrapping Up
While caching is a powerful tool, it is essential to use it judiciously. Caching should be implemented for parts of your application that require significant processing time or handle high-traffic.
When implemented properly, CodeIgniter’s caching features can boost the performance of your application, providing a faster, smoother experience to your users. It’s important to remember that different applications have different requirements – there is no one-size-fits-all solution. Therefore, experiment with different caching strategies and select the ones that fit your application’s needs.
Remember, a well-performing application not only leads to happier users but also to higher SEO rankings, leading to increased visibility and growth. So, harness the power of caching in CodeIgniter and take your applications to the next level!
Table of Contents
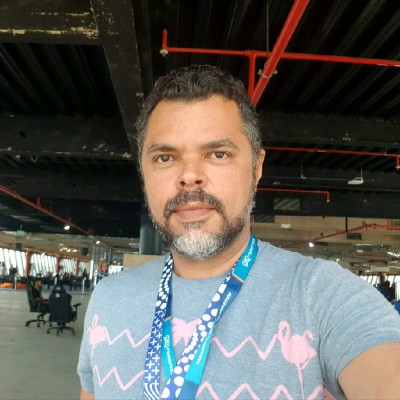
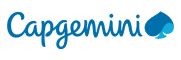