CRUD Operations in CodeIgniter: An Easy Path to Your Own Blog
CodeIgniter is a PHP MVC (Model-View-Controller) framework that simplifies web application development. It’s known for its speed, flexibility, and ease of use. One of the fundamental aspects of any web application is CRUD operations – the ability to Create, Read, Update, and Delete data. In this blog post, we’ll guide you through building a simple blog application using CodeIgniter and perform CRUD operations.
Setting Up CodeIgniter
Before starting, ensure you have a local development environment with PHP, MySQL, and Composer installed.
Download CodeIgniter from the official website or via Composer:
``` composer create-project codeigniter4/appstarter blog ```
After downloading, navigate to the project folder (i.e., ‘blog’) and run the built-in development server:
``` php spark serve ```
The application should be running on http://localhost:8080.
Creating the Database and Configuration
For our blog, we’ll create a simple MySQL database named ‘blog_db’. In it, we’ll have a table ‘posts’ with the following fields: id, title, body, and created_at.
```sql CREATE DATABASE blog_db; USE blog_db; CREATE TABLE posts ( id int(11) NOT NULL AUTO_INCREMENT, title varchar(255) NOT NULL, body text NOT NULL, created_at datetime NOT NULL DEFAULT current_timestamp(), PRIMARY KEY (id) ); ```
Now, let’s configure CodeIgniter to use this database. Go to ‘app/Config/Database.php’ and add your database details:
```php public $default = [ 'DSN' => '', 'hostname' => 'localhost', 'username' => 'root', 'password' => '', 'database' => 'blog_db', 'DBDriver' => 'MySQLi', ... ]; ```
Setting Up MVC Structure
- Model: Let’s create a model for our blog posts. Under the ‘app/Models’ directory, create a new file ‘PostModel.php’:
```php namespace App\Models; use CodeIgniter\Model; class PostModel extends Model { protected $table = 'posts'; protected $primaryKey = 'id'; protected $allowedFields = ['title', 'body']; } ```
- View: Under ‘app/Views’, create a directory ‘posts’ and inside it create ‘index.php’. This will be the landing page of our blog:
```html <h2>Blog Posts</h2> <!-- We will dynamically generate blog posts here --> ```
- Controller: Under ‘app/Controllers’, create ‘PostController.php’:
```php namespace App\Controllers; use App\Models\PostModel; class PostController extends BaseController { public function index() { $model = new PostModel(); $data['posts'] = $model->findAll(); return view('posts/index', $data); } } ```
You can now visit http://localhost:8080/posts to see the index page.
CRUD Operations
- Create: In the ‘PostController’, add a ‘create’ method:
```php public function create() { return view('posts/create'); } ```
And create a ‘create.php’ view in ‘app/Views/posts’:
```html <h2>Create a New Post</h2> <form method="post" action="/posts/store"> <!-- We will add form fields here --> </form> ```
In the ‘PostController’, add a ‘store’ method to save the post:
```php public function store() { $model = new PostModel(); $data = [ 'title' => $this->request->getPost('title'), 'body' => $this->request->getPost('body'), ]; $model->insert($data); return redirect()->to('/posts'); } ```
- Read: We have already implemented the read operation in our ‘index’ method, where we fetch all posts.
- Update: In the ‘PostController’, add an ‘edit’ method:
```php public function edit($id = null) { $model = new PostModel(); $data['post'] = $model->find($id); return view('posts/edit', $data); } ```
Create an ‘edit.php’ view in ‘app/Views/posts’ similar to ‘create.php’ but pre-filled with existing data. Add an ‘update’ method in the ‘PostController’ to save changes:
```php public function update($id = null) { $model = new PostModel(); $data = [ 'title' => $this->request->getPost('title'), 'body' => $this->request->getPost('body'), ]; $model->update($id, $data); return redirect()->to('/posts'); } ```
- Delete: In the ‘PostController’, add a ‘delete’ method:
```php public function delete($id = null) { $model = new PostModel(); $model->delete($id); return redirect()->to('/posts'); } ```
Remember to secure these operations according to your needs and handle potential errors.
Conclusion
By now, you should have a simple blog running on your local server, complete with CRUD operations. CodeIgniter’s straightforward approach helps developers create dynamic web applications with less complexity.
This tutorial provides you a baseline. You can extend this blog by adding user authentication, comments, tags, and many more features. Keep exploring and Happy Coding!
Table of Contents
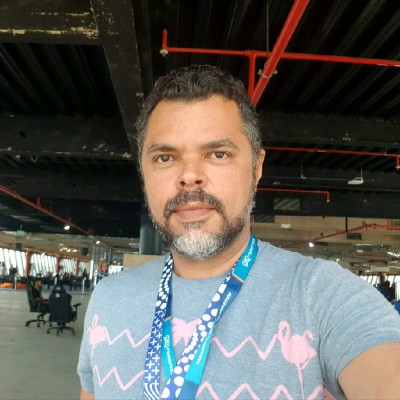
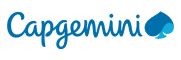