Unlocking Database Potential with CodeIgniter: A Comprehensive Guide to MySQL Connectivity and Beyond
CodeIgniter, a powerful PHP framework, has been widely acclaimed for its speed, small footprint, flexibility, and security. When you hire CodeIgniter developers, their expertise can be a game-changer, transforming your project into a robust application. At the heart of any web application, a database plays an integral role, and CodeIgniter, when managed effectively by professional CodeIgniter developers, makes database management efficient, easy, and effective.
Today, let’s delve deep into the world of CodeIgniter database connectivity, as used by top CodeIgniter developers, focusing on MySQL, and explore other database systems CodeIgniter supports.
Overview of CodeIgniter’s Database Library
CodeIgniter’s Database Library provides a unified interface to interact with various types of database systems. It supports MySQL, MySQLi, PostgreSQL, ODBC, SQLite, and more. CodeIgniter’s database abstraction layer allows you to use various database functions irrespective of the underlying database type, providing seamless operation and compatibility.
Connecting to a Database
Before you can interact with any database, the first step is to establish a connection. In CodeIgniter, you configure database settings in the database configuration file found at application/config/database.php.
A simple MySQL database configuration might look like this:
```php $db['default'] = array( 'dsn' => '', 'hostname' => 'localhost', 'username' => 'root', 'password' => '', 'database' => 'test_db', 'dbdriver' => 'mysqli', 'dbprefix' => '', 'pconnect' => FALSE, 'db_debug' => TRUE, 'cache_on' => FALSE, 'cachedir' => '', 'char_set' => 'utf8', 'dbcollat' => 'utf8_general_ci', 'swap_pre' => '', 'encrypt' => FALSE, 'compress' => FALSE, 'stricton' => FALSE, 'failover' => array(), 'save_queries' => TRUE );
In this example, ‘hostname’ is your server (usually localhost), ‘username’ and ‘password’ are your database credentials, ‘database’ is the name of your database, and ‘dbdriver’ is the database type you are using. Set these according to your database credentials, and CodeIgniter will take care of the rest.
Database Querying
Once you’ve established a connection, you can use CodeIgniter’s database library to create, read, update, and delete records.
For instance, to fetch all records from a table named ‘users‘, you could do:
```php $this->load->database(); // load database library $query = $this->db->get('users'); // perform the query $result = $query->result(); // get the result
Here, `$query->result()` returns an array of objects, each representing a record (row) in the ‘users’ table.
To insert a new record, you might do:
```php $data = array( 'username' => 'johndoe', 'email' => 'johndoe@example.com' ); $this->db->insert('users', $data);
In this example, an array is created with keys representing the table’s column names and values representing the data to insert. The insert function adds the record to the database.
Updating a record works similarly:
```php $data = array( 'email' => 'newemail@example.com' ); $this->db->where('username', 'johndoe'); $this->db->update('users', $data);
Here, ‘where’ method is used to specify the record to update, and the ‘update’ method updates the record.
Deleting a record is straightforward:
```php $this->db->where('username', 'johndoe '); $this->db->delete('users');
This code would delete the user ‘johndoe’ from the ‘users’ table.
Working with Different Database Types
One of the major strengths of CodeIgniter’s database library, which is frequently leveraged by those who hire CodeIgniter developers, is its ability to work with multiple types of databases using the same functions. If you’re connecting to a PostgreSQL database, for example, you just need to change the ‘dbdriver’ in your database configuration to ‘postgre’ and possibly adjust the hostname, username, password, and database settings accordingly. This is a key reason why businesses opt to hire CodeIgniter developers – their familiarity with such diverse database operations streamlines development processes.
Query Builder Class
To simplify database interactions and ensure compatibility across different database types, CodeIgniter provides the Query Builder class. It enables you to build queries using functions, which handle the nuances of different SQL dialects for you.
A basic select operation using Query Builder could look like this:
```php $this->db->select('*'); $this->db->from('users'); $query = $this->db->get();
This code fetches all columns (as denoted by ‘*’) from the ‘users’ table.
Active Record and Beyond
CodeIgniter’s Active Record class provides an interface to build and execute database queries. It’s not a full-featured ORM (Object-Relational Mapping) solution, but it offers a more straightforward and less error-prone way of working with your database.
Beyond the Active Record class, CodeIgniter also supports more advanced database operations, like transactions, custom SQL queries, and advanced where clauses. You can even extend the database class to add custom functions.
Conclusion
CodeIgniter’s database library is a powerful tool that allows developers to interact with a variety of database types in a straightforward, unified manner. It simplifies database operations, allowing you to focus more on your application logic rather than worrying about database compatibility or SQL syntax differences.
From connecting to your database to executing complex queries, CodeIgniter’s database library, a tool that skilled CodeIgniter developers use to their advantage, along with features like the Query Builder class and Active Record, makes working with databases in PHP a much more manageable task.
When you hire CodeIgniter developers, you leverage their hands-on experience and deep understanding of these complex tasks, saving you the time and effort of mastering these intricacies on your own.
Remember, the key to mastering CodeIgniter’s database library is practice. However, if you want to expedite your project, you might consider the option to hire CodeIgniter developers. They come with the skill and knowledge to unlock the power of CodeIgniter’s database connectivity right from the get-go!
Table of Contents
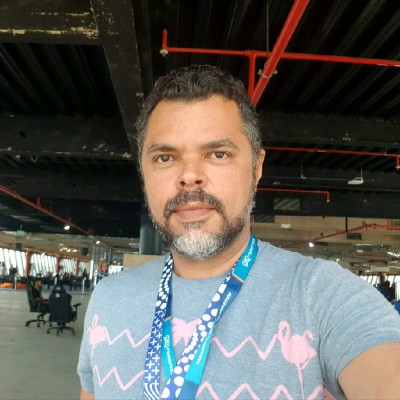
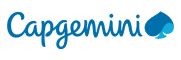