CodeIgniter Q & A
How to perform database operations using CodeIgniter’s active record?
Performing database operations using CodeIgniter’s Active Record is a straightforward and efficient process that simplifies database interactions. Active Record provides an object-oriented approach to working with databases, making it easy to create, retrieve, update, and delete records. Here are the fundamental steps for performing database operations using CodeIgniter’s Active Record:
- Load the Database Library: Start by loading the CodeIgniter database library in your controller or model. You can do this using the `$this->load->database()` method, typically in your controller’s constructor.
- Create an Active Record Instance: To work with a specific database table, create an Active Record instance associated with that table. You can do this by calling `$this->db->get(‘table_name’)`, where `’table_name’` is the name of the database table you want to interact with.
- Retrieve Data (SELECT): To retrieve data from the table, you can use various methods like `get()`, `get_where()`, and `select()`. For example, `$this->db->get(‘table_name’)` fetches all records from the table.
- Insert Data (INSERT): To insert data into the table, use the `insert()` method. Provide an associative array with the data you want to insert as a parameter. For example, `$data = array(‘column1’ => ‘value1’, ‘column2’ => ‘value2’); $this->db->insert(‘table_name’, $data);`
- Update Data (UPDATE): To update existing records, use the `update()` method. Specify the table name, data to update, and a `where` condition to identify the records to be updated. For example, `$this->db->update(‘table_name’, $data, array(‘id’ => $record_id));`
- Delete Data (DELETE): To delete records, use the `delete()` method. Specify the table name and a `where` condition to identify the records to be deleted. For example, `$this->db->delete(‘table_name’, array(‘id’ => $record_id));`
- Method Chaining: Active Record supports method chaining, allowing you to combine multiple operations in a single query for more complex scenarios.
By following these steps and using CodeIgniter’s Active Record methods, you can perform a wide range of database operations efficiently and with a clear, readable syntax. Active Record abstracts the underlying SQL queries, making database interactions in your CodeIgniter application more organized and manageable.
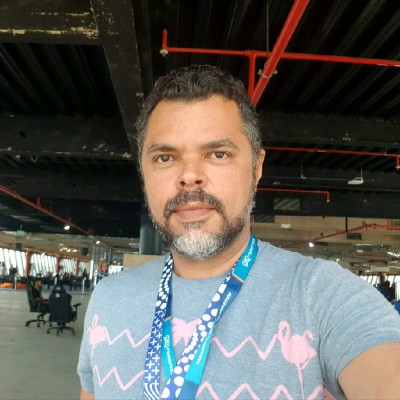
Previously at
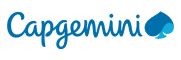
Experienced Full Stack Systems Analyst, Proficient in CodeIgniter with extensive 5+ years experience. Strong in SQL, Git, Agile.