Enhancing Security in CodeIgniter Applications: Essential Best Practices
CodeIgniter, a highly regarded open-source PHP framework, assists developers worldwide in creating robust and scalable web applications. Its simplicity and flexibility make it an attractive choice for many businesses looking to hire CodeIgniter developers. However, as with any web development framework, security is an aspect that must never be overlooked.
Given the paramount importance of application security, many businesses choose to hire CodeIgniter developers who understand not just how to build applications, but also how to secure them. In this blog post, we will delve into several best practices for securing your CodeIgniter applications. These strategies are routinely used by experienced CodeIgniter developers to ensure the applications they develop are as secure as they are functional.
In this blog post, we’ll explore several best practices for securing your CodeIgniter applications, accompanied by real-world examples.
1. Escaping Queries
Escaping queries is a fundamental step in securing your CodeIgniter application against SQL Injection attacks. CodeIgniter provides an inbuilt method for this:
```php $sql = "SELECT * FROM users WHERE username = ? AND password = ?"; $this->db->query($sql, array($username, $password));
In the above example, the ‘?’ symbol is replaced by the user’s input, and CodeIgniter automatically escapes it.
2. Cross-Site Request Forgery (CSRF) Protection
CSRF is a type of attack that tricks an unsuspecting user into executing actions on your web application without their consent. CodeIgniter offers in-built CSRF protection. You can enable it by setting `$config[‘csrf_protection’] = TRUE;` in your `config.php` file.
Then, when creating forms, use the `form_open()` helper function which automatically inserts a CSRF token:
```php echo form_open('user/login');
3. Cross-Site Scripting (XSS) Protection
XSS attacks involve injecting malicious scripts into web pages viewed by users. CodeIgniter provides an XSS filtering function that can prevent such attacks. For instance, you can activate global XSS filtering via your `config.php` file:
```php $config['global_xss_filtering'] = TRUE;
Alternatively, you can use the `xss_clean()` method to filter individual data:
```php $post = $this->security->xss_clean($this->input->post());
4. Input Data Validation
Ensure all data received from users is valid and safe. Use the CodeIgniter’s Form Validation Class to enforce rules for your form data:
```php $this->form_validation->set_rules('username', 'Username', 'required|alpha_numeric');
The above example enforces that the username should be alphanumeric and not empty.
5. Secure Session Handling
Sessions can be hijacked, allowing unauthorized access to your application. To enhance session security, CodeIgniter allows you to use database sessions, rather than default file-based sessions. Set `$config[‘sess_driver’] = ‘database’;` in your `config.php` file.
You can also enable session data encryption by setting `$config[‘sess_encrypt_cookie’] = TRUE;`.
6. Secure Password Handling
Always hash passwords, rather than storing them in plaintext. CodeIgniter’s Security Class provides a useful hashing function:
```php $hashedPassword = password_hash($password, PASSWORD_DEFAULT);
To verify a password, use `password_verify()`, like so:
```php if (password_verify($password, $hashedPassword)) { // Password is valid } else { // Invalid password }
7. Hide Sensitive Information
Refrain from revealing sensitive information such as database details, application keys, or error details to users. In your `config.php` file, ensure you’ve set `$config[‘index_page’] = ”`; to hide the `index.php` from the URL.
In the `database.php` configuration file, ensure you don’t hard code your database credentials. Instead, use environment variables or a configuration file outside your document root.
8. Keep your CodeIgniter Updated
Updating your CodeIgniter framework ensures you have the latest security patches and features. Make it a habit to keep your application up-to-date with the latest version of the framework.
9. Use HTTPS
Using HTTPS encrypts the data transmitted between the client and server, adding an extra layer of security. You can enforce HTTPS in CodeIgniter by using `redirect()` function:
```php if (!isset($_SERVER['HTTPS']) || $_SERVER['HTTPS'] != "on") { redirect(base_url(uri_string())); exit; }
10. Limit Rate of Requests
To protect your application against brute-force attacks, limit the rate of requests. CodeIgniter doesn’t provide this feature by default, but you can use third-party libraries like “CodeIgniter-RateLimiter”.
Conclusion
Securing your web application is a continuous process, one that requires a consistent and diligent approach. The tips we’ve explored today will undoubtedly assist in making your CodeIgniter application more secure, but these alone are only part of a comprehensive security strategy.
This underscores the importance of why many organizations choose to hire CodeIgniter developers. Expert developers bring an in-depth understanding of the framework, are well-versed in the latest security best practices, and can apply these methodologies accordingly to your application.
Remember, a secure application not only safeguards your data but also fosters trust with your users. When users know that their data is secure, they’re more likely to continue using your services. Hence, to ensure that your CodeIgniter application is secure from the get-go, consider hiring experienced CodeIgniter developers who can employ these best practices and more.
In conclusion, security is not a destination but a journey that requires ongoing vigilance and updates. Always stay updated with the latest security best practices, which can often be achieved most effectively when you hire CodeIgniter developers dedicated to ensuring the robustness and security of your application.
Table of Contents
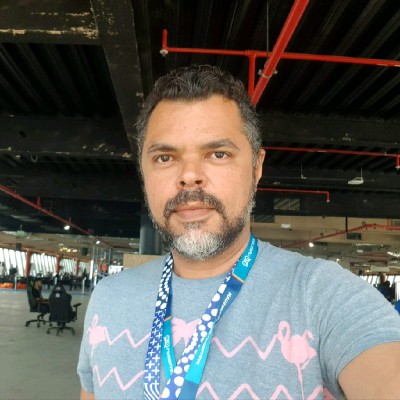
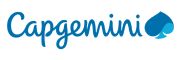