Ace Your Coding with CodeIgniter: Master Error Handling & Debugging
In the realm of PHP development, CodeIgniter is a renowned open-source framework. It provides developers with tools and libraries to build robust web applications swiftly and with ease. However, during the course of development, encountering errors and exceptions is quite common, but luckily, CodeIgniter has a well-organized error handling mechanism.
This article aims to highlight different ways to handle errors in CodeIgniter, provide some useful debugging techniques, and guide you in troubleshooting common issues. Let’s start by understanding how to display errors in CodeIgniter.
Displaying Errors in CodeIgniter
In CodeIgniter, error reporting is managed by a simple function `error_reporting()` in the index.php file. By default, all PHP errors are shown except notices and deprecated messages. This setting is ideal during development.
```php error_reporting(E_ALL & ~E_NOTICE & ~E_DEPRECATED); ```
If you want to see all errors including notices, you can modify the above line as follows:
```php error_reporting(E_ALL); ```
In a production environment, it is better to disable error reporting or limit it to critical errors only to avoid exposing sensitive information to the users.
```php error_reporting(E_ERROR | E_PARSE); ```
Custom Error Handling in CodeIgniter
Apart from PHP’s inbuilt error reporting mechanism, CodeIgniter also allows you to handle errors via the `show_error()` and `log_message()` functions.
The `show_error()` function displays errors in an HTML format. It has two parameters: the error message and the HTTP response status code. This is ideal for displaying custom errors to the user.
```php show_error('This is a custom error message', 500); ```
The `log_message()` function is used to write log messages. It requires two arguments: the error level and the error message. The error level can be ‘error’, ‘debug’ or ‘info’.
```php log_message('error', 'An error occurred'); ```
Debugging in CodeIgniter
CodeIgniter provides a few helper functions that can aid in debugging your application.
- print_r() and var_dump() – These are native PHP functions but are commonly used in CodeIgniter for inspecting variables during debugging.
```php $data = array('apple', 'banana', 'cherry'); print_r($data); $details = array('name' => 'John', 'age' => 22); var_dump($details); ```
- Debugging Functions – CodeIgniter also provides some additional functions for debugging like `debug_helper()`, which is used to print array or object as a string.
```php $this->load->helper('debug'); $data = array('apple', 'banana', 'cherry'); debug_var($data); ```
Error Logging
It’s not always practical to find bugs by manually inspecting the code. Sometimes, the error occurs on the production server where debugging is not an option. In such cases, error logs come to the rescue.
You can enable error logging in CodeIgniter by modifying the `$config[‘log_threshold’]` configuration in the application/config/config.php file. There are different levels of logging available:
0 = Disables logging, Error logging TURNED OFF
1 = Error Messages (including PHP errors)
2 = Debug Messages
3 = Informational Messages
4 = All Messages
For example, to log all messages, you can set:
```php $config['log_threshold'] = 4; ```
Error logs are saved in the application/logs folder. Make sure this folder is writable. If the date is set in your config file, the log files will be named log-YYYY-MM-DD.php.
Troubleshooting Common CodeIgniter Errors
Now, let’s look at some common errors and how you can troubleshoot them.
- 404 Page Not Found: This error typically occurs when the controller or method specified in the URL doesn’t exist. To fix it, ensure that the controller and method names in your URL match exactly with their actual definitions in your code, considering case sensitivity.
- Database Error: Errors like ‘Unable to connect to your database server’ may occur due to incorrect database credentials or server unavailability. Check your database settings in application/config/database.php file and make sure your database server is running.
- Fatal error: Call to undefined function: This error occurs when you are trying to call a function that has not been defined or has not been loaded. To fix it, check if the function is defined and the file containing the function is properly loaded.
Conclusion
CodeIgniter’s built-in error handling and debugging tools can significantly ease the process of identifying and fixing issues. However, effective error handling is not just about fixing errors but preventing potential issues that might occur in the future. By leveraging these tools, you can create robust applications that handle errors gracefully and provide meaningful error messages to the users. Remember, the key to successful debugging is persistence and a systematic approach.
Table of Contents
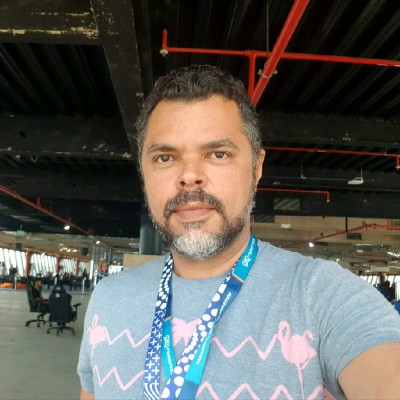
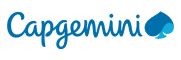