Diving Deep into CodeIgniter: An In-Depth Exploration of the PHP Framework
In the vast landscape of web development, PHP remains one of the dominant scripting languages due to its simplicity, flexibility, and robustness. Among the tools that have enhanced PHP’s prowess is CodeIgniter – a robust PHP framework that offers a streamlined, efficient platform for building web applications. This is why many businesses choose to hire CodeIgniter developers for their projects.
This ultimate guide explores CodeIgniter’s features, setup process, and use cases to help both developers and companies looking to hire CodeIgniter developers to leverage its full potential.
What is CodeIgniter?
CodeIgniter is an open-source PHP framework that simplifies the process of building dynamic web applications. It stands out for its lightweight nature, high performance, clear documentation, and broad compatibility with various hosting platforms. CodeIgniter employs an MVC (Model-View-Controller) architecture that compartmentalizes the various elements of an application for clean, manageable code.
Why Choose CodeIgniter?
- Lightweight and Fast
CodeIgniter is remarkably lightweight, with the core system requiring only a small library. Other libraries can be added on an as-needed basis, which enhances the speed and performance of the framework.
- MVC Architecture
CodeIgniter uses the Model-View-Controller architectural design, which organizes code into Models (data structure), Views (user interface), and Controllers (application logic), thereby promoting maintainability and scalability.
- Excellent Documentation
CodeIgniter has very well-documented code. Its user guide comes with a clear, systematic description of each component, making it a breeze to learn and implement.
- Built-in Security Features
CodeIgniter has numerous in-built security features, like input and output filtering, XSS filtering, and password handling, which simplify the process of building secure applications.
Setting up CodeIgniter
Before setting up CodeIgniter, ensure that you have PHP 5.6 or newer installed on your local development environment. The installation process is as follows:
- Download the latest version of CodeIgniter from the official website.
- Extract the downloaded file to your local server directory (for example, htdocs in XAMPP).
- Navigate to `http://localhost/<directory_name>` in your browser, where `<directory_name>` is the name of your extracted CodeIgniter folder. You should see a welcome message, confirming that CodeIgniter is successfully installed.
Building a Basic Application in CodeIgniter
Let’s build a simple “Hello World” application to demonstrate CodeIgniter’s basic functionality. This application will use the MVC architecture.
Step 1: Set Up the Controller
Create a new file named `Hello.php` in the `application/controllers` directory and add the following code:
```php <?php class Hello extends CI_Controller { public function index() { $this->load->view('hello_world'); } } ?>
This code creates a `Hello` controller with a single function, `index()`, which loads the `hello_world` view.
Step 2: Create the View
Create a new file named `hello_world.php` in the `application/views` directory and add the following code:
```php <html> <head> <title>Hello World</title> </head> <body> <h1>Hello, world!</h1> </body> </html>
This is the view that will be displayed when the `index()` function of the `Hello` controller is called.
Step 3: Test the Application
To test your application, navigate to `http://localhost/<directory_name>/index.php/hello`. You should see a webpage displaying “Hello, world!”
Enhancing Your Application with Models
Models in CodeIgniter are essential tools used to work with the data in your application. They contain functions that help you retrieve, insert, and update information in your database. Understanding these functions is a fundamental skill that people seek when they hire CodeIgniter developers.
For example, consider a `User_model` with functions to get and set user data:
```php <?php class User_model extends CI_Model { public function get_users() { // Query to fetch users from database } public function add_user($data) { // Query to add user to database } } ?>
In your Controller, you can load this model and use its functions:
```php <?php class User extends CI_Controller { public function index() { $this->load->model('User_model'); $users = $this->User_model->get_users(); $this->load->view('user_view', ['users' => $users]); } public function add() { // Load the form helper and library $this->load->helper('form'); $this->load->library('form_validation'); // Validate the form // ... // Add user to the database $this->load->model('User_model'); $this->User_model->add_user($this->input->post()); // Redirect to user index redirect('user/index'); } } ?>
The `User` controller uses the `User_model` to fetch and add users in the `index()` and `add()` methods, respectively.
Conclusion
This guide offers an insightful look into CodeIgniter, highlighting its key features, installation process, and basic usage. However, the framework is a versatile tool with myriad additional features like form validation, session management, email sending, and more. These complex features are often the reason why businesses opt to hire CodeIgniter developers. By delving deeper into CodeIgniter, whether as a developer or a business looking to hire CodeIgniter developers, you will uncover a wealth of tools to make your PHP development faster, cleaner, and more secure.
Table of Contents
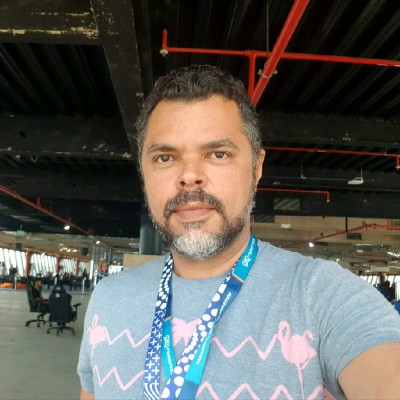
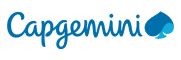