Mastering Dynamic Web Form Creation and Validation with CodeIgniter
CodeIgniter is a powerful PHP framework that simplifies web application development and empowers developers to write clean, efficient, and reusable code. This powerful tool makes it a wise decision to hire CodeIgniter developers for your projects. One aspect where CodeIgniter outshines others is in building dynamic web forms.
This blog post will guide you, or the CodeIgniter developers you hire, through the process of creating web forms using CodeIgniter, focusing on how to make them dynamic, adaptable, and user-friendly.
Introduction to CodeIgniter
CodeIgniter is known for its small footprint and high performance. It is not a restrictive PHP framework and offers a simple interface, comprehensive libraries, and a logical structure to access these libraries. The library is rich in features, enabling tasks like sending emails, uploading files, managing sessions, and much more.
CodeIgniter also provides a strong security infrastructure, including input and output filtering, encryption, and XSS filtering. It comes with complete tools for form validation and data sanitization, crucial for any data-intensive application like web forms.
Installing CodeIgniter
Installing CodeIgniter is a straightforward process that can be done in a few steps:
- Download CodeIgniter: The first step is to download the latest version of CodeIgniter from the official website. The download will be a .zip file.
- Extract the Downloaded File: Once the download is complete, you will need to extract the downloaded file. This will result in a folder that contains all the CodeIgniter files and folders.
- Move the CodeIgniter Files to the Web Root: You will need to move the extracted CodeIgniter folder to your web server’s root directory. This will typically be a folder named `htdocs` or `www`, depending on your server setup.
- Set the Base URL: After moving the CodeIgniter files, you’ll need to set the base URL for your application. This is done in the `application/config/config.php` file. You should set `$config[‘base_url’]` to the URL where you installed CodeIgniter.
- Check the Installation: To check if the installation was successful, open your web browser and navigate to the URL where you installed CodeIgniter. If you see a welcome message, that means CodeIgniter has been successfully installed.
These are the basic steps to get CodeIgniter installed and ready to use. Once installed, you can start building dynamic web applications using its various features and tools.
CodeIgniter Form Helper
CodeIgniter’s Form Helper is a set of functions that assist in working with forms. Instead of manually writing HTML form elements, you can use the Form Helper functions to generate them. This can make your code cleaner and easier to manage.
To use the Form Helper, it needs to be loaded in your controller using `$this->load->helper(‘form’);`.
Here are some of the commonly used Form Helper functions:
- `form_open(‘path’, $attributes)`: Generates an opening form tag. The first parameter is the path where the form data will be submitted, and the second optional parameter is an array of additional attributes.
- `form_input($data, $value, $extra)`: Generates a standard text input field. You can also pass data for name, id, value etc. in the first parameter as an associative array.
- `form_password($data, $value, $extra)`: Similar to `form_input()`, but generates a password input field.
- `form_upload($data, $value, $extra)`: Generates a file upload field.
- `form_submit($data, $value, $extra)`: Generates a submit button.
- `form_close($extra)`: Generates a closing form tag.
These are just a few of the available Form Helper functions. There are also functions for generating checkboxes, radio buttons, dropdowns, and more.
Using CodeIgniter’s Form Helper not only simplifies form creation but also helps with form validation and security by providing XSS filtering, which can be enabled by setting the second parameter of the `form_open()` function to TRUE. This makes the Form Helper a valuable tool in any CodeIgniter developer’s toolkit.
Setting up the Form Controller
Let’s create a form that allows users to register an account. We will start by creating a new controller called “Form”. This controller will handle all the routes related to our form.
```php <?php class Form extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form', 'url')); $this->load->library('form_validation'); } public function index() { $this->load->view('form_view'); } } ?>
In the above code, we have created a new class named `Form` that extends `CI_Controller`. In the constructor function, we load the form and URL helper and the form validation library.
Building the Registration Form
Now let’s create the form in `form_view.php`. We will use CodeIgniter’s Form Helper functions to generate form HTML. Our form will have fields for the username, email, password, and a password confirmation field.
```html <html> <head> <title>Registration Form</title> </head> <body> <?php echo form_open('form'); ?> <h5>Username</h5> <?php echo form_input('username', set_value('username')); ?> <h5>Password</h5> <?php echo form_password('password'); ?> <h5>Password Confirm</h5> <?php echo form_password('passconf'); ?> <h5>Email Address</h5> <?php echo form_input('email', set_value('email')); ?> <div><input type="submit" value="Submit" /></div> <?php echo form_close(); ?> </body> </html>
In the above code, which can be effectively implemented by those who hire CodeIgniter developers, `form_open(‘form’)` generates the opening form tag. The argument ‘form’ specifies the form data should be sent to the ‘form’ controller. The functions `form_input()` and `form_password()` are used to generate an input element of type text and a password field respectively. Another useful function is `set_value()`, often utilized by CodeIgniter developers, to repopulate the form field when there is an error or when the form is not validated. Such dynamic form management is among the reasons why many opt to hire CodeIgniter developers.
Conclusion
CodeIgniter provides a robust and intuitive environment for creating dynamic web forms, making it a compelling reason to hire CodeIgniter developers for your web projects. It’s comprehensive Form Helper and Form Validation library facilitates the easy and efficient construction of user-friendly forms. Not only does it help developers, including those you might hire as CodeIgniter developers, to quickly generate form HTML, but it also takes care of input validation, error handling, and repopulation of form fields. As such, CodeIgniter proves to be an excellent choice for any developer or business looking to enhance their web application’s interactive and dynamic features, making the process of form handling a breeze rather than a chore. Whether you’re a seasoned developer, a beginner stepping into the world of PHP web development, or a business looking to hire CodeIgniter developers, mastering CodeIgniter will certainly take your skills or your project to the next level.
Table of Contents
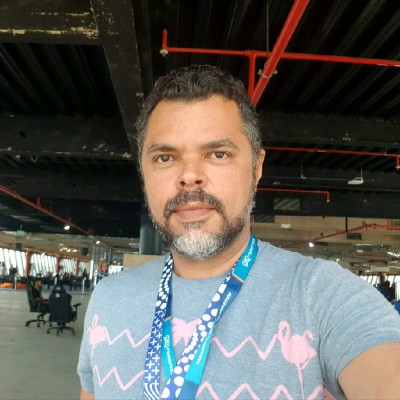
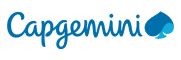