Enhancing Web Security with CodeIgniter’s Form Validation Library
Form validation is the process of ensuring that the data entered by users fits the expected structure, format, and type. It also implies the removal of any potential malicious or harmful data. In web development, form validation is typically performed both on the client-side (for a user-friendly interface) and the server-side (for security and data integrity).
In CodeIgniter, form validation is conveniently handled by the Form Validation Library, which provides a set of built-in rules and functions to validate user input, allowing you to create more secure, robust web applications.
Setting Up Form Validation in CodeIgniter
To use CodeIgniter’s Form Validation Library, we first need to load it in the controller. This can be achieved with the `$this->load->library(‘form_validation’)` statement. After loading the library, we can then use `$this->form_validation->set_rules()` to define our validation rules for form fields.
Here’s a basic example of a controller in CodeIgniter with form validation:
```php <?php class FormController extends CI_Controller { public function index() { // Load the form validation library $this->load->library('form_validation'); // Set form validation rules $this->form_validation->set_rules('username', 'Username', 'required'); $this->form_validation->set_rules('password', 'Password', 'required'); // Check if form validation fails or passes if ($this->form_validation->run() == FALSE) { // Validation failed, show error messages $this->load->view('myform'); } else { // Validation passed, process data $this->load->view('formsuccess'); } } } ?> ```
In this example, we have two fields, username and password, which we have marked as required. If the user submits the form without filling in these fields, CodeIgniter will automatically generate an error message.
Validation Rules
CodeIgniter allows you to set multiple rules for each form field using a pipe-separated list. This includes built-in rules such as `required`, `min_length`, `max_length`, `valid_email`, `matches`, etc.
Let’s look at an example where we apply multiple validation rules:
```php <?php class RegisterController extends CI_Controller { public function index() { $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required|min_length[5]|max_length[12]'); $this->form_validation->set_rules('password', 'Password', 'required|min_length[8]'); $this->form_validation->set_rules('passwordConf', 'Password Confirmation', 'required|matches[password]'); $this->form_validation->set_rules('email', 'Email', 'required|valid_email'); if ($this->form_validation->run() == FALSE) { $this->load->view('registerform'); } else { $this->load->view('registersuccess'); } } } ?> ```
In the example above, the `username` field must be between 5 to 12 characters, the `password` field must have a minimum of 8 characters, and the `passwordConf` field must match the `password` field. The `email` field must contain a valid email address. If any of these conditions are not met, the form submission will fail, and the user will be shown an error message.
Custom Callbacks
One of the powerful features of CodeIgniter’s Form Validation library is the ability to create custom validation rules using callbacks. A callback is a user-defined function that gets executed when the form data is validated against it.
For instance, let’s create a custom callback function to check if a username already exists in our database:
```php <?php class RegisterController extends CI_Controller { public function index() { $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'callback_username_check'); // ... other rules if ($this->form_validation->run() == FALSE) { $this->load->view('registerform'); } else { $this->load->view('registersuccess'); } } // Callback function public function username_check($str) { // load the model $this->load->model('user_model'); if ($this->user_model->check_username_exists($str)) { $this->form_validation->set_message('username_check', 'The {field} field already exists.'); return FALSE; } else { return TRUE; } } } ?> ```
In this example, the `username_check` callback function is linked to the `username` field. This function checks the database for the existence of the username. If it exists, it sets an error message, returns `FALSE`, and form validation fails.
Error Messages
CodeIgniter allows you to customize error messages for each validation rule. You can use the `$this->form_validation->set_message()` function to set a custom error message. The `set_message` function accepts two parameters: the name of the rule and the error message.
Here’s an example:
```php <?php class RegisterController extends CI_Controller { public function index() { $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required|min_length[5]'); $this->form_validation->set_message('required', '{field} is required.'); $this->form_validation->set_message('min_length', '{field} must be at least {param} characters long.'); // ... rest of the code } } ?> ```
In this example, the `required` and `min_length` validation rules will now display custom error messages. The `{field}` and `{param}` placeholders will be replaced by the actual field name and parameter value, respectively.
Conclusion
Form validation is crucial to maintaining the security, usability, and integrity of your web application. CodeIgniter’s Form Validation library provides a powerful, flexible way to ensure that user input is valid, sanitized, and safe.
With built-in validation rules, customizable error messages, and the ability to define your own custom callback functions, you can create comprehensive validation routines that fit your application’s unique needs.
Remember that while form validation is a critical part of application security, it should not be the only measure taken to protect your application. Always adhere to best practices for secure coding to protect your application against potential threats.
Table of Contents
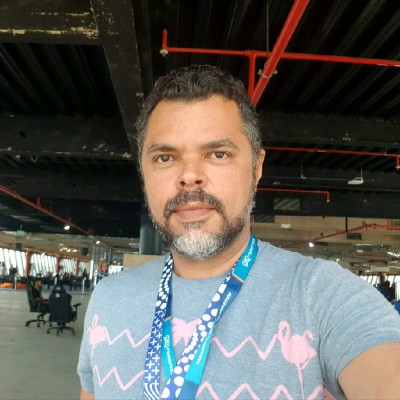
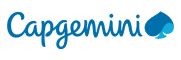