CodeIgniter Q & A
How to implement full-text search functionality in CodeIgniter?
Implementing full-text search functionality in a CodeIgniter application can significantly enhance the user experience by allowing users to find relevant information quickly and efficiently. Here’s a step-by-step guide on how to implement full-text search in CodeIgniter:
- Database Setup: First, ensure that your database table, which you want to search within, is using the MyISAM or InnoDB storage engine. These engines support full-text indexing, a prerequisite for full-text search.
- Enable Full-Text Indexing: In your database table, specify the columns you want to enable for full-text indexing. You can do this while creating the table or alter an existing table. For example:
```sql ALTER TABLE products ADD FULLTEXT(product_name, product_description); ```
- Create a Search Form: In your CodeIgniter view, create a search form that allows users to input their search query. This form should submit a POST request to a designated search controller method.
- Controller Method: In your CodeIgniter controller, create a method to handle the search request. Retrieve the user’s search query from the POST data and sanitize it to prevent SQL injection.
- Model Function: Create a model function that constructs the SQL query for full-text search. Use the `MATCH` and `AGAINST` operators to perform the search. For example:
```php $query = $this->db->select('*') ->from('products') ->where('MATCH(product_name, product_description) AGAINST("' . $search_query . '")', NULL, FALSE) ->get(); ```
- Display Results: Pass the search results to your view for display. You can loop through the results and present them to the user in a user-friendly format.
- Pagination: If your search results may span multiple pages, consider implementing pagination to improve user experience.
- Optimize and Fine-Tune: Full-text search can be resource-intensive, so it’s important to optimize your queries and database indexing for performance. You can explore features like relevance ranking, stemming, and stop words to improve the accuracy of your search results.
- Error Handling: Implement error handling to gracefully handle scenarios where no results are found or other unexpected issues arise during the search.
- Testing: Thoroughly test your search functionality to ensure it behaves as expected and provides relevant results to users.
By following these steps, you can successfully implement full-text search functionality in your CodeIgniter application, making it easier for users to find the information they need quickly and efficiently.
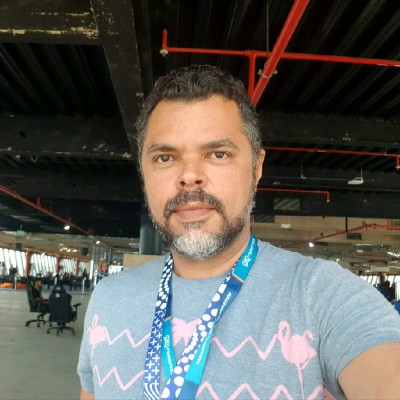
Previously at
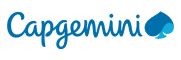
Experienced Full Stack Systems Analyst, Proficient in CodeIgniter with extensive 5+ years experience. Strong in SQL, Git, Agile.