Essential Image Manipulation Techniques with CodeIgniter
One of the common tasks in any web application is handling image uploads and editing. In this blog post, we’ll be using CodeIgniter, a powerful PHP framework, to carry out these tasks. CodeIgniter offers robust libraries for managing images, making it quite easy to implement these features.
First, let’s quickly brush up on CodeIgniter’s essentials. CodeIgniter is an MVC (Model, View, Controller) framework. This design pattern separates the data layer, user interface, and control flow into distinct components, making it easier for developers to manage complex applications.
Now, let’s dive into how we can use CodeIgniter for image uploading and editing.
Uploading Images
The first step in handling images is uploading them. CodeIgniter provides the File Uploading Class for this purpose. Below is an example of how to use it:
```php class Upload extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form', 'url')); } public function index() { $this->load->view('upload_form', array('error' => ' ' )); } public function do_upload() { $config['upload_path'] = './uploads/'; $config['allowed_types'] = 'gif|jpg|png'; $config['max_size'] = 1000; $config['max_width'] = 1024; $config['max_height'] = 768; $this->load->library('upload', $config); if (!$this->upload->do_upload('userfile')) { $error = array('error' => $this->upload->display_errors()); $this->load->view('upload_form', $error); } else { $data = array('upload_data' => $this->upload->data()); $this->load->view('upload_success', $data); } } } ```
This controller loads the form helper and url helper in the constructor. The `do_upload()` function first sets the configuration for the upload (like where the files should be uploaded, which file types are allowed, and the maximum size, width, and height). After setting up the configuration, the upload library is loaded and the upload is executed.
If the upload is successful, the user is redirected to the ‘upload_success’ view with the upload data. If the upload fails, the user is redirected back to the ‘upload_form’ view, and the error messages are displayed.
The ‘userfile’ used in `do_upload(‘userfile’)` corresponds to the name attribute of the file input form.
Editing Images
Once you’ve managed to upload images, you may often find the need to edit them. Common editing operations might include resizing, cropping, or rotating images. Fortunately, CodeIgniter also offers a robust Image Manipulation Class to help us do that.
1. Resizing Images
Here’s an example of resizing an image:
```php class Images extends CI_Controller { public function __construct() { parent::__construct(); $this->load->library('image_lib'); } public function resize() { $config['image_library'] = 'gd2'; $config['source_image'] = '/path/to/image/mypic.jpg'; $config['create_thumb'] = TRUE; $config['maintain_ratio'] = TRUE; $config['width'] = 75; $config['height'] = 50; $this->image_lib->clear(); $this->image_lib->initialize($config); if (!$this->image_lib->resize()) { echo $this->image_lib->display_errors(); } else { echo "Image resized successfully"; } } } ```
This controller loads the image library in the constructor. The `resize()` function sets the configuration for the image manipulation and calls the `resize()` method. If the operation fails, the errors are displayed, and if it succeeds, a success message is shown.
2. Cropping Images
Cropping is another common operation, here’s an example:
```php public function crop() { $config['image_library'] = 'gd2'; $config['source_image'] = '/path/to/image/mypic.jpg'; $config['width'] = 200; $config['height'] = 200; $config['x_axis'] = 100; $config['y_axis'] = 60; $this->image_lib->clear(); $this->image_lib->initialize($config); if (!$this->image_lib->crop()) { echo $this->image_lib->display_errors(); } else { echo "Image cropped successfully"; } } ```
This works quite similarly to the resizing operation but calls the `crop()` method instead.
3. Rotating Images
Finally, let’s take a look at how to rotate an image:
```php public function rotate() { $config['image_library'] = 'gd2'; $config['source_image'] = '/path/to/image/mypic.jpg'; $config['rotation_angle'] = '90'; $this->image_lib->clear(); $this->image_lib->initialize($config); if (!$this->image_lib->rotate()) { echo $this->image_lib->display_errors(); } else { echo "Image rotated successfully"; } } ```
Again, this is similar to the previous operations, but this time, we use the `rotate()` method. The `rotation_angle` can be ’90’, ‘180’, or ‘270’.
Conclusion
In this blog post, we’ve gone through how to handle image uploads and edits in a CodeIgniter application. CodeIgniter provides powerful, easy-to-use libraries for these tasks. By leveraging these libraries, you can easily manage image files in your application without the need for third-party libraries or complex code.
Remember that the examples provided in this post are just the tip of the iceberg. CodeIgniter’s libraries offer a lot more options and capabilities that you can harness according to your application’s needs. So, do explore the official CodeIgniter documentation for more in-depth information.
Table of Contents
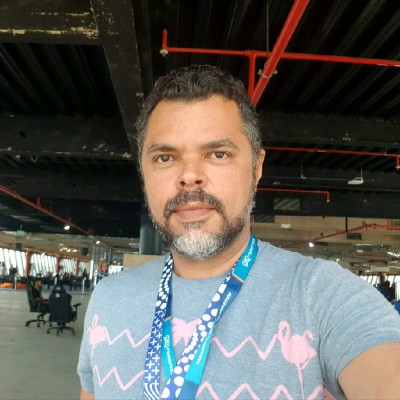
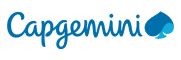