How to implement a file upload feature with CodeIgniter?
Implementing a file upload feature in CodeIgniter is a common requirement for many web applications, especially when dealing with user-generated content, such as profile pictures, documents, or media files. CodeIgniter provides a straightforward way to handle file uploads. Here’s a step-by-step guide on how to implement a file upload feature:
- Setup Configuration:
First, ensure that the `multipart/form-data` enctype is set in your HTML form, which is required for file uploads. Your form tag should look like this:
```html <form method="post" action="upload_controller/upload" enctype="multipart/form-data"> ```
- Create an Upload Controller:
Create a controller in CodeIgniter that will handle the file upload. In this controller, you will define the upload configuration and process the uploaded file. Here’s a basic example:
```php // application/controllers/Upload_controller.php class Upload_controller extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form', 'url')); } public function upload() { $config['upload_path'] = './uploads/'; // Set the upload directory $config['allowed_types'] = 'gif|jpg|jpeg|png|pdf'; // Specify allowed file types $config['max_size'] = 2048; // Set a maximum file size (in kilobytes) $this->load->library('upload', $config); if (!$this->upload->do_upload('userfile')) { $error = array('error' => $this->upload->display_errors()); $this->load->view('upload_form', $error); } else { $data = array('upload_data' => $this->upload->data()); $this->load->view('upload_success', $data); } } } ```
- Create Views:
Create views to display the file upload form and the success/error messages. In the example above, we load views named ‘upload_form’ and ‘upload_success’.
- Handle File Upload:
In the controller, we use the CodeIgniter Upload library to handle the file upload. The `do_upload()` method checks if the upload is successful, and if not, it displays errors. If the upload is successful, it provides information about the uploaded file.
- Handle Uploaded File:
You can further process the uploaded file as needed, such as storing its details in a database, moving the file to a specific location, or generating thumbnails.
By following these steps, you can easily implement a file upload feature in your CodeIgniter application, allowing users to upload files to your server. Be sure to handle security considerations like file type validation, maximum file size, and sanitizing user inputs to ensure a secure and robust implementation.
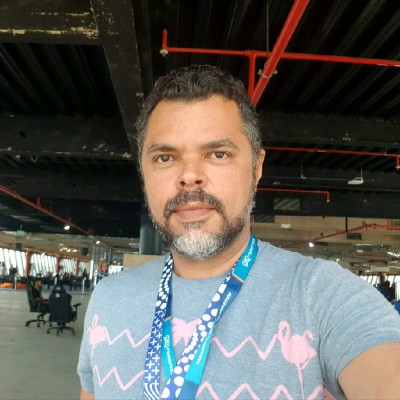
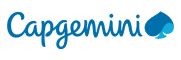