CodeIgniter Developer Interview: Top Questions and Proven Techniques
CodeIgniter is a popular PHP framework known for its simplicity and ease of use, making it a preferred choice for web application development. When hiring CodeIgniter developers, it is essential to assess their technical skills, experience with the framework, problem-solving abilities, and familiarity with PHP and MySQL to ensure you find the right fit for your projects.
Table of Contents
This comprehensive interview guide will help you identify top CodeIgniter talent and build a skilled development team.
1. Top Skills of CodeIgniter Developers to Look Out For:
Before diving into the interview process, let’s highlight the key skills you should look for in CodeIgniter developers:
- Proficiency in PHP: Strong knowledge and experience in PHP development and object-oriented programming (OOP) principles.
- CodeIgniter Framework Expertise: In-depth understanding of CodeIgniter’s architecture, routing, and MVC (Model-View-Controller) pattern.
- Front-end Technologies: Familiarity with HTML, CSS, and JavaScript/jQuery for building responsive and interactive web applications.
- Database Management: Experience in working with MySQL or other relational databases to design and optimize database schema.
- Security Best Practices: Knowledge of security vulnerabilities and best practices to ensure secure web application development.
- RESTful APIs: Understanding of building and consuming RESTful APIs for seamless integration with external services.
2. Overview of the CodeIgniter Developer Hiring Process:
- Defining Job Requirements & Skills: Clearly outline the technical skills and experience you are looking for in a CodeIgniter developer, including proficiency in PHP, CodeIgniter framework, front-end technologies, and database management.
- Creating an Effective Job Description: Craft a comprehensive job description with a clear title, specific requirements, and details about the projects the developer will work on.
- Preparing Interview Questions: Develop a list of technical questions to assess candidates’ CodeIgniter knowledge, problem-solving abilities, and experience in web application development.
- Evaluating Candidates: Use a combination of technical assessments, coding challenges, and face-to-face interviews to evaluate potential candidates and determine the best fit for your organization.
3. Technical Interview Questions and Sample Answers:
Q1. Explain the MVC pattern and how it is implemented in CodeIgniter.
Sample Answer:
The Model-View-Controller (MVC) pattern separates the application into three components: Model (for data handling), View (for user interface), and Controller (for managing user requests and interactions). In CodeIgniter, the Model represents the data and database interactions, View handles the presentation and user interface, and Controller processes user requests, interacts with the Model, and renders the appropriate View.
Q2. How do you load a CodeIgniter helper and library in a controller?
Sample Answer:
To load a CodeIgniter helper or library in a controller, we use the ‘load’ method. For example, to load a ‘URL’ helper and a ‘Database’ library:
// Load URL Helper $this->load->helper('url'); // Load Database Library $this->load->library('database');
Q3. Explain the concept of routing in CodeIgniter. How do you define custom routes?
Sample Answer:
Routing in CodeIgniter maps URLs to specific controllers and methods. The ‘routes.php’ file in the ‘config’ folder defines the routing rules. For custom routes, we use the ‘route’ method. For example, to create a custom route for ‘example.com/products’ to map to ‘Products’ controller and ‘index’ method:
$route['products'] = 'Products/index';
Q4. How do you validate form data in CodeIgniter, and what built-in validation functions does it offer?
Sample Answer:
CodeIgniter provides form validation as part of its form helper. To validate form data, we use the ‘form_validation’ library and set validation rules using the ‘set_rules’ method. Some built-in validation functions include ‘required’, ‘min_length’, ‘max_length’, ‘numeric’, ‘valid_email’, etc.
// Example of setting validation rules $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required|min_length[5]|max_length[12]');
Q5. How do you handle file uploads in CodeIgniter, and what are the key configuration settings to consider?
Sample Answer:
To handle file uploads in CodeIgniter, we use the ‘upload’ library. Key configuration settings include ‘upload_path’ to specify the directory where files will be uploaded, ‘allowed_types’ to define the allowed file types, and ‘max_size’ to set the maximum file size allowed.
$config['upload_path'] = './uploads/'; $config['allowed_types'] = 'gif|jpg|png'; $config['max_size'] = 2048; $this->load->library('upload', $config); if ($this->upload->do_upload('file')) { // File uploaded successfully } else { // Error in file upload }
Q6. How do you use CodeIgniter’s database class to perform CRUD operations?
Sample Answer:
CodeIgniter’s database class provides a convenient way to perform CRUD (Create, Read, Update, Delete) operations. We can use methods like ‘insert’, ‘get’, ‘update’, and ‘delete’ to interact with the database. For example:
// Insert data into the database $data = array('name' => 'John', 'email' => 'john@example.com'); $this->db->insert('users', $data); // Retrieve data from the database $query = $this->db->get('users'); $result = $query->result(); // Update data in the database $data = array('name' => 'Jane'); $this->db->where('id', 1); $this->db->update('users', $data); // Delete data from the database $this->db->where('id', 1); $this->db->delete('users');
Q7. Explain how you can use CodeIgniter’s session class to manage user sessions.
Sample Answer:
CodeIgniter’s session class provides a way to manage user sessions. To start a session, we load the ‘session’ library. We can set session data using the ‘set_userdata’ method and retrieve it using the ‘userdata’ method. For example:
// Load session library $this->load->library('session'); // Set session data $data = array('user_id' => 123, 'username' => 'john_doe'); $this->session->set_userdata($data); // Retrieve session data $user_id = $this->session->userdata('user_id'); $username = $this->session->userdata('username');
Q8. How do you implement pagination in CodeIgniter to display large sets of data?
Sample Answer:
CodeIgniter provides a pagination library to implement pagination. We need to configure pagination settings and load the ‘pagination’ library. Then, we can use the ‘limit’ and ‘offset’ methods to retrieve a specific subset of data based on the current page number. For example:
// Load pagination library $this->load->library('pagination'); // Configure pagination settings $config['base_url'] = 'https://example.com/products'; $config['total_rows'] = 1000; $config['per_page'] = 10; $this->pagination->initialize($config); // Retrieve data for the current page $page = $this->uri->segment(3) ? $this->uri->segment(3) : 0; $this->db->limit($config['per_page'], $page); $query = $this->db->get('products'); $result = $query->result();
Q9. How do you handle user authentication and authorization in CodeIgniter?
Sample Answer:
User authentication and authorization can be managed using CodeIgniter’s ‘session’ library and custom access control logic. After a successful login, we can set user-related data in the session to identify the authenticated user. Additionally, we can use custom middleware or hooks to handle role-based authorization and restrict access to certain parts of the application based on user roles.
Q10. Explain how CodeIgniter handles error handling and logging.
Sample Answer:
CodeIgniter provides error handling and logging mechanisms to track and troubleshoot application errors. The ‘log_message’ function is used to log custom messages, warnings, and errors. Error logging can be enabled in the ‘config.php’ file by setting the ‘log_threshold’ value. When an error occurs, CodeIgniter shows the corresponding error page along with logged error details, helping developers identify and resolve issues efficiently.”
4. Importance of the Interview Process to Find the Right CodeIgniter Talent:
The interview process plays a crucial role in finding the right CodeIgniter developers for your projects. Assessing technical skills, hands-on experience with the framework, problem-solving abilities, and understanding of web application development are vital to building a competent development team.
A thorough interview process helps in identifying developers who not only possess the technical expertise but also align with your company’s culture and values. CodeIgniter developers who can effectively collaborate with the team and contribute innovative solutions are key to the success of your web application projects.
5. How CloudDevs Can Help You Hire CodeIgniter Developers:
Hiring CodeIgniter developers can be challenging, especially when you have specific requirements and project deadlines to meet. CloudDevs offers a platform that simplifies the hiring process by providing access to a curated pool of pre-screened senior CodeIgniter developers.
With CloudDevs, you can:
- Connect with a dedicated consultant to discuss your project requirements and desired skill sets.
- Receive a shortlist of top CodeIgniter developers within 24 hours, saving you time and effort in the initial screening process.
- Conduct interviews and assess candidates’ technical skills through coding challenges and face-to-face interviews.
- Start a no-risk trial with your selected CodeIgniter developer to ensure the perfect fit for your projects.
By partnering with CloudDevs, you gain access to highly skilled CodeIgniter developers who can deliver high-quality web applications and drive your business growth. Let CloudDevs handle the hiring process while you focus on building innovative web solutions.
Table of Contents
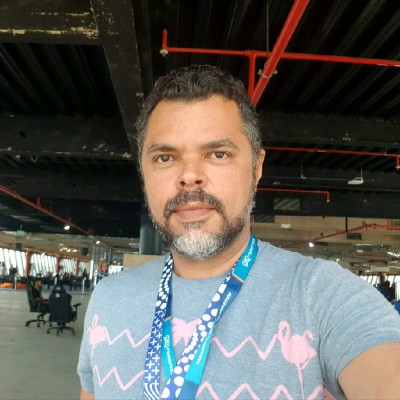
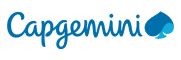