A Comprehensive Guide: Taking Your CodeIgniter App to the Next Level with Microservices
In today’s evolving technological landscape, building scalable web applications is more important than ever. When it comes to PHP frameworks, CodeIgniter has been a favored choice of many developers due to its simplicity, performance, and modularity. But how does it fit into the new age of microservices? In this post, we’ll explore how to use CodeIgniter with a microservices architecture to create a scalable and efficient application.
1. Understanding Microservices
Microservices, also known as the microservices architecture, is a software architectural style that breaks applications into smaller, manageable, and loosely coupled services. These services run as independent processes and communicate with each other via well-defined APIs.
The primary benefits of microservices include:
– Scalability: Individual services can be scaled independently.
– Flexibility: Services can be developed, deployed, and scaled using different technologies.
– Reliability: Failure in one service doesn’t necessarily bring down the entire application.
2. Why CodeIgniter?
While CodeIgniter wasn’t explicitly designed for microservices, its lightweight and modular nature makes it suitable for building individual services. Some reasons why you might consider CodeIgniter for your microservices architecture include:
– Performance: CodeIgniter is known for its speed and efficiency.
– Simplicity: It’s easy to set up and doesn’t force convention.
– Flexibility: Allows developers to integrate third-party libraries easily.
3. Building a Microservice with CodeIgniter
3.1. Setup a new CodeIgniter project
Assuming you have Composer and PHP installed, create a new project:
```bash composer create-project codeigniter4/appstarter codeigniter-microservice ```
3.2. Create an API Controller
For our example, let’s create a simple User service. First, let’s make a new controller:
```bash php spark make:controller API/Users ```
Inside the controller, let’s create a method to fetch user details:
```php public function getUser($id) { // Fetch the user from the database (this is a mockup for simplicity) $user = [ 'id' => $id, 'name' => 'John Doe', 'email' => 'john.doe@example.com' ]; return $this->response->setJSON($user); } ```
3.3. Define routes
In `app/Config/Routes.php`, set up a route for the API endpoint:
```php $routes->group('api', function($routes) { $routes->get('users/(:num)', 'API\Users::getUser/\'); }); ```
4. Connecting Microservices
In a real-world scenario, you’d have multiple such microservices. These services would communicate with each other via HTTP or any other lightweight protocol.
Imagine a scenario where you have another service, an `Order Service`, which needs to fetch user details. You’d make an HTTP request to the User service:
```php $httpClient = \Config\Services::curlrequest(); $response = $httpClient->request('GET', 'http://user-service/api/users/1'); $user = $response->getBody(); ```
5. Best Practices
- Separate Database for Each Service: Each microservice should have its own database to ensure that services are loosely coupled.
- Stateless Services: Ensure that your services are stateless. This makes them easier to scale because any instance of a service can handle any request.
- Monitoring and Logging: Since debugging issues can be more challenging in a distributed system, ensure that you have proper monitoring and logging in place.
- Service Discovery: As the number of services grows, consider using service discovery tools like Consul or Eureka to keep track of service instances.
Conclusion
CodeIgniter and microservices might seem an unusual pair, but their combination can result in a high-performing and scalable architecture. By leveraging CodeIgniter’s simplicity and performance, one can create lightweight and efficient services that align well with the microservices paradigm.
However, it’s essential to be mindful of the complexities that come with microservices. As with any architectural decision, weigh the benefits against the challenges and determine if it’s the right fit for your application. But with the right practices in place, CodeIgniter can become a powerful tool in your microservices toolkit.
Table of Contents
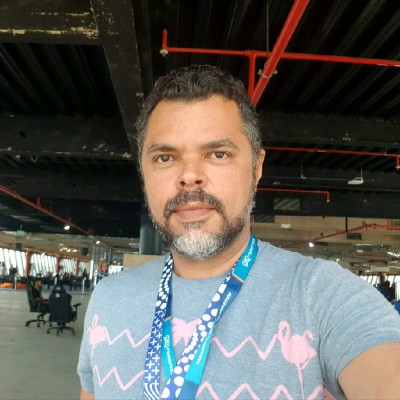
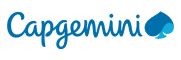