Bridge Language Barriers: Multilingual Apps Made Easy with CodeIgniter
Building multilingual applications can be a daunting task, especially when it comes to integrating different languages into one cohesive platform. However, CodeIgniter, an open-source software web framework, has simplified this process through internationalization. In this blog post, we’ll dive into the process of internationalization using CodeIgniter, providing you with detailed examples to guide you along the way.
What is Internationalization?
Internationalization, often abbreviated as i18n (because there are 18 letters between the ‘i’ and ‘n’ in Internationalization), is the process of designing and preparing your software to be adaptable to different languages and regions without necessitating design changes.
Why is Internationalization Important?
Internationalization is crucial for any business aiming to provide services to a global audience. Without it, businesses risk alienating a large portion of potential customers who may not be able to interact with the application due to language barriers.
CodeIgniter’s internationalization support makes the framework a great choice for developers looking to build globally friendly applications.
CodeIgniter Internationalization: A Step-by-Step Guide
Step 1: Setup
Assuming you have CodeIgniter already installed, the first step involves downloading and setting up the language files. CodeIgniter provides an easy way to manage language files, which are essentially PHP files returning an array of key-value pairs, where keys are the English version and values are the translations.
Let’s create a language file for French. In the `application/language` directory, create a new folder named `french` and within it, create a file `message_lang.php`:
```php <?php $lang['welcome_message'] = 'Bienvenue sur notre application!'; $lang['logout_message'] = 'Vous avez été déconnecté avec succès.'; // You can add as many messages as you want ```
You would do this for every language your application will support, using the appropriate folder name for each language (e.g., `german`, `spanish`, etc.).
Step 2: Loading Language Files
Now, it’s time to load these language files. CodeIgniter provides the `$this->lang->load()` method for this purpose. In your controller, you might have something like this:
```php <?php class Welcome extends CI_Controller { public function __construct() { parent::__construct(); // Assume 'english' is the default language. $this->lang->load('message', 'english'); } public function index() { $this->load->view('welcome_message'); } } ```
In this example, the second argument of `$this->lang->load()` is the language directory.
Step 3: Using the Language Keys
Now that the language file is loaded, we can use the keys defined in the language files in our views. CodeIgniter provides the `lang()` helper function for this purpose:
```php <h1><?php echo lang('welcome_message'); ?></h1> <p><?php echo lang('logout_message'); ?></p> ```
If the current language is English, this will display:
``` Welcome to our application! You have been successfully logged out. ```
Step 4: Switching Languages
To allow users to switch languages, we need to detect their choice and load the appropriate language file. We can store the user’s language preference in a session. Here’s a controller method that changes the language:
```php public function switchLang($lang = 'english') { $this->session->set_userdata('site_lang', $lang); redirect($_SERVER['HTTP_REFERER']); } ```
In this method, we set the chosen language in the session and then redirect the user back to the page they were on. You can call this method from a language selector on your website.
Now, in the constructor, we load the language file based on the user’s preference:
```php public function __construct() { parent::__construct(); $siteLang = $this->session->userdata('site_lang'); $this->lang->load('message', $siteLang); } ```
Step 5: Language Auto-detection
In some scenarios, you may want to detect the user’s preferred language based on their browser settings. CodeIgniter’s Input class provides a method to do this, `$this->input->server(‘HTTP_ACCEPT_LANGUAGE’)`. You can use this to detect the language and set it as the default if the user hasn’t chosen a language yet.
```php public function __construct() { parent::__construct(); $siteLang = $this->session->userdata('site_lang'); if ($siteLang) { $this->lang->load('message', $siteLang); } else { // Extract language from HTTP_ACCEPT_LANGUAGE and load it $browserLang = substr($this->input->server('HTTP_ACCEPT_LANGUAGE'), 0, 2); $this->lang->load('message', $browserLang); } } ```
Final Words
CodeIgniter’s support for internationalization enables developers to build applications ready for a global audience, breaking down language barriers. With the steps detailed above, you can integrate multilingual support into your CodeIgniter application, enhancing user experience and broadening your application’s reach. Remember, an application that speaks the user’s language is an application that values its users.
Table of Contents
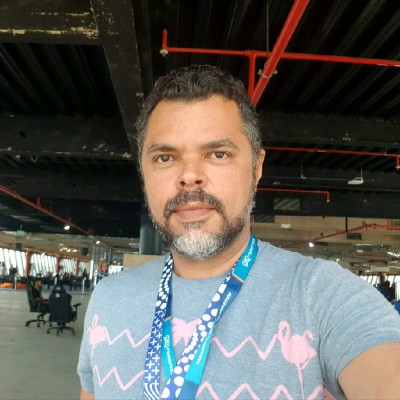
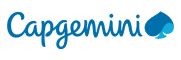