Say Goodbye to Cluttered Data: CodeIgniter Pagination to the Rescue
The management of vast sets of data is a common concern in web development, especially when dealing with databases that store thousands or even millions of records. Delivering such a huge amount of information to the user at once isn’t just impractical; it’s overwhelming and significantly slows down page load times. That’s where pagination comes into play, and for anyone working in PHP, CodeIgniter offers a handy library for this purpose.
In this blog post, we’ll explore how to leverage the pagination class of the CodeIgniter framework to manage large data sets effectively.
Understanding Pagination
In simple terms, pagination is the process of dividing content into separate pages. You’ve undoubtedly seen this in action on blogs, search engine results, or e-commerce sites. By implementing pagination, we ensure that the user interface remains clean, organized, and easy to navigate, making the user experience significantly more enjoyable.
The CodeIgniter Pagination Class
CodeIgniter, an open-source software web framework built for PHP coders, includes a built-in pagination class that simplifies the whole process of creating pagination. It comes with a wide array of options that can be customized to fit your specific requirements.
Configuring the Pagination
The first step in setting up pagination in CodeIgniter is loading the Pagination library.
```php $this->load->library('pagination'); ```
Next, we need to configure the options. CodeIgniter’s pagination class allows you to customize many options such as the base URL, the total number of rows, and the number of records per page.
```php $config['base_url'] = 'http://example.com/index.php/test/page/'; $config['total_rows'] = 200; $config['per_page'] = 20; $this->pagination->initialize($config); ```
In this example, ‘base_url’ is the URL you want to paginate. ‘total_rows’ is the total number of rows in the result set. And ‘per_page’ is the number of records you want to display per page.
Let’s get more practical and see how pagination works with a real database.
Implementing Pagination with a Database
Suppose we have a ‘users’ table and we want to display 10 users per page. First, in our controller, we’ll load the necessary library and helper, and get the total number of users.
```php $this->load->library('pagination'); $this->load->helper('url'); $config['base_url'] = site_url('users/index'); $config['total_rows'] = $this->db->count_all('users'); $config['per_page'] = 10; $this->pagination->initialize($config); ```
Next, we want to fetch the appropriate set of data from the database, based on the current page.
```php $offset = $this->uri->segment(3); // Get offset from URL $data['users'] = $this->db->get('users', $config['per_page'], $offset)->result(); ```
Here we are using the ‘get’ method of the database class to fetch 10 users at a time from the database.
Finally, we pass the data to our view and create the links for the pagination.
```php $data['links'] = $this->pagination->create_links(); $this->load->view('user_view', $data); ```
In our view file ‘user_view.php’, we will display the users and the links.
```php foreach ($users as $user) { echo $user->name . '<br>'; } echo $links; ```
Customizing the Links
The CodeIgniter Pagination class offers numerous options for customizing the generated links. For instance, you can set the ‘first_link’, ‘next_link’, ‘prev_link’, ‘last_link’, and more.
```php $config['first_link'] = 'First'; $config['last_link'] = 'Last'; $config['next_link'] = 'Next'; $config['prev_link'] = 'Previous'; $config['cur_tag_open'] = '<strong>'; $config['cur_tag_close'] = '</strong>'; $this->pagination->initialize($config); ```
In this example, ‘first_link’ and ‘last_link’ are the text you want to be used for the “First” and “Last” links. ‘next_link’ and ‘prev_link’ are the text for the “Next” and “Previous” links. ‘cur_tag_open’ and ‘cur_tag_close’ are the opening and closing tags around the selected page number.
Conclusion
Pagination is a crucial feature for any application dealing with large amounts of data. It not only provides a clean, organized interface but also enhances the user experience by making data navigation more manageable. CodeIgniter’s Pagination class is a powerful tool that simplifies the implementation of pagination. With its extensive customization options, developers can easily tailor it to suit their specific needs.
Remember to keep exploring and playing around with different configurations of the CodeIgniter Pagination class to get the most out of it. With practice and patience, you will master pagination and be able to effectively manage large data sets in your applications.
Table of Contents
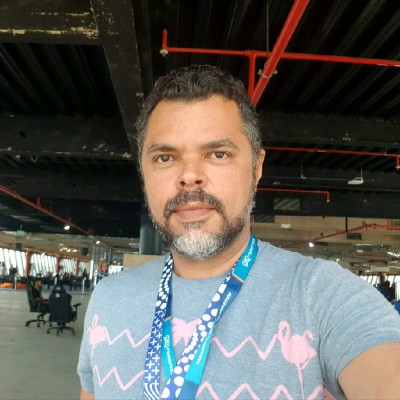
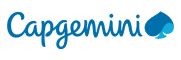