A Complete Roadmap to Building RESTful APIs with CodeIgniter
In this modern digital era, APIs have become a critical part of web and mobile applications. They are the unseen glue connecting the front-end and back-end, ensuring that everything runs smoothly. In particular, RESTful APIs have gained popularity for their simplicity, scalability, and flexibility.
Among many frameworks that enable you to create RESTful APIs, CodeIgniter stands out with its simplicity, lightweight, and speed. This article will guide you through the process of building web services using RESTful APIs in CodeIgniter.
Introduction to REST and CodeIgniter
Before we dive into the code, let’s start with the basics.
REST (Representational State Transfer) is an architectural style for designing networked applications. It relies on stateless, client-server communication, which can be cached to improve performance. It uses HTTP methods like GET, POST, PUT, DELETE for interaction.
CodeIgniter is a powerful PHP framework that enables developers to build dynamic web applications rapidly. It provides libraries for connecting to databases and performing operations like sending emails, uploading files, managing sessions, etc.
Setting Up CodeIgniter
Before we begin, ensure you have PHP, MySQL, and a server like Apache installed on your machine. To set up CodeIgniter, follow these steps:
- Download the latest version of CodeIgniter from the official website.
- Unzip the package.
- Upload the CodeIgniter folders and files to your server. Normally, the index.php file will be at your root.
- Open the application/config/config.php file with a text editor and set your base URL.
If you’ve done everything right, you should see a welcome page when you navigate to your base URL.
Installing REST Server Library
Phil Sturgeon developed an excellent REST server library for CodeIgniter, which we’ll use in our tutorial. Here’s how to install it:
- Download the code from the GitHub repo here (https://github.com/chriskacerguis/codeigniter-restserver).
- Extract the downloaded files.
- Move the extracted files to the application directory of your CodeIgniter installation.
Building a RESTful API
Let’s get down to business and build a simple API for managing a list of users. We’ll implement endpoints for creating, reading, updating, and deleting users.
Creating the User Model
First, we’ll create a user model for handling database operations. Create a new file called User_model.php in the application/models directory and add the following code:
```php <?php class User_model extends CI_Model { public function __construct() { $this->load->database(); } public function get_users($id = NULL) { if ($id === NULL) { $query = $this->db->get('users'); return $query->result_array(); } $query = $this->db->get_where('users', array('id' => $id)); return $query->row_array(); } public function set_users($id = NULL) { $data = array( 'username' => $this->input->post('username'), 'email' => $this->input->post('email') ); if ($id == NULL) { return $this->db->insert('users', $data); } else { $this->db->where('id', $id); return $this->db->update('users', $data); } } public function delete_users($id) { $this->db->where('id', $id); return $this->db->delete('users'); } } ?> ```
Creating the User Controller
Now, let’s create a user controller in application/controllers/api directory. We’ll call it User.php and add the following code:
```php <?php require APPPATH . '/libraries/REST_Controller.php'; class User extends REST_Controller { public function __construct() { parent::__construct(); $this->load->model('user_model'); } public function index_get() { $id = $this->get('id'); if ($id === NULL) { $users = $this->user_model->get_users(); if ($users) { $this->response($users, REST_Controller::HTTP_OK); } else { $this->response([ 'status' => FALSE, 'message' => 'No users were found' ], REST_Controller::HTTP_NOT_FOUND); } } else { $user = $this->user_model->get_users($id); if ($user) { $this->response($user, REST_Controller::HTTP_OK); } else { $this->response([ 'status' => FALSE, 'message' => 'No user was found' ], REST_Controller::HTTP_NOT_FOUND); } } } public function index_post() { $result = $this->user_model->set_users(); if ($result) { $this->response([ 'status' => TRUE, 'message' => 'User created successfully' ], REST_Controller::HTTP_OK); } else { $this->response([ 'status' => FALSE, 'message' => 'User could not be created' ], REST_Controller::HTTP_BAD_REQUEST); } } public function index_put() { $id = $this->get('id'); $result = $this->user_model->set_users($id); if ($result) { $this->response([ 'status' => TRUE, 'message' => 'User updated successfully' ], REST_Controller::HTTP_OK); } else { $this->response([ 'status' => FALSE, 'message' => 'User could not be updated' ], REST_Controller::HTTP_BAD_REQUEST); } } public function index_delete() { $id = $this->get('id'); if ($id === NULL) { $this->response([ 'status' => FALSE, 'message' => 'No ID was provided' ], REST_Controller::HTTP_BAD_REQUEST); } $result = $this->user_model->delete_users($id); if ($result) { $this->response([ 'status' => TRUE, 'message' => 'User deleted successfully' ], REST_Controller::HTTP_OK); } else { $this->response([ 'status' => FALSE, 'message' => 'User could not be deleted' ], REST_Controller::HTTP_BAD_REQUEST); } } } ?> ```
Congratulations! You have built a RESTful API using CodeIgniter. Now, you can test these APIs using Postman or any other tool of your choice. Remember to replace the URLs with your own server.
Conclusion
CodeIgniter’s lightweight and straightforward design makes it an excellent choice for developing RESTful APIs. Its speed and simplicity, combined with the power of the REST server library, allow for efficient creation and manipulation of web services. By using the approach laid out in this article, you can quickly and reliably develop complex applications.
Keep in mind that real-world applications often require more sophisticated functionalities such as authentication, error handling, rate limiting, etc. Be sure to study and implement these aspects to create secure and robust web services.
Remember, continuous learning and practice is the key to mastering any framework. Happy coding!
Table of Contents
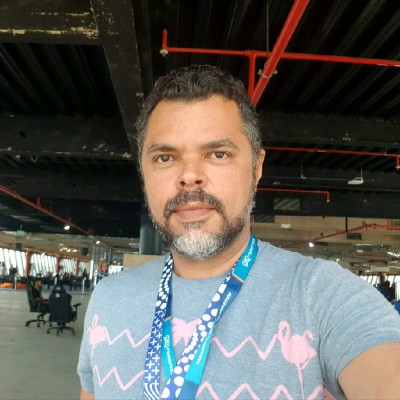
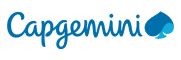