Elevate Your CodeIgniter Apps with Advanced SSO Integration
CodeIgniter is a renowned PHP framework known for its simplicity and lightweight approach to web application development. While it provides robust capabilities for handling user authentication, modern applications often require more sophisticated methods to handle user sessions across multiple platforms and services. This is where Single Sign-On (SSO) comes in handy.
In this blog post, we will delve into the benefits of integrating Single Sign-On with CodeIgniter and provide examples of how to implement this efficiently.
1. What is Single Sign-On (SSO)?
Single Sign-On (SSO) is an authentication process that allows users to access multiple services and applications using a single set of credentials (like a username and password). This means that once a user is authenticated with one application, they can seamlessly navigate to another connected application without having to log in again.
2. Benefits of SSO with CodeIgniter
- Improved User Experience: Users don’t have to remember multiple passwords for different services, leading to fewer login frustrations.
- Enhanced Security: Centralized authentication can have stringent security protocols, reducing the vulnerability points.
- Simplified Management: Fewer passwords and centralized authentication means easier user management and maintenance.
- Reduced Password Fatigue: Less need for users to remember and manage multiple credentials.
3. Implementation of SSO with CodeIgniter
3.1. Setting up a Centralized Authentication Server
Before you can implement SSO in your CodeIgniter application, you need a centralized authentication server. This server will be responsible for authenticating users and providing tokens for authenticated sessions.
For this example, we’ll use an OAuth2 server. (There are various OAuth2 server implementations available; choose one that suits your needs.)
3.2. Modify CodeIgniter for SSO
Once the OAuth2 server is up, we have to modify our CodeIgniter application to redirect users for authentication to this server.
- Installing necessary libraries
Use Composer to install required OAuth2 client libraries:
```bash composer require league/oauth2-client ```
- Integrating OAuth2 client with CodeIgniter
In your controller, redirect the user to the OAuth2 server for authentication:
```php public function login() { $provider = new \League\OAuth2\Client\Provider\GenericProvider([ 'clientId' => 'your-client-id', 'clientSecret' => 'your-client-secret', 'redirectUri' => 'http://your-ci-app/callback', 'urlAuthorize' => 'http://oauth-server/authorize', 'urlAccessToken' => 'http://oauth-server/token', 'urlResourceOwnerDetails' => 'http://oauth-server/resource' ]); // If we don't have an authorization code, get one if (!isset($_GET['code'])) { $authorizationUrl = $provider->getAuthorizationUrl(); $_SESSION['oauth2state'] = $provider->getState(); header('Location: ' . $authorizationUrl); exit; } else { // Check the state if (empty($_GET['state']) || ($_GET['state'] !== $_SESSION['oauth2state'])) { unset($_SESSION['oauth2state']); exit('Invalid state'); } // Get an access token $accessToken = $provider->getAccessToken('authorization_code', [ 'code' => $_GET['code'] ]); // Store the token in the session $_SESSION['access_token'] = $accessToken->getToken(); } } ```
- Handling User Data
Once the user is authenticated, the OAuth2 server will return user details. Integrate these details into your CodeIgniter’s authentication system. Depending on your setup, you might store this data in a session, cache, or database.
3. Handling Logouts
When a user logs out, you must ensure the session is terminated not only in your CodeIgniter app but also in the central authentication server:
```php public function logout() { // Destroy local session session_destroy(); // Redirect to OAuth2 server to destroy the session there as well header('Location: http://oauth-server/logout'); } ```
Conclusion
Single Sign-On (SSO) is a robust way to enhance user experience and security across multiple platforms and services. With CodeIgniter’s flexible framework, integrating SSO, particularly using the OAuth2 standard, is relatively straightforward. As web services grow and evolve, leveraging technologies like SSO will become increasingly vital to providing seamless and secure user experiences.
Table of Contents
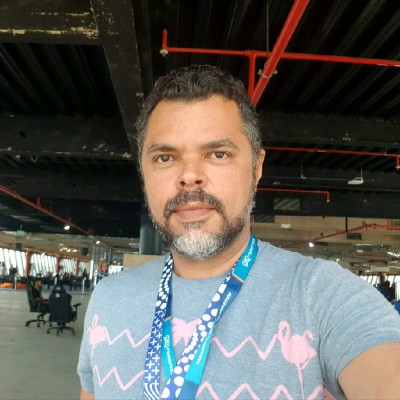
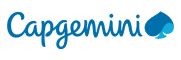