Ensure Flawless Code: Dive into Unit Testing with CodeIgniter & PHPUnit
Code quality is paramount to any successful software project. One of the most effective ways to ensure quality and reliability is through unit testing. For those developing with the popular PHP framework, CodeIgniter, integrating unit tests can be a powerful way to build more robust applications. If you’re aiming for top-tier results, you might consider seeking to hire CodeIgniter developers to fortify your team. In this blog post, we’ll explore the basics of unit testing in CodeIgniter and provide examples to help you get started.
Table of Contents
1. What is Unit Testing?
Unit testing is a software testing method in which individual units or components of a software are tested to determine if they work as expected. Each unit is tested in isolation from the rest of the code to ensure its correctness.
2. Benefits of Unit Testing:
- Error Detection: Identify bugs and issues early in the development cycle.
- Refactoring Confidence: Ensure that changes or additions to the code don’t introduce new bugs.
- Improved Design: Encourages writing cleaner, more modular code.
- Documentation: Tests act as documentation, showing how a piece of functionality is supposed to work.
- Faster Development: With a suite of tests, developers can make changes faster, knowing they haven’t broken existing functionality.
3. CodeIgniter and PHPUnit
While CodeIgniter does not come with built-in unit testing tools, it is compatible with PHPUnit, the de facto standard for unit testing in PHP. This provides a robust platform for writing and executing tests.
4. Setting Up PHPUnit with CodeIgniter:
Before you begin, ensure that you have CodeIgniter and Composer set up. To integrate PHPUnit:
- Install PHPUnit via Composer:
```bash composer require --dev phpunit/phpunit ```
- Create a `phpunit.xml` in your project root to configure your testing environment. Here’s a basic example:
```xml <?xml version="1.0" encoding="UTF-8"?> <phpunit bootstrap="vendor/autoload.php"> <testsuites> <testsuite name="CodeIgniter Unit Tests"> <directory>./application/tests</directory> </testsuite> </testsuites> </phpunit> ```
- Create a `tests` directory inside the `application` directory, where your test cases will reside.
5. Writing a Simple Test:
Assume you have a model named `User_model` in CodeIgniter with a method `getUsername($id)` that fetches a username based on the user’s ID.
- Create a new test file: `application/tests/UserModelTest.php`.
- Write the test
```php <?php use PHPUnit\Framework\TestCase; class UserModelTest extends TestCase { public function setUp(): void { // Load the CodeIgniter instance $this->CI = &get_instance(); $this->CI->load->model('User_model'); $this->obj = $this->CI->User_model; } public function testGetUsername() { // Assume there's a user with ID 1 and username 'john_doe' $username = $this->obj->getUsername(1); $this->assertEquals('john_doe', $username); } } ```
- Run the test using PHPUnit:
```bash ./vendor/bin/phpunit ```
If everything is set up correctly, PHPUnit will execute the test and you’ll see the results.
6. Mocking
Often, you’ll want to isolate the unit of code you’re testing from external dependencies, like databases or APIs. In such cases, mocking becomes invaluable.
Using the same `User_model`, assume you want to test the method `createUser($data)` without actually inserting a user into the database.
```php public function testCreateUser() { $mockedData = ['id' => 2, 'username' => 'jane_doe']; // Mock the User_model $mock = $this->getMockBuilder('User_model') ->setMethods(['insert']) ->getMock(); // Set up the expectation $mock->expects($this->once()) ->method('insert') ->with($this->equalTo($mockedData)) ->willReturn(true); // Use the mock instead of the real model $this->obj = $mock; $result = $this->obj->createUser($mockedData); $this->assertTrue($result); } ```
Final Thoughts
Unit testing with CodeIgniter, while not inherently integrated, can be easily achieved using PHPUnit. By embracing unit testing, you can elevate the quality of your CodeIgniter applications and ensure that they remain reliable and bug-free as they grow and evolve. If you’re looking to take your projects to the next level, you might consider seeking to hire CodeIgniter developers.
Remember, the key to effective unit testing is to test early, test often, and focus on isolating and testing each component of your software in a dedicated manner. Happy coding and testing!
Table of Contents
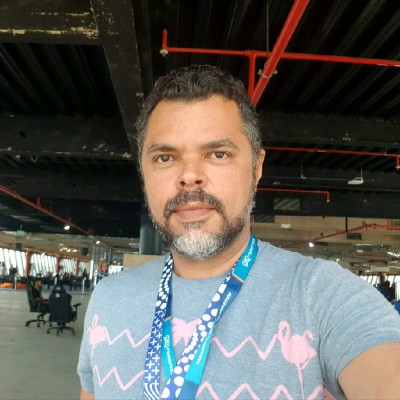
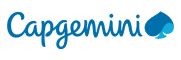