Get Ahead with CodeIgniter: User Registration and Login Simplified
In the realm of web development, one of the most common functionalities that you will need to implement is user registration and login. In the PHP ecosystem, CodeIgniter is a powerful and popular framework that simplifies this task with its robust and user-friendly functionalities. In this post, we’ll explore a simple yet practical method to implement user registration and login using CodeIgniter.
Pre-Requisites
Before we proceed, ensure that you have a fundamental understanding of PHP and a basic knowledge of CodeIgniter. Additionally, ensure that you have CodeIgniter installed on your local development environment and MySQL setup for database operations.
Setting Up the Database
Let’s start by setting up our user’s database table. Here is a basic structure:
```sql CREATE TABLE `users` ( `id` INT(11) NOT NULL AUTO_INCREMENT, `username` VARCHAR(255) NOT NULL, `email` VARCHAR(255) NOT NULL, `password` VARCHAR(255) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8; ```
Creating a User Model
Create a model named `User_model.php` under the `application/models` directory.
```php <?php class User_model extends CI_Model { public function __construct() { $this->load->database(); } public function register($username, $email, $password) { $data = array( 'username' => $username, 'email' => $email, 'password' => password_hash($password, PASSWORD_BCRYPT) ); return $this->db->insert('users', $data); } public function login($username, $password) { $query = $this->db->get_where('users', array('username' => $username)); if($query->num_rows() == 1) { $user = $query->row(); if(password_verify($password, $user->password)) { return $user->id; } else { return false; } } else { return false; } } } ?> ```
Creating the Controller
Now, we need to create a controller. This will handle the request and response. Create a file named `Auth.php` under the `application/controllers` directory.
```php <?php defined('BASEPATH') OR exit('No direct script access allowed'); class Auth extends CI_Controller { public function __construct() { parent::__construct(); $this->load->model('User_model'); } public function register() { $this->load->helper(array('form', 'url')); $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required'); $this->form_validation->set_rules('email', 'Email', 'required|valid_email'); $this->form_validation->set_rules('password', 'Password', 'required|min_length[8]'); if ($this->form_validation->run() === FALSE) { $this->load->view('register'); } else { $this->User_model->register( $this->input->post('username'), $this->input->post('email'), $this->input->post('password') ); redirect('login'); } } public function login() { $this->load->helper(array('form', 'url')); $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required'); $this->form_validation->set_rules('password', 'Password', 'required'); if ($this->form_validation->run() === FALSE) { $this->load->view('login'); } else { $login_id = $this->User_model->login( $this->input->post('username'), $this->input->post('password') ); if($login_id) { $this->session->set_userdata('user_id', $login_id); redirect('dashboard'); } else { $this->session->set_flashdata('error', 'Invalid username or password'); redirect('login'); } } } public function logout() { $this->session->unset_userdata('user_id'); redirect('login'); } } ?> ```
Creating Views
Views in CodeIgniter are the HTML files that the user sees. In our case, we’ll have three views: `register.php`, `login.php`, and `dashboard.php`. These views should be placed in the `application/views` directory.
1. Register View
```html <!DOCTYPE html> <html> <head> <title>Registration</title> </head> <body> <h2>Register</h2> <?php echo validation_errors(); ?> <?php echo form_open('auth/register'); ?> <input type="text" name="username" placeholder="Username" required> <input type="email" name="email" placeholder="Email" required> <input type="password" name="password" placeholder="Password" required> <button type="submit">Register</button> </form> </body> </html> ```
2. Login View
```html <!DOCTYPE html> <html> <head> <title>Login</title> </head> <body> <h2>Login</h2> <?php if(isset($_SESSION['error'])) { echo $_SESSION['error']; } ?> <?php echo validation_errors(); ?> <?php echo form_open('auth/login'); ?> <input type="text" name="username" placeholder="Username" required> <input type="password" name="password" placeholder="Password" required> <button type="submit">Login</button> </form> </body> </html> ```
3. Dashboard View
```html <!DOCTYPE html> <html> <head> <title>Dashboard</title> </head> <body> <h2>Dashboard</h2> <p>Welcome, you are logged in!</p> <a href="<?php echo base_url('auth/logout'); ?>">Logout</a> </body> </html> ```
Conclusion
Now, we have a simple authentication system in place using CodeIgniter. The registration page allows users to create an account, and the login page verifies the credentials and allows access to the dashboard. The logout function clears the session and redirects the user back to the login page.
Always remember, this tutorial demonstrates a basic authentication system. For a production-level application, consider incorporating more advanced features like email verification, password resets, and most importantly, implementing better security practices to protect sensitive user information.
CodeIgniter’s clear and concise structure makes it a great tool for developing robust and secure applications. Keep exploring its extensive features and happy coding!
Table of Contents
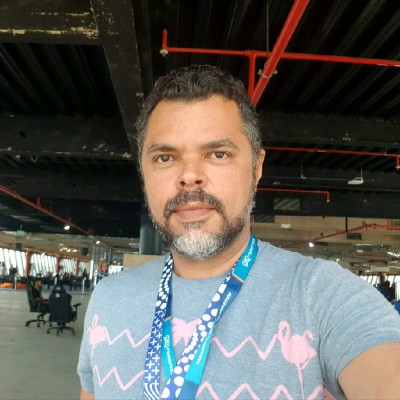
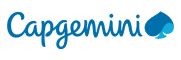