CodeIgniter and Web Security: Protecting Your App from Common Threats
Web security is a critical concern for developers, especially when building applications with frameworks like CodeIgniter. Understanding common security threats and implementing robust defenses can protect your app and its users. In this blog, we will explore key security threats and best practices for securing your CodeIgniter application.
Understanding Common Web Security Threats
SQL Injection
SQL Injection is a vulnerability that allows attackers to manipulate SQL queries by injecting malicious SQL code. This can lead to unauthorized access to the database, data leaks, or even deletion of data.
Example:
```php // Vulnerable code $username = $_POST['username']; $query = "SELECT * FROM users WHERE username = '$username'"; $result = $this->db->query($query); ```
Prevention:
Always use parameterized queries or CodeIgniter’s Query Builder to prevent SQL injection.
```php // Secure code $username = $this->input->post('username'); $query = $this->db->get_where('users', ['username' => $username]); ```
Cross-Site Scripting (XSS)
XSS attacks occur when malicious scripts are injected into web pages viewed by other users. This can lead to session hijacking, defacement, or redirecting users to malicious websites.
Example:
```php // Vulnerable code echo "<h1>Welcome, " . $_GET['name'] . "!</h1>"; ```
Prevention:
Use CodeIgniter’s html_escape function to escape user input.
```php // Secure code echo "<h1>Welcome, " . html_escape($this->input->get('name')) . "!</h1>"; ```
Cross-Site Request Forgery (CSRF)
CSRF is an attack that tricks a user into performing actions on a web application without their consent. This is possible when an attacker sends a user to a URL that executes actions using the user’s credentials.
Prevention:
CodeIgniter provides built-in CSRF protection that can be enabled in the config.php file.
```php $config['csrf_protection'] = TRUE; $config['csrf_token_name'] = 'csrf_test_name'; $config['csrf_cookie_name'] = 'csrf_cookie_name'; ```
When CSRF protection is enabled, CodeIgniter automatically generates and validates tokens for form submissions.
Best Practices for Securing CodeIgniter Applications
Use HTTPS
Ensure your application uses HTTPS to encrypt data transmitted between the client and server. This prevents data from being intercepted by attackers.
Secure Session Management
Proper session management is crucial to prevent session hijacking. CodeIgniter offers several session configuration options:
- Regenerate Session ID: Use the sess_regenerate_destroy setting to regenerate session IDs periodically.
- Secure Cookies: Set the cookie_secure option to TRUE to ensure cookies are only sent over HTTPS.
Validate and Sanitize Input
Always validate and sanitize user input to prevent various types of attacks. Use CodeIgniter’s form validation library to enforce input validation rules.
Example:
```php $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required|alpha_numeric'); ```
Use Latest CodeIgniter Version
Regularly update your CodeIgniter framework to the latest version. Security vulnerabilities are often patched in newer releases, so keeping your framework up-to-date is essential.
Further Reading
Table of Contents
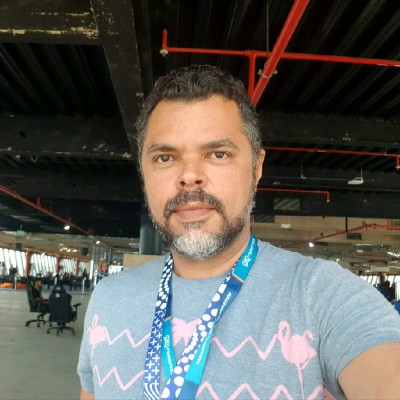
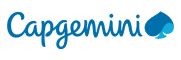