How to add custom middleware for authentication in Django REST framework?
Adding custom middleware for authentication in Django REST framework (DRF) can be useful when you need to implement authentication logic that goes beyond the built-in authentication classes provided by DRF. Custom middleware allows you to perform additional checks or custom processing before or after the DRF authentication classes are executed. Here’s how to add custom middleware for authentication in DRF:
- Create Your Custom Middleware:
Start by creating a custom middleware class. This class should define a `__init__` method for initialization and a `__call__` method for processing requests. Here’s a basic example:
```python class CustomAuthenticationMiddleware: def __init__(self, get_response): self.get_response = get_response def __call__(self, request): # Your custom authentication logic goes here # You can access request headers, session, or other data # and set request.user and request.auth accordingly # Example: # if some_condition: # request.user = CustomUser() # request.auth = "custom_token" response = self.get_response(request) return response ```
- Configure Middleware in Settings:
In your Django project’s settings, add your custom middleware to the `MIDDLEWARE` setting. Place it before the DRF’s `AuthenticationMiddleware` so that it runs before DRF’s authentication classes:
```python MIDDLEWARE = [ # ... 'path.to.CustomAuthenticationMiddleware', # Add your custom middleware here 'rest_framework.authentication.AuthenticationMiddleware', # ... ] ```
- Implement Authentication Logic:
Within your custom middleware’s `__call__` method, implement the authentication logic you need. You can access request headers, session data, or any other relevant information to determine the authentication status. Set `request.user` to the authenticated user (if any) and `request.auth` to the authentication token (if applicable).
- Error Handling:
Handle authentication errors gracefully by raising exceptions or returning appropriate responses when authentication fails. You can raise `AuthenticationFailed` exceptions or customize responses based on your application’s requirements.
By following these steps, you can add custom middleware for authentication in DRF. This middleware can perform additional authentication checks or apply custom authentication methods before passing the request to DRF’s built-in authentication classes. Custom middleware offers flexibility and allows you to tailor the authentication process to your specific project’s needs.
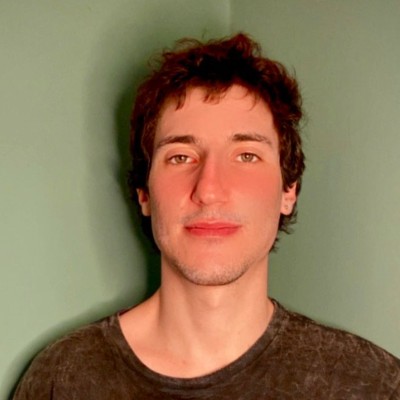
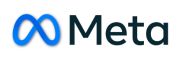