How to add real-time chat functionality to a application in Django?
Adding real-time chat functionality to a Django application can greatly enhance its interactivity and engagement. To achieve this, you can use Django Channels, which is an extension that enables handling real-time features like WebSocket communication. Here’s a step-by-step guide on how to add real-time chat to your Django application:
- Install Django Channels:
First, you need to install Django Channels. You can do this using pip:
``` pip install channels ```
- Configure Django Channels:
In your Django project’s settings, add `’channels’` to your `INSTALLED_APPS` and configure Django to use Channels as the default backend for ASGI (Asynchronous Server Gateway Interface). Update your `ASGI_APPLICATION` setting to point to your project’s routing configuration.
- Create a Chat App:
Create a new Django app specifically for handling chat functionality. You can name it something like `’chat’`. Inside this app, you’ll define models, serializers, and views for chat messages and rooms.
- Define Chat Models:
Define models for messages and chat rooms. For example, you can have a `Message` model with fields like `sender`, `content`, and `timestamp`, and a `Room` model to represent chat rooms.
- Create API Views:
Create API views using Django REST framework to send and receive chat messages. Implement endpoints for sending messages, retrieving chat history, and joining or creating chat rooms.
- Implement WebSocket Consumers:
Use Django Channels to implement WebSocket consumers for handling real-time chat. You’ll need to create consumers that handle WebSocket connections, disconnections, and message broadcasting to participants in a chat room.
- Frontend Integration:
Develop the frontend of your chat application using a JavaScript framework like React or Vue.js. Utilize WebSocket libraries such as `django-channels`, `channels-redux`, or `socket.io` to establish WebSocket connections from the frontend to your Django backend.
- Authentication and Authorization:
Implement user authentication and authorization to ensure that only authorized users can access and participate in chat rooms.
- Testing and Debugging:
Thoroughly test your chat functionality, especially WebSocket communication, using tools like Django’s testing framework and WebSocket debugging tools.
- Deployment:
Deploy your Django application, ensuring that WebSocket support is available on your production server. You might need to use Channels’ built-in support for channels layers, which can be backed by Redis or other message brokers.
- Scaling and Optimization:
Depending on your application’s expected load, consider scaling and optimizing your chat functionality using load balancers, auto-scaling, and database optimizations.
By following these steps, you can successfully integrate real-time chat functionality into your Django application, creating a dynamic and engaging user experience.
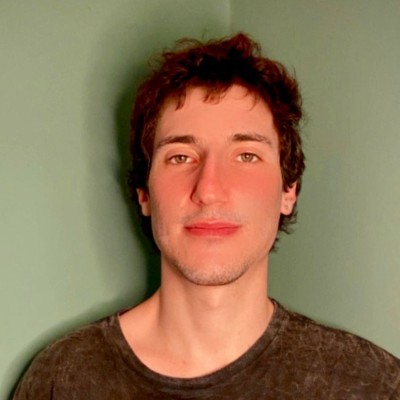
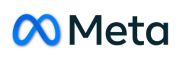